用c语言写个俄罗斯方块
时间: 2023-05-11 10:03:41 浏览: 56
非常感谢您的提问,以下是用C语言编写俄罗斯方块的代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 10
#define HEIGHT 20
int board[HEIGHT][WIDTH] = {0};
int shapes[7][4][4] = {
{{0,0,0,0},
{0,1,1,0},
{0,1,1,0},
{0,0,0,0}},
{{0,0,0,0},
{0,0,2,0},
{0,2,2,2},
{0,0,0,0}},
{{0,0,0,0},
{0,3,3,0},
{0,0,3,3},
{0,0,0,0}},
{{0,0,0,0},
{0,4,0,0},
{0,4,4,4},
{0,0,0,0}},
{{0,0,0,0},
{0,0,5,0},
{0,0,5,0},
{0,5,5,0}},
{{0,0,0,0},
{0,6,0,0},
{0,6,6,0},
{0,0,6,0}},
{{0,0,0,0},
{0,0,7,0},
{0,0,7,0},
{0,0,7,7}}
};
int current_shape[4][4] = {0};
int current_x = 0;
int current_y = 0;
int current_type = 0;
int score = 0;
void draw_board();
void draw_shape(int x, int y, int type);
void erase_shape(int x, int y, int type);
void new_shape();
int check_collision(int x, int y, int type);
void rotate_shape();
void move_left();
void move_right();
void move_down();
void clear_lines();
int main()
{
srand(time(NULL));
new_shape();
while (1) {
draw_board();
draw_shape(current_x, current_y, current_type);
Sleep(1000);
erase_shape(current_x, current_y, current_type);
move_down();
if (check_collision(current_x, current_y+1, current_type)) {
draw_shape(current_x, current_y, current_type);
clear_lines();
new_shape();
}
}
return 0;
}
void draw_board()
{
system("cls");
printf("Score: %d\n", score);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
printf(".");
} else {
printf("*");
}
}
printf("\n");
}
}
void draw_shape(int x, int y, int type)
{
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[type][i][j] != 0) {
board[y+i][x+j] = shapes[type][i][j];
}
}
}
}
void erase_shape(int x, int y, int type)
{
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[type][i][j] != 0) {
board[y+i][x+j] = 0;
}
}
}
}
void new_shape()
{
current_x = WIDTH/2 - 2;
current_y = 0;
current_type = rand() % 7;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
current_shape[i][j] = shapes[current_type][i][j];
}
}
}
int check_collision(int x, int y, int type)
{
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[type][i][j] != 0) {
if (y+i >= HEIGHT || x+j < 0 || x+j >= WIDTH || board[y+i][x+j] != 0) {
return 1;
}
}
}
}
return 0;
}
void rotate_shape()
{
int temp[4][4] = {0};
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
temp[i][j] = current_shape[i][j];
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
current_shape[i][j] = temp[3-j][i];
}
}
if (check_collision(current_x, current_y, current_type)) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
current_shape[i][j] = temp[i][j];
}
}
}
}
void move_left()
{
if (!check_collision(current_x-1, current_y, current_type)) {
current_x--;
}
}
void move_right()
{
if (!check_collision(current_x+1, current_y, current_type)) {
current_x++;
}
}
void move_down()
{
if (!check_collision(current_x, current_y+1, current_type)) {
current_y++;
}
}
void clear_lines()
{
int lines_cleared = 0;
for (int i = HEIGHT-1; i >= 0; i--) {
int line_full = 1;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
line_full = 0;
break;
}
}
if (line_full) {
lines_cleared++;
for (int j = 0; j < WIDTH; j++) {
for (int k = i; k > 0; k--) {
board[k][j] = board[k-1][j];
}
board[0][j] = 0;
}
i++;
}
}
score += lines_cleared * lines_cleared * 100;
}
相关推荐
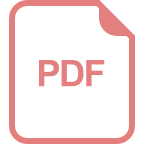
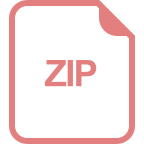


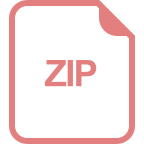
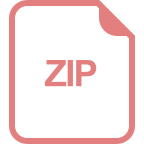
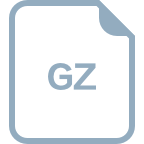
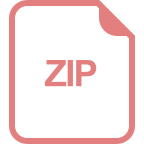
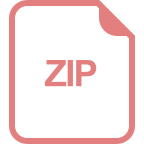