用eclipse和mysql的数据库和Tomcat服务器做一个网页 请你帮我写出代码
时间: 2024-02-12 12:03:05 浏览: 158
好的,请您先按照以下步骤进行环境准备:
1. 安装 Eclipse IDE、MySQL 数据库和 Tomcat 服务器。
2. 在 Eclipse 中创建一个动态 Web 项目,并将其添加到 Tomcat 服务器中。
3. 在项目中引入 MySQL 的 JDBC 驱动程序。
接下来,我将为您提供一个简单的示例代码,用于连接 MySQL 数据库并查询数据并显示在网页上。
首先,创建一个 JSP 页面,例如 `index.jsp`,并在其中添加以下代码:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>MySQL 数据库查询示例</title>
</head>
<body>
<h1>MySQL 数据库查询示例</h1>
<table>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
</tr>
<%
try {
// 加载 MySQL JDBC 驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 连接 MySQL 数据库
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// 创建 SQL 语句
String sql = "SELECT * FROM users";
// 执行 SQL 语句
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql);
// 遍历查询结果并输出到网页上
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
%>
<tr>
<td><%=id %></td>
<td><%=name %></td>
<td><%=age %></td>
</tr>
<%
}
// 关闭数据库连接
rs.close();
stmt.close();
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
%>
</table>
</body>
</html>
```
在上述代码中,我们首先加载了 MySQL JDBC 驱动程序,然后连接到 MySQL 数据库并创建了一个 SQL 语句,然后使用 Statement 对象执行 SQL 语句并获取结果集,最后遍历结果集并将数据输出到网页上。
请注意,上述代码仅作为示例,实际开发中您需要根据自己的需求进行修改和完善,例如将数据库连接信息、SQL 语句等配置信息抽取到配置文件中,使用 PreparedStatement 对象替代 Statement 对象等。
阅读全文
相关推荐
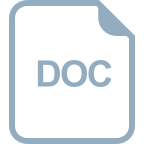
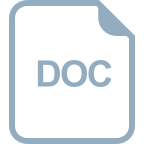
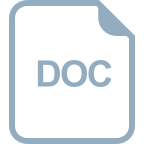



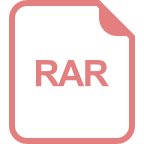
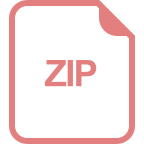
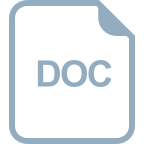
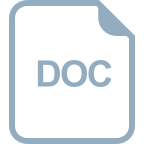
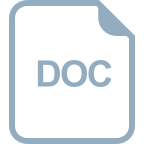
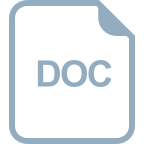
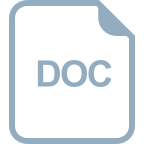
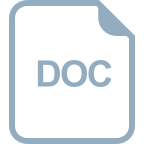
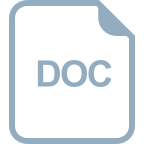
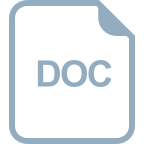


