翻译 <form id="addUserForm" th:action="@{/saveUser}" method="post">
时间: 2023-10-22 21:27:55 浏览: 56
这段代码片段是一个HTML表单,其中id为"addUserForm",action属性指定了表单提交的URL地址为"/saveUser",method属性指定了表单提交的HTTP方法为"post"。在使用Thymeleaf模板引擎时,th:action指定了表单提交的URL地址可以使用Thymeleaf的表达式语言来动态生成。
阅读全文
相关推荐
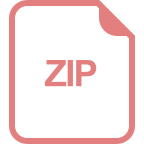
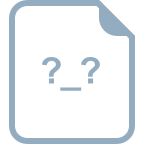
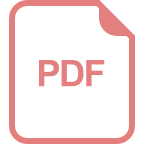
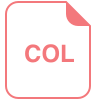
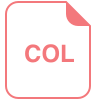
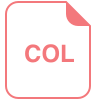
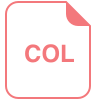
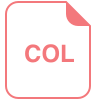
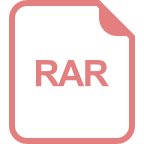
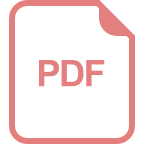
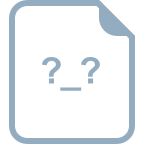
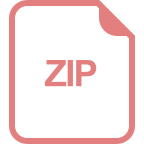
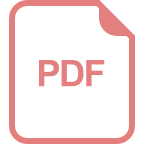
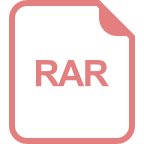
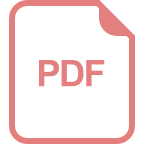
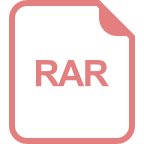
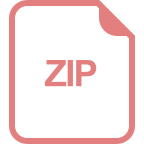
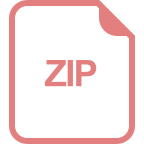