用C语言编写一个函数,以一个单向链表的头指针做参数,函数返回值是这个链表的节点个数
时间: 2024-02-01 17:14:45 浏览: 77
以下是用C语言编写的函数,可以用于计算单向链表的节点个数:
```c
#include <stdio.h>
#include <stdlib.h>
//定义链表结构体
struct Node {
int data;
struct Node* next;
};
//定义计算链表节点个数的函数
int countNodes(struct Node* head) {
int count = 0; //计数器
struct Node* current = head; //指向当前节点的指针
//遍历链表
while (current != NULL) {
count++; //节点个数加1
current = current->next; //指向下一个节点
}
return count; //返回节点个数
}
int main() {
//创建链表
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
//调用计算链表节点个数的函数
int nodeCount = countNodes(head);
printf("链表节点个数为:%d\n", nodeCount);
return 0;
}
```
这个程序中,我们首先定义了一个链表结构体 `Node`,其中包含两个成员变量 `data` 和 `next`。`data` 表示节点中存储的数据,`next` 是一个指向下一个节点的指针。
接着,我们定义了一个计算链表节点个数的函数 `countNodes`,该函数的参数是链表的头指针 `head`。在函数中,我们定义了一个计数器 `count` 和一个指向当前节点的指针 `current`。我们使用 `while` 循环遍历链表,在每次循环中将计数器加1,同时将指针指向下一个节点。最后,函数返回计数器的值,即链表的节点个数。
在 `main` 函数中,我们创建了一个包含三个节点的链表,并调用了 `countNodes` 函数来计算节点个数。最终程序输出了链表节点的个数。
阅读全文
相关推荐
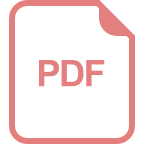
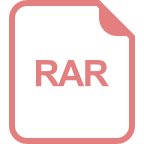
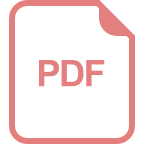
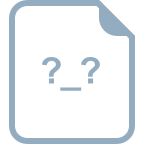
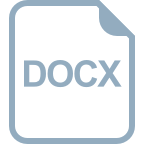
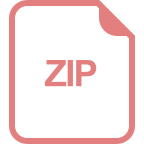
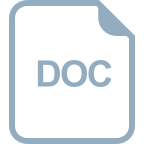
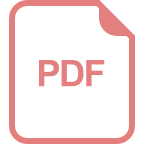
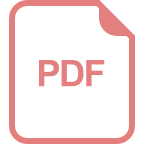
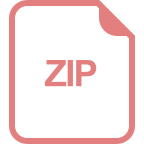
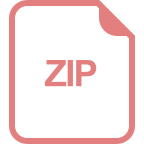