#ifndef_point_h #define_point_h class point { public: void initpoint(float x = 0, float y = 0) { this->x = x; this->y = y; } void move(float offx, float offy) { x += offx; y += offy; } float getx()const { return x; } float gety()const { return y; } private: float x, y; }; #endif
时间: 2024-04-25 11:27:23 浏览: 47
这段代码定义了一个名为point的类,它表示二维平面中的一个点。该类包含了以下成员函数:
- initpoint(float x = 0, float y = 0):该函数用于初始化该点的坐标,可以通过传入参数x和y来指定坐标值,否则默认为0。
- move(float offx, float offy):该函数用于移动该点的位置,并且传入参数offx和offy分别表示该点在x和y方向上的偏移量。
- getx()const:该函数用于获取该点的x坐标,它是一个常成员函数,表示该函数不会修改该类的成员变量。
- gety()const:该函数用于获取该点的y坐标,它也是一个常成员函数。
该类只有两个私有成员变量x和y,它们分别表示该点的x和y坐标。该类的定义使用了头文件保护,避免了重复包含该头文件。
相关问题
precision_recall.h文件代码
以下是 pcl/evaluation/precision_recall.h 文件中的代码示例,包括 PrecisionRecallCurve 类和 confusionMatrix 函数的定义:
```c++
#ifndef PCL_EVALUATION_PRECISION_RECALL_H_
#define PCL_EVALUATION_PRECISION_RECALL_H_
#include <pcl/common/common.h>
#include <pcl/point_types.h>
#include <vector>
#include <map>
#include <iostream>
#include <string>
#include <utility>
namespace pcl
{
namespace evaluation
{
/** \brief A class that computes precision-recall curves.
* \author Radu Bogdan Rusu
* \ingroup evaluation
*/
template<typename PointT>
class PrecisionRecallCurve
{
public:
typedef typename pcl::PointCloud<PointT>::Ptr PointCloudPtr;
typedef typename pcl::PointCloud<PointT>::ConstPtr PointCloudConstPtr;
/** \brief Constructor. */
PrecisionRecallCurve () : tp_ (0), fp_ (0), tn_ (0), fn_ (0) {};
/** \brief Destructor. */
virtual ~PrecisionRecallCurve () {};
/** \brief Add ground truth data.
* \param[in] ground_truth the input ground truth data
*/
virtual void
addGroundTruth (const PointCloudPtr &ground_truth);
/** \brief Add computed data.
* \param[in] computed_data the input computed data
*/
virtual void
addComputedData (const PointCloudPtr &computed_data);
/** \brief Compute the precision-recall curve for the given data.
* \param[out] precision_recall_curve the output precision-recall curve
*/
virtual void
compute (std::vector<std::pair<float, float> > &precision_recall_curve);
/** \brief Reset the internal counters. */
virtual void
reset ();
protected:
/** \brief The number of true positives. */
unsigned int tp_;
/** \brief The number of false positives. */
unsigned int fp_;
/** \brief The number of true negatives. */
unsigned int tn_;
/** \brief The number of false negatives. */
unsigned int fn_;
};
/** \brief Compute the confusion matrix.
* \param[in] ground_truth the input ground truth data
* \param[in] computed_data the input computed data
* \param[out] confusion the output confusion matrix
*/
void
confusionMatrix (const pcl::PointCloud<pcl::PointXYZRGBA>::ConstPtr &ground_truth,
const pcl::PointCloud<pcl::PointXYZRGBA>::ConstPtr &computed_data,
std::map<std::string, unsigned int> &confusion);
}
}
#include <pcl/evaluation/impl/precision_recall.hpp>
#endif // PCL_EVALUATION_PRECISION_RECALL_H_
```
其中,PrecisionRecallCurve 类提供了计算精度-召回率曲线的接口,addGroundTruth 和 addComputedData 函数用于添加实际标签和预测标签数据,compute 函数用于计算精度-召回率曲线,reset 函数用于重置内部计数器。confusionMatrix 函数用于计算混淆矩阵。
阅读全文
相关推荐
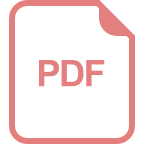
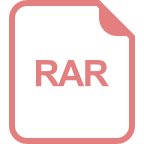
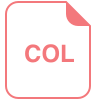
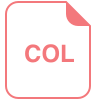
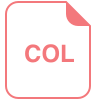
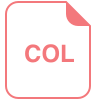
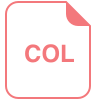
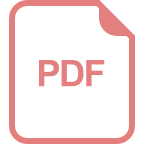