springboot整合spring security oauth2 代码示例
时间: 2023-05-17 15:07:26 浏览: 197
以下是一个简单的 Spring Boot 整合 Spring Security OAuth2 的代码示例:
1. 添加依赖
在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.3.RELEASE</version>
</dependency>
```
2. 配置 OAuth2 客户端
在 application.yml 文件中添加以下配置:
```
security:
oauth2:
client:
clientId: your-client-id
clientSecret: your-client-secret
accessTokenUri: https://your-auth-server.com/oauth/token
userAuthorizationUri: https://your-auth-server.com/oauth/authorize
clientAuthenticationScheme: form
scope: read write
```
3. 配置 Spring Security
创建一个继承自 WebSecurityConfigurerAdapter 的类,并添加以下配置:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/oauth/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.csrf().disable();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER");
}
}
```
4. 创建资源服务器
创建一个继承自 ResourceServerConfigurerAdapter 的类,并添加以下配置:
```
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.anyRequest().permitAll();
}
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.resourceId("resource-id");
}
}
```
5. 创建控制器
创建一个简单的控制器,用于测试 OAuth2 认证:
```
@RestController
public class TestController {
@GetMapping("/api/test")
public String test() {
return "Hello, World!";
}
}
```
6. 运行应用程序
现在你可以运行应用程序,并访问 http://localhost:8080/api/test 进行测试。如果你没有提供正确的 OAuth2 认证信息,你将会收到一个 401 Unauthorized 错误。
阅读全文
相关推荐
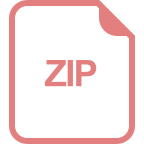
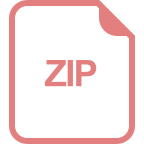
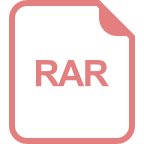
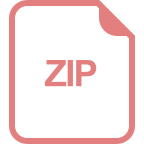
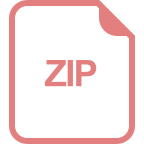
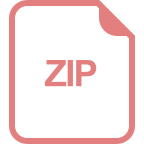
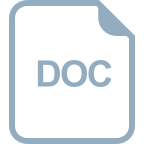
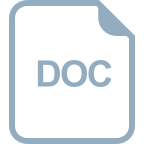
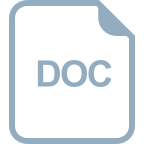



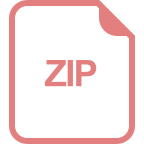
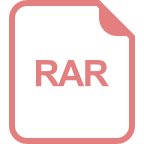
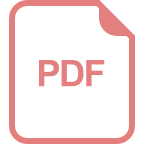
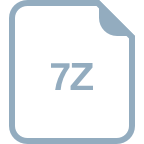
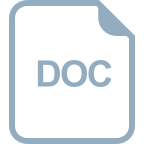