用C语言写一个生成数据的程序:⚫生成input.ifo文件(文件格式可以是文本文件,也可以是二进制文件),包含30个人名字(字符串:自己名字+随机编号,要求名字不同,随机编号用随机数生成,并保证不同)
时间: 2023-12-21 16:07:41 浏览: 31
以下是一个简单的用C语言生成input.ifo文件的程序,其中使用了rand()函数生成随机数来生成随机编号。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define NUM_NAMES 30
#define MAX_NAME_LEN 20
int main() {
// Open the output file for writing
FILE* outfile = fopen("input.ifo", "w");
if (!outfile) {
printf("Error: Could not open file for writing!\n");
return 1;
}
// Set up an array of first names
const char* first_names[] = {
"Alice",
"Bob",
"Charlie",
"David",
"Eve",
"Frank",
"Grace",
"Heidi",
"Ivan",
"John",
"Kate",
"Larry",
"Mallory",
"Nancy",
"Oscar",
"Peggy",
"Quincy",
"Randy",
"Steve",
"Tina",
"Ursula",
"Victor",
"Wendy",
"Xavier",
"Yvonne",
"Zoe"
};
const int num_first_names = sizeof(first_names) / sizeof(first_names[0]);
// Generate and write out the names
for (int i = 0; i < NUM_NAMES; i++) {
// Generate a random number for the last name
int last_name_num = rand() % 10000;
// Choose a random first name
const char* first_name = first_names[rand() % num_first_names];
// Build the full name
char full_name[MAX_NAME_LEN + 1];
snprintf(full_name, MAX_NAME_LEN + 1, "%s_%d", first_name, last_name_num);
// Write the name to the file
fprintf(outfile, "%s\n", full_name);
}
// Close the output file
fclose(outfile);
printf("Done!\n");
return 0;
}
```
相关推荐
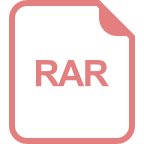















