python写一个打地鼠游戏代码
时间: 2023-07-22 10:41:16 浏览: 111
好的,下面是一个简单的 Python 实现打地鼠游戏的代码:
```python
import random
import time
class WhacAMole:
def __init__(self, num_holes):
self.num_holes = num_holes
self.holes = [0] * num_holes
self.score = 0
def print_board(self):
print("Score: {}".format(self.score))
for i in range(self.num_holes):
if self.holes[i] == 0:
print("|{}|".format(i+1), end="")
else:
print("|X|", end="")
print()
def add_mole(self):
index = random.randint(0, self.num_holes-1)
self.holes[index] = 1
def remove_mole(self, index):
self.holes[index] = 0
def play(self):
print("Welcome to Whac-A-Mole!")
print("You have 30 seconds to whack as many moles as you can.")
start_time = time.time()
while time.time() - start_time < 30:
self.print_board()
index = input("Choose a hole to whack (1-{}): ".format(self.num_holes))
index = int(index) - 1
if self.holes[index] == 1:
self.score += 1
self.remove_mole(index)
else:
print("Missed!")
self.add_mole()
time.sleep(1)
print("Time's up! Final score: {}".format(self.score))
# 测试
game = WhacAMole(6)
game.play()
```
这个程序实现一个有 6 个洞的打地鼠游戏。每隔一秒钟,程序会在随机的一个洞中出现一个地鼠。玩家需要在 30 秒内尽可能多地击中地鼠,每击中一个地鼠就会得到一分,未击中则不得分。最终输出总分数。
在这个代码实现中,我们使用了 `time` 模块来计时,并使用 `random` 模块随机生成地鼠出现的位置。玩家需要输入一个数字来选择击打的洞,如果该洞中有地鼠,则得分,否则不得分。你可以尝试修改这个代码来实现更多的功能,比如让游戏自动适应屏幕大小、增加更多的洞和地鼠等。
阅读全文
相关推荐










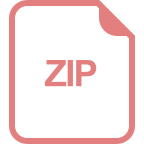
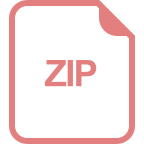
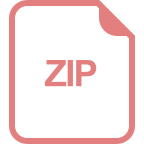