用python写一个模拟用户鼠标操作录制
时间: 2023-07-15 14:14:46 浏览: 56
可以使用 Python 的 PyAutoGUI 库来模拟用户鼠标操作录制。该库可以在 Windows、Mac 和 Linux 等操作系统上使用。
以下是一个示例代码,可以模拟用户在屏幕上单击、拖动和释放鼠标,然后将这些操作保存到文件中:
```python
import pyautogui
import time
# 记录鼠标操作的列表
actions = []
# 开始录制
print('Recording started...')
print('Press Ctrl-C to stop.')
time.sleep(2) # 等待 2 秒钟,以便打开需要操作的窗口
try:
while True:
x, y = pyautogui.position() # 获取鼠标的当前位置
action = {'x': x, 'y': y}
if pyautogui.mouseDown():
action['event'] = 'mousedown'
elif pyautogui.mouseUp():
action['event'] = 'mouseup'
elif pyautogui.drag():
action['event'] = 'drag'
actions.append(action)
time.sleep(0.1) # 等待 0.1 秒钟,以便捕获足够的动作
except KeyboardInterrupt:
# 用户按下了 Ctrl-C,停止录制并保存操作列表
print('Recording stopped.')
filename = input('Enter filename to save actions: ')
with open(filename, 'w') as f:
f.write(str(actions))
print(f'Actions saved to {filename}.')
```
该代码会在控制台输出一些提示信息,然后等待 2 秒钟以便打开需要操作的窗口。然后,它会进入一个无限循环,捕获鼠标的位置和动作,并将它们添加到 `actions` 列表中。当用户按下 Ctrl-C 时,程序会停止录制并要求用户输入一个文件名,然后将所有操作保存到该文件中。
可以使用以下代码来播放保存的操作:
```python
import ast
import pyautogui
import time
# 读取保存的操作列表
filename = input('Enter filename to play: ')
with open(filename, 'r') as f:
actions = ast.literal_eval(f.read())
# 开始播放操作
print('Playing actions...')
print('Press Ctrl-C to stop.')
time.sleep(2) # 等待 2 秒钟,以便打开需要操作的窗口
try:
for action in actions:
x, y = action['x'], action['y']
if 'event' not in action:
pyautogui.moveTo(x, y)
elif action['event'] == 'mousedown':
pyautogui.click(x, y, button='left')
elif action['event'] == 'mouseup':
pyautogui.mouseUp()
elif action['event'] == 'drag':
pyautogui.dragTo(x, y)
time.sleep(0.1) # 等待 0.1 秒钟,以便模拟真实操作的速度
except KeyboardInterrupt:
# 用户按下了 Ctrl-C,停止播放操作
print('Playback stopped.')
```
该代码会要求用户输入保存操作的文件名,然后读取该文件并播放其中保存的操作。与录制代码类似,该代码也会等待 2 秒钟以便打开需要操作的窗口。在播放过程中,它会模拟用户鼠标操作,并将它们发送到屏幕上。当用户按下 Ctrl-C 时,程序会停止播放。
相关推荐
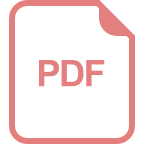














