mybatis-plus selectpage
时间: 2023-04-24 17:06:27 浏览: 202
Mybatis-Plus 的 selectPage 方法是用于分页查询数据的。它可以根据传入的参数进行分页查询,并返回查询结果和分页信息。在使用该方法时,需要先创建一个 Page 对象,然后将查询条件和分页信息设置到该对象中,最后调用 selectPage 方法进行查询。查询结果会被封装到 Page 对象中,可以通过该对象获取查询结果和分页信息。
相关问题
mybatis-plus selectPage
MyBatis-Plus is an enhanced version of MyBatis, a popular open-source Java persistence framework. It provides additional features and utilities to simplify database operations.
To perform a pagination query using MyBatis-Plus, you can use the `selectPage` method provided by the `com.baomidou.mybatisplus.core.mapper.BaseMapper` interface.
Here's an example of how to use the `selectPage` method:
```java
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.core.metadata.IPage;
// Assuming you have a mapper interface that extends BaseMapper
public interface YourMapper extends BaseMapper<YourEntity> {
}
// In your service or repository class
@Autowired
private YourMapper yourMapper;
public IPage<YourEntity> getEntityPage(int pageNo, int pageSize) {
// Create a Page object with the desired page number and page size
Page<YourEntity> page = new Page<>(pageNo, pageSize);
// Create a QueryWrapper object for your query conditions (if any)
QueryWrapper<YourEntity> queryWrapper = new QueryWrapper<>();
// Perform the pagination query using selectPage
return yourMapper.selectPage(page, queryWrapper);
}
```
In the above example, `YourEntity` represents your entity class, `YourMapper` is the mapper interface extending `BaseMapper`, and `yourMapper` is an instance of the mapper interface injected using the `@Autowired` annotation.
The `getEntityPage` method takes two arguments: `pageNo` for the desired page number and `pageSize` for the number of records per page. It creates a `Page` object with these parameters and a `QueryWrapper` object for any query conditions. Finally, it calls the `selectPage` method on the mapper interface to perform the pagination query and returns the resulting `IPage` object.
You can customize the `QueryWrapper` object to add various query conditions such as equals, greater than, less than, etc., depending on your requirements.
Please note that this is just a basic example, and there are many other features and options available in MyBatis-Plus for pagination and database operations. You can refer to the official documentation for more details: [MyBatis-Plus Documentation](https://mybatis.plus/)
mybatis-plus selectpage接口写什么
Mybatis-Plus提供了一个非常方便的`selectPage`接口,用于分页查询数据。该接口可以直接返回一个Page对象,其中包含了查询出来的数据列表和分页信息。
使用该接口需要先创建一个Page对象,然后调用`selectPage`方法进行查询。示例代码如下:
```java
// 创建分页对象,指定当前页和每页记录数
Page<User> page = new Page<>(1, 10);
// 构建查询条件
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("age", 20);
// 执行分页查询
IPage<User> userPage = userService.getBaseMapper().selectPage(page, queryWrapper);
// 获取分页信息和查询结果
long total = userPage.getTotal();
List<User> userList = userPage.getRecords();
```
在以上示例代码中,我们创建了一个Page对象,并指定了当前页和每页记录数。然后使用QueryWrapper构建了查询条件,最后调用`selectPage`方法进行分页查询。查询结果会被封装到一个IPage对象中,我们可以通过该对象获取分页信息和查询结果。
阅读全文
相关推荐



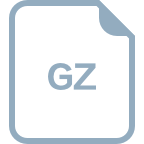











