python批量将字符串转化为数字
时间: 2023-04-03 07:03:46 浏览: 178
可以使用Python内置的map函数和int函数来实现批量将字符串转化为数字,示例代码如下:
```
str_list = ['1', '2', '3', '4', '5']
num_list = list(map(int, str_list))
print(num_list)
```
输出结果为:[1, 2, 3, 4, 5]
相关问题
python dataFrame批量将日期字符串转为datetime
在Python中,如果你有一个DataFrame(Pandas库的数据结构)并且其中包含日期字符串,你可以使用`pandas`库中的`to_datetime`函数来批量转换这些日期字符串为`datetime`对象。这里有两个不同的方法:
1. 使用`strptime`函数[^1]:
```python
import pandas as pd
from datetime import datetime
# 假设df是一个DataFrame,startDate列是你想要转换的日期字符串
startDateCol = df['startDate']
dateformat = "%Y-%m-%d" # 根据实际日期格式设置此变量
df['convertedDate'] = startDateCol.apply(lambda x: datetime.strptime(x, dateformat).date())
```
这会将`startDateCol`列中的每个日期字符串转换为`date`对象,并添加一个新的列`convertedDate`到DataFrame。
2. 创建一个新的日期并格式化为字符串:
```python
df['startDate'] = df['startDate'].apply(lambda x: (datetime.strptime(x, dateformat).date()).strftime(dateformat))
```
这个方法是创建一个`datetime`对象,然后立即转换回原始格式的字符串。
python字符串 拼接
### Python 字符串拼接方法及实例
#### 使用加号 `+` 进行字符串连接
最简单的字符串拼接方式是使用加号 `+` 将两个或多个字符串相连。
```python
str1 = "Hello"
str2 = "World"
result = str1 + ", " + str2 + "!"
print(result) # 输出: Hello, World!
```
这种方法适用于少量字符串的简单拼接[^4]。
#### 使用 `join()` 方法进行批量拼接
对于大量字符串或者列表中的字符串,可以使用 `join()` 方法来高效地完成拼接工作。此方法接受一个可迭代对象作为参数,并将其中的所有元素按照指定分隔符连接成一个新的字符串。
```python
words = ["hello", "beautiful", "world"]
separator = "-"
joined_string = separator.join(words)
print(joined_string) # 输出: hello-beautiful-world
```
当需要在每两个单词之间加入特定字符时,这种方式非常方便[^3]。
#### 利用 f-string 实现表达式内嵌
自 Python 3.6 起引入了格式化字符串字面量(f-string),它允许直接在字符串前加上字母"f" 或者 "F" ,并在大括号 `{}` 中编写变量名或其他表达式来进行即时求值并插入到最终输出中。
```python
name = "Alice"
age = 30
greeting = f"My name is {name}, and I am {age} years old."
print(greeting) # 输出: My name is Alice, and I am 30 years old.
```
这种语法简洁明了,在处理动态数据时尤为有用[^1]。
#### 处理不同类型的数据混合拼接
如果尝试直接通过 `+` 符号把整数和其他类型的数值同字符串相加,则会引发错误;此时可以通过转换函数如 `str()`, 或采用上述提到的各种灵活多变的方式来实现安全有效的组合[^2].
阅读全文
相关推荐
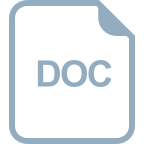
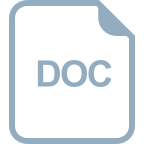
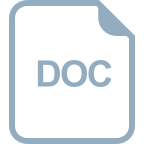
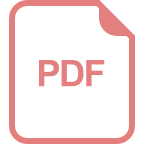
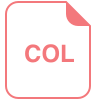
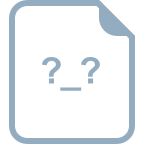
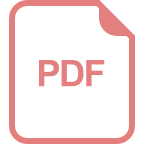
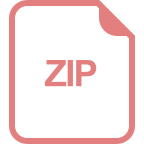
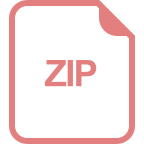
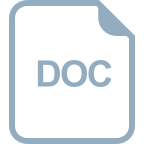
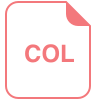
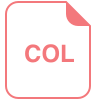




