基于15单片机OLED显示屏代码
时间: 2023-11-09 11:07:39 浏览: 257
基于15单片机的OLED显示屏代码,可以使用一些OLED驱动芯片,如SSD1306、SH1106等。这里以SSD1306为例,提供一份基础的OLED显示屏代码:
```c
#include <reg51.h>
#include <intrins.h>
#define SCL P2_1
#define SDA P2_0
#define OLED_ADDR 0x78
void delay(int time) {
int i, j;
for (i = 0; i < time; i++) {
for (j = 0; j < 100; j++);
}
}
void iic_start() {
SDA = 1;
SCL = 1;
_nop_();
SDA = 0;
_nop_();
SCL = 0;
}
void iic_stop() {
SDA = 0;
SCL = 1;
_nop_();
SDA = 1;
_nop_();
SCL = 0;
}
void iic_write_byte(unsigned char dat) {
int i;
for (i = 0; i < 8; i++) {
SDA = dat & 0x80;
SCL = 1;
_nop_();
_nop_();
SCL = 0;
dat <<= 1;
}
}
void oled_init() {
iic_start();
iic_write_byte(OLED_ADDR);
iic_write_byte(0xAE);
iic_write_byte(0x00);
iic_write_byte(0x10);
iic_write_byte(0x40);
iic_write_byte(0x81);
iic_write_byte(0xCF);
iic_write_byte(0xA1);
iic_write_byte(0xC8);
iic_write_byte(0xA6);
iic_write_byte(0xA8);
iic_write_byte(0x3F);
iic_write_byte(0xD3);
iic_write_byte(0x00);
iic_write_byte(0xD5);
iic_write_byte(0x80);
iic_write_byte(0xD9);
iic_write_byte(0xF1);
iic_write_byte(0xDA);
iic_write_byte(0x12);
iic_write_byte(0xDB);
iic_write_byte(0x20);
iic_write_byte(0x8D);
iic_write_byte(0x14);
iic_write_byte(0xAF);
iic_stop();
}
void oled_set_pos(unsigned char x, unsigned char y) {
iic_start();
iic_write_byte(OLED_ADDR);
iic_write_byte(0xb0 + y);
iic_write_byte(((x & 0xf0) >> 4) | 0x10);
iic_write_byte((x & 0x0f) | 0x00);
iic_stop();
}
void oled_show_char(unsigned char x, unsigned char y, unsigned char chr) {
unsigned char c = 0, i = 0;
c = chr - ' ';
oled_set_pos(x, y);
for (i = 0; i < 8; i++) {
iic_write_byte(font8x16[c * 16 + i]);
}
oled_set_pos(x, y + 1);
for (i = 0; i < 8; i++) {
iic_write_byte(font8x16[c * 16 + i + 8]);
}
}
void oled_show_string(unsigned char x, unsigned char y, unsigned char *str) {
unsigned char j = 0;
while (str[j] != '\0') {
oled_show_char(x, y, str[j]);
x += 8;
if (x > 120) {
x = 0;
y += 2;
}
j++;
}
}
void oled_clear() {
unsigned char i, n;
for (i = 0; i < 8; i++) {
iic_start();
iic_write_byte(OLED_ADDR);
iic_write_byte(0xb0 + i);
iic_write_byte(0x00);
iic_write_byte(0x10);
for (n = 0; n < 128; n++) {
iic_write_byte(0x00);
}
iic_stop();
}
}
void main() {
oled_init();
oled_clear();
oled_show_string(0, 0, "Hello, world!");
while (1);
}
```
以上代码使用了基于IIC通信协议的OLED驱动方式,支持在OLED上显示字符串等信息。在使用时,需要将字库文件font8x16.h包含进来,其中定义了一些常用字符的字模信息。此外,还需要根据具体的OLED型号,修改一些初始化命令和通信地址等参数。
阅读全文
相关推荐
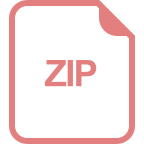
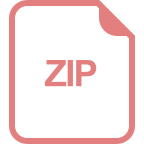
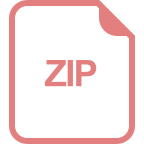
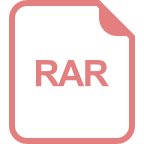
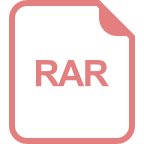
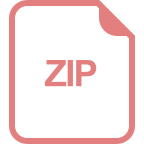
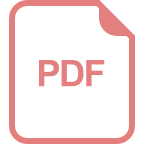
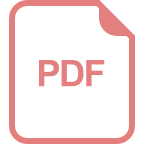
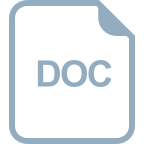
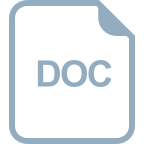
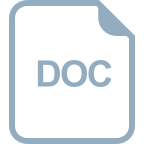
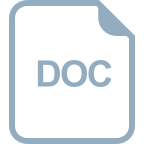
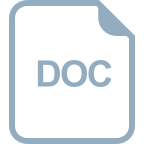
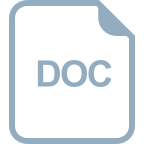


