def fenpei():#循环分配 driver.find_element_by_xpath("/html/body/div/div/div[2]/section/div/div[1]/div/div/ul/li[2]/span").click() sleep(1) print("点击待分配") test_danshu=driver.find_element_by_xpath("//*[@id='app']/div/div[2]/section/div/div[2]/div[2]/div/div[1]").text if test_danshu=="全部: 0单 禁:0单 预计批款总额: 0万": print("---------一单都没---------") driver.close() else: sleep(1) driver.find_element_by_xpath("//*[@id='app']/div/div[2]/section/div/div[2]/div[2]/div/div[2]/div/div[2]/div[8]/div/button[1]/span").click() print("点击派单") i = 0 while True: i += 1 print(i) test_paid = driver.find_element_by_xpath(f"//*[@id='app']/div/div[2]/section/div/div[2]/div[3]/table/tbody/tr[1]/td[{i}]/div/span").text print("12") if test_paid != "可预约": print("不能预约") if driver.find_element_by_xpath("//*[@id='app']/div/div[2]/section/div/div[2]/div[3]/table/tbody/tr[1]/td[15]/div/span"): print("第15个") element = driver.find_element_by_xpath("//*[@id='app']/div/div[2]/section/div/div[2]/div[3]") print("定位滚动条") sleep(1) driver.execute_script("arguments[0].scrollLeft = window.innerWidth", element) print("滑动") sleep(1) else: print("没有找到第15个") else: test_paid.click() print("122")为什么不执行print("12")
时间: 2024-04-27 09:19:37 浏览: 16
从代码中来看,如果 `test_paid` 不等于 "可预约",则会判断是否存在第 15 个元素,如果存在则滑动滚动条,否则打印 "没有找到第15个"。在这个过程中,如果 `test_paid` 不等于 "可预约",则不会执行 `test_paid.click()` 以及后面的代码,所以也不会执行 `print("12")`。可能的原因是:
- `test_paid` 的值一直为 "不能预约",导致没有执行 `test_paid.click()`。
- 在查找第 15 个元素时出现了异常,导致没有执行滑动滚动条的代码。
建议在代码中添加一些调试信息,例如打印 `test_paid` 的值,或者在滑动滚动条的代码前添加一个 `try-except` 语句来捕获异常,并打印异常信息,以便找到出错的原因。
相关问题
from selenium import webdriver import time # 导入selenium4 from selenium.webdriver.common.by import By # 创建一个浏览器实例 driver = webdriver.Chrome() # 打开网站 driver.get('http://192.168.31.114:4399/') time.sleep(3) # 输入账号 driver.find_element(By.XPATH, "/html/body/div[1]/div/div/div[1]/div[2]/div[1]/div/v-card-texts/form/div[1]/div[1]/div/div[3]/input").send_keys("13732237096") # 输入密码 driver.find_element(By.XPATH, "/html/body/div[1]/div/div/div[1]/div[2]/div[1]/div/v-card-texts/form/div[2]/div[1]/div/div[3]/input").send_keys( "zxcvbnm123") # 点击空白处等待登陆按钮生效 driver.find_element(By.CLASS_NAME, "v-messages").click() # 点击登陆 driver.find_element(By.XPATH, "/html/body/div[1]/div/div/div[1]/div[2]/div[1]/div/v-card-texts/form/button").click() time.sleep(2) # 关闭浏览器 driver.quit() print("注册成功")怎么对这段代码进行封装,提高可维护性
可以将这段代码封装成一个函数,函数名可以为`login_website`,函数的参数可以包括账号和密码,这样可以方便地调用函数来实现登录网站的功能。同时,可以使用`try except`语句来捕获异常,以便更好地处理错误。以下是示例代码:
```python
from selenium import webdriver
import time
from selenium.webdriver.common.by import By
def login_website(username, password):
try:
# 创建一个浏览器实例
driver = webdriver.Chrome()
# 打开网站
driver.get('http://192.168.31.114:4399/')
time.sleep(3)
# 输入账号
driver.find_element(By.XPATH, "/html/body/div[1]/div/div/div[1]/div[2]/div[1]/div/v-card-texts/form/div[1]/div[1]/div/div[3]/input").send_keys(username)
# 输入密码
driver.find_element(By.XPATH, "/html/body/div[1]/div/div/div[1]/div[2]/div[1]/div/v-card-texts/form/div[2]/div[1]/div/div[3]/input").send_keys(password)
# 点击空白处等待登陆按钮生效
driver.find_element(By.CLASS_NAME, "v-messages").click()
# 点击登陆
driver.find_element(By.XPATH, "/html/body/div[1]/div/div/div[1]/div[2]/div[1]/div/v-card-texts/form/button").click()
time.sleep(2)
# 关闭浏览器
driver.quit()
print("登录成功")
except Exception as e:
print("登录失败:", e)
# 调用函数
login_website("13732237096", "zxcvbnm123")
```
这样,当需要登录网站时,只需要调用`login_website`函数并传入正确的账号和密码即可。这种方式可以提高代码的可维护性,使代码更加简洁易读。
def test_case_01(self): """测试用例1""" self.driver.find_element(By.ID, "kw").send_keys("csdn") sleep(2) self.driver.find_element(By.ID, "su").click() sleep(5) self.driver.find_element(By.XPATH, "/html/body/div[3]/div[4]/div[1]/div[3]/div[1]/div/div[1]/div[2]/div/div[2]/div/a/span").click() sleep(8) all_h = self.driver.window_handles # 获取所有句柄 self.driver.switch_to.window(all_h[1]) # 切换到第二个窗口句柄 self.driver.find_element(By.ID, "toolbar-search-input").send_keys("软件测试") self.driver.find_element(By.ID, "toolbar-search-button").click() sleep(8) all_h = self.driver.window_handles self.driver.switch_to.window(all_h[2]) self.driver.find_element(By.XPATH, "/html/body/div[2]/div[2]/div[2]/div[1]/div[2]/div[2]/div[1]/div[1]/span[3]").click() sleep(2) self.driver.find_element(By.XPATH, "/html/body/div[2]/div[2]/div[2]/div[1]/div[2]/div[1]/div[2]").click() sleep(7) 帮我加一个断言判断是否登录成功
好的,可以在登录成功后,判断页面是否存在用户信息来判断是否登录成功。以下是例子代码:
```python
def test_case_01(self):
"""测试用例"""
self.driver.find(By.ID, "kw").send_keys("dn")
sleep(2)
self.driver.find_element(By.ID, "su").click()
sleep(5)
self.driver.find_element(By.XPATH, "/html/body/div[3]/div[4]/div[1]/div[3]/div[1]/div/div[1]/div[2]/div/div[2]/div/a/span").click()
sleep(8)
all_h = self.driver.window_handles # 获取所有句柄
self.driver.switch_to.window(all_h[1]) # 切换到第二个窗口句柄
self.driver.find_element(By.ID, "toolbar-search-input").send_keys("软件测试")
self.driver.find_element(By.ID, "toolbar-search-button").click()
sleep(8)
all_h = self.driver.window_handles
self.driver.switch_to.window(all_h[2])
self.driver.find_element(By.XPATH, "/html/body/div[2]/div[2]/div[2]/div[1]/div[2]/div[2]/div[1]/div[1]/span[3]").click()
sleep(2)
self.driver.find_element(By.XPATH, "/html/body/div[2]/div[2]/div[2]/div[1]/div[2]/div[1]/div[2]").click()
sleep(7)
# 判断是否登录成功
user_info = self.driver.find_element(By.XPATH, "//span[@class='header-nav__nickname']")
assert user_info.text == 'XXX' # 用户信息替换为实际值
```
在代码中,我使用了`find_element(By.XPATH, "//span[@class='header-nav__nickname']")`来查找用户信息元素,并使用`assert`语句断言用户信息是否符合预期。如果用户信息正确,则认为登录成功;否则登录失败。
相关推荐
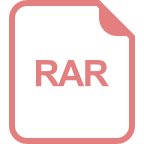
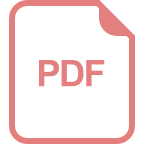






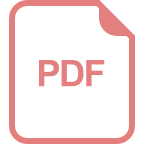
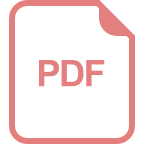
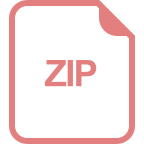
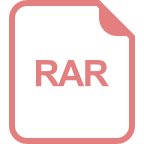