vue 判断字符串是否包含 <li class="line">
时间: 2023-02-07 13:57:51 浏览: 376
可以使用 JavaScript 中的 `String.prototype.includes()` 方法来判断字符串中是否包含某个子字符串。
```
let str = '<li class="line">';
if (string.includes(str)) {
// 字符串中包含 str
} else {
// 字符串中不包含 str
}
```
如果要在 Vue 组件中判断,可以将这段代码放在 Vue 组件的方法中,然后在组件模板中调用这个方法。
例如:
```
<template>
<div>
<button @click="checkString">检查字符串</button>
</div>
</template>
<script>
export default {
methods: {
checkString() {
let str = '<li class="line">';
if (this.string.includes(str)) {
console.log('字符串中包含 str');
} else {
console.log('字符串中不包含 str');
}
}
}
}
</script>
```
相关问题
<template> <ul class="list"> <li class="item" v-for="item of letters" :key="item" :ref="item" @touchstart.prevent="handleTouchStart" @touchmove="handleTouchMove" @touchend="handleTouchEnd" @click="handleLetterClick" > {{item}} </li> </ul> </template> <script> export default { name: 'CityAlphabet', props: { cities: Object }, computed: { letters () { const letters = [] for (let i in this.cities) { letters.push(i) } return letters } }, data () { return { touchStatus: false, startY: 0, timer: null } }, updated () { this.startY = this.$refs['A'][0].offsetTop }, methods: { handleLetterClick (e) { this.$emit('change', e.target.innerText) }, handleTouchStart () { this.touchStatus = true }, handleTouchMove (e) { if (this.touchStatus) { if (this.timer) { clearTimeout(this.timer) } this.timer = setTimeout(() => { const touchY = e.touches[0].clientY - 79 const index = Math.floor((touchY - this.startY) / 20) if (index >= 0 && index < this.letters.length) { this.$emit('change', this.letters[index]) } }, 8) } }, handleTouchEnd () { this.touchStatus = false } } } </script> <style lang="stylus" scoped> @import '~styles/varibles.styl' .list display: flex flex-direction: column justify-content: center position: absolute top: 1.58rem right: 0 bottom: 0 width: .4rem .item line-height: .4rem text-align: center color: $bgColor </style>解释一下这段代码
这段代码是一个 Vue 组件,用于渲染城市列表右侧的字母导航栏。具体解释如下:
- 模板部分:使用一个无序列表(ul)来展示字母导航栏,每个字母作为一个列表项(li)展示,并且使用 v-for 指令来循环遍历 letters 数组,同时使用 :key 和 :ref 指令来标识每个列表项。
- 脚本部分:定义了一个名为 CityAlphabet 的 Vue 组件,其中包含一个 props 属性 cities,用于接收城市列表数据。通过 computed 计算属性 letters 来获取 cities 对象中的所有属性名,即城市的首字母,并返回一个数组。
- 数据部分:使用 data 函数来定义组件的数据状态,包括 touchStatus(用于标识触摸状态)、startY(用于记录滑动开始的 Y 坐标)、timer(用于记录定时器)。
- 生命周期部分:在 updated 钩子函数中,当组件更新后,获取字母导航栏第一个字母所在的位置,即 $refs['A'][0].offsetTop,用于计算滑动时当前字母的索引值。
- 方法部分:定义了三个事件处理函数,分别是 handleLetterClick(处理点击字母的事件)、handleTouchStart(处理触摸开始的事件)、handleTouchMove(处理触摸滑动的事件)以及 handleTouchEnd(处理触摸结束的事件)。在 handleTouchMove 中,通过计算滑动距离和字母导航栏第一个字母所在位置,来获取当前所在的字母索引值,并触发 change 事件向父组件传递该字母信息。
- 样式部分:使用 stylus 预处理器编写样式,设置导航栏的位置、大小、颜色等属性。
<template> <div> <van-nav-bar title="重庆" left-arrow @click-left="$router.go(-1)" /> </div> </template> <script > </script> <style scoped> </style> 在此代码基础上写出可以执行的vue项目代码关于长沙火锅的专栏
以下是示例代码:
<template>
<div>
<van-nav-bar title="长沙火锅" left-arrow @click-left="$router.go(-1)" />
<div class="content">
<h3>长沙火锅简介</h3>
<p>长沙火锅是湖南特色美食之一,以牛肉、羊肉、鸭血、豆腐等为主要原料,其特点是麻辣鲜香,让人回味无穷。</p>
<h3>长沙火锅店推荐</h3>
<ul>
<li>老码头火锅:位于湘江风光带旁,环境宜人,口味地道。</li>
<li>红人火锅:精选优质食材,调味独特,吃过一次难以忘怀。</li>
<li>巴蜀麻辣烫:虽然名字中没有“火锅”二字,但其麻辣烫也是长沙人爱吃的美食之一。</li>
</ul>
</div>
</div>
</template>
<script>
export default {
name: 'ChangshaHotpot',
}
</script>
<style scoped>
.content {
padding: 20px;
font-size: 16px;
line-height: 1.5;
}
h3 {
margin: 20px 0 10px;
font-size: 20px;
}
</style>
阅读全文
相关推荐
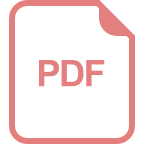
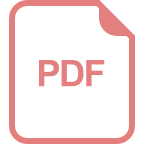
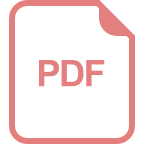
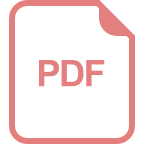
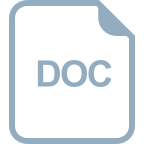
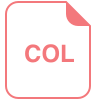
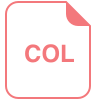









