多重继承。定义一个描述日期(年、月、日)的类Date、一个描述时间(时、分、秒)的类Time,并由这两个类公有多重派生出日期时间类DateTime。主函数完成对日期时间类DateTime的测试,即通过一个DateTime类对象调用成员函数完成测试工作。要求如下: (1)定义普通函数 void itoa(int n, char *s) 将整数n转换成对应的十进制数字字符串并存放到s所指向的串,例如若n是168,则结果串s中存储的是"168"。此函数是公用函数,供以下两个类的成员函数调用。 (2)定义日期类 Date 私有数据成员: int year, month, day; 分别存放年、月、日的值,(3)定义时间类Time 私有数据成员: int hour, minute, second; 分别存放时、分、秒的值 公有成员函数: ①造函数:三个整型参数,完成时、分、秒的初始化 ②void setTime(int, int, int); 设置时、分、秒的值 ③void getTime(char *); 将时、分、秒转换成格式为“hh:mm:ss”(例如 "12:30:20")的字符串,存入参数所指向的字符串中。提示:调用函数itoa()实现将数值转换成字符串。 (4)日期时间类 DateTime:公有多重继承,继承日期类和时间类
时间: 2024-02-22 07:59:57 浏览: 20
好的,我明白了。这是一个多重继承的问题,需要定义一个日期类和一个时间类,并通过多重继承公有派生出日期时间类。最后需要完成对日期时间类的测试,即通过一个DateTime类对象调用成员函数完成测试工作。下面是代码实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void itoa(int n, char *s) {
if (n / 10) {
itoa(n / 10, s);
}
*s++ = n % 10 + '0';
*s = '\0';
}
class Date {
private:
int year, month, day;
public:
Date(int y=0, int m=0, int d=0): year(y), month(m), day(d) {}
void setDate(int y, int m, int d) {
year = y;
month = m;
day = d;
}
void getDate(char *s) {
char buf[5];
itoa(year, buf);
strcpy(s, buf);
strcat(s, "/");
itoa(month, buf);
if (month < 10) {
strcat(s, "0");
}
strcat(s, buf);
strcat(s, "/");
itoa(day, buf);
if (day < 10) {
strcat(s, "0");
}
strcat(s, buf);
}
};
class Time {
private:
int hour, minute, second;
public:
Time(int h=0, int m=0, int s=0): hour(h), minute(m), second(s) {}
void setTime(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
void getTime(char *s) {
char buf[3];
itoa(hour, buf);
if (hour < 10) {
strcat(s, "0");
}
strcat(s, buf);
strcat(s, ":");
itoa(minute, buf);
if (minute < 10) {
strcat(s, "0");
}
strcat(s, buf);
strcat(s, ":");
itoa(second, buf);
if (second < 10) {
strcat(s, "0");
}
strcat(s, buf);
}
};
class DateTime: public Date, public Time {
public:
DateTime(int y=0, int m=0, int d=0, int h=0, int mi=0, int s=0): Date(y, m, d), Time(h, mi, s) {}
};
int main() {
DateTime dt(2022, 9, 30, 12, 30, 20);
char s[20];
dt.getDate(s);
cout << s << endl;
dt.getTime(s);
cout << s << endl;
return 0;
}
```
这里定义了一个Date类和一个Time类,并通过多重继承公有派生出DateTime类。其中Date类用于描述日期,包括年、月、日三个数据成员,提供了设置和获取日期的函数;Time类用于描述时间,包括时、分、秒三个数据成员,提供了设置和获取时间的函数;DateTime类继承了Date类和Time类的所有成员,并提供了构造函数,用于初始化日期时间。
在主函数中,定义了一个DateTime类对象dt,并通过成员函数getDate和getTime获取日期和时间的字符串表示,最后输出结果。
相关推荐
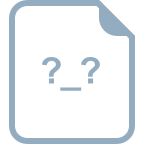
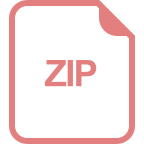
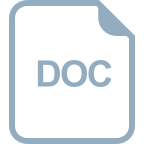















