将下列python代码转化为c语言代码 import random def dist(x_mid, x_tang, y_mid, y_tang): return abs(x_mid - y_mid) + abs(x_tang - y_tang) data = [[0.697, 0.460],[0.774, 0.376],[0.634, 0.264], [0.608, 0.318],[0.556, 0.215],[0.403, 0.237], [0.481, 0.149],[0.437, 0.211],[0.666, 0.091], [0.243, 0.267],[0.245, 0.057],[0.343, 0.099], [0.639, 0.161],[0.657, 0.198],[0.360, 0.370], [0.593, 0.042],[0.719, 0.103],[0.359, 0.188], [0.339, 0.241],[0.282, 0.257],[0.748, 0.232], [0.714, 0.346],[0.483, 0.312],[0.478, 0.437], [0.525, 0.369],[0.751, 0.489],[0.532, 0.472], [0.473, 0.376],[0.725, 0.445],[0.446, 0.459]] sample_1 = data[0] sample_2 = data[1] while 1: ans_mid_x = 0 ans_mid_y = 0 ans_tang_x = 0 ans_tang_y = 0 count_x = 0 count_y = 0 tem_sample_1 = [] tem_sample_2 = [] rand = random.randint(0,1) for i in data: tem_x = dist(sample_1[0], sample_1[1], i[0], i[1]) tem_y = dist(sample_2[0], sample_2[1], i[0], i[1]) if tem_x < tem_y or (tem_x == tem_y and rand == 1): count_x += 1; ans_mid_x += i[0]; ans_tang_x += i[1] else: count_y += 1; ans_mid_y += i[0]; ans_tang_y += i[1] tem_sample_1 = [round((ans_mid_x / count_x),3), round((ans_tang_x / count_x),3)] tem_sample_2 = [round((ans_mid_y / count_y),3), round((ans_tang_y / count_y),3)] if tem_sample_1 == sample_1 and tem_sample_2 == sample_2: break else: sample_1 = tem_sample_1 sample_2 = tem_sample_2 print(sample_1) print(sample_2)
时间: 2024-01-23 19:03:10 浏览: 176
以下是将Python代码转换为C语言代码的示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
double dist(double x_mid, double x_tang, double y_mid, double y_tang) {
return fabs(x_mid - y_mid) + fabs(x_tang - y_tang);
}
int main() {
double data[30][2] = {
{0.697, 0.460}, {0.774, 0.376}, {0.634, 0.264},
{0.608, 0.318}, {0.556, 0.215}, {0.403, 0.237},
{0.481, 0.149}, {0.437, 0.211}, {0.666, 0.091},
{0.243, 0.267}, {0.245, 0.057}, {0.343, 0.099},
{0.639, 0.161}, {0.657, 0.198}, {0.360, 0.370},
{0.593, 0.042}, {0.719, 0.103}, {0.359, 0.188},
{0.339, 0.241}, {0.282, 0.257}, {0.748, 0.232},
{0.714, 0.346}, {0.483, 0.312}, {0.478, 0.437},
{0.525, 0.369}, {0.751, 0.489}, {0.532, 0.472},
{0.473, 0.376}, {0.725, 0.445}, {0.446, 0.459}
};
double* sample_1 = data[0];
double* sample_2 = data[1];
while (1) {
double ans_mid_x = 0;
double ans_mid_y = 0;
double ans_tang_x = 0;
double ans_tang_y = 0;
int count_x = 0;
int count_y = 0;
double tem_sample_1[2] = {0.0, 0.0};
double tem_sample_2[2] = {0.0, 0.0};
int rand_num = rand() % 2;
for (int i = 0; i < 30; i++) {
double tem_x = dist(sample_1[0], sample_1[1], data[i][0], data[i][1]);
double tem_y = dist(sample_2[0], sample_2[1], data[i][0], data[i][1]);
if (tem_x < tem_y || (tem_x == tem_y && rand_num == 1)) {
count_x++;
ans_mid_x += data[i][0];
ans_tang_x += data[i][1];
} else {
count_y++;
ans_mid_y += data[i][0];
ans_tang_y += data[i][1];
}
}
tem_sample_1[0] = round((ans_mid_x / count_x) * 1000) / 1000;
tem_sample_1[1] = round((ans_tang_x / count_x) * 1000) / 1000;
tem_sample_2[0] = round((ans_mid_y / count_y) * 1000) / 1000;
tem_sample_2[1] = round((ans_tang_y / count_y) * 1000) / 1000;
if (tem_sample_1[0] == sample_1[0] && tem_sample_1[1] == sample_1[1] &&
tem_sample_2[0] == sample_2[0] && tem_sample_2[1] == sample_2[1]) {
break;
} else {
sample_1 = tem_sample_1;
sample_2 = tem_sample_2;
}
}
printf("Sample 1: (%f, %f)\n", sample_1[0], sample_1[1]);
printf("Sample 2: (%f, %f)\n", sample_2[0], sample_2[1]);
return 0;
}
```
需要注意的是,在C语言中,需要引入头文件来使用各种函数和类型,如`<stdio.h>`和`<stdlib.h>`。此外,为了使用随机数,还需要引入`<time.h>`头文件,并使用`rand()`函数来生成随机数。在C语言中,数组的下标从0开始,因此需要将Python代码中的下标进行修改。还需要注意在进行浮点数运算时可能会出现精度问题,因此需要使用`round()`函数来四舍五入取整,并将结果保留到小数点后三位。
阅读全文
相关推荐
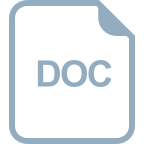
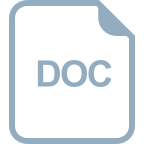
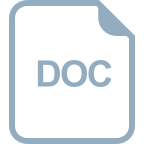
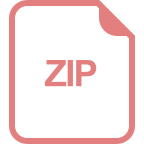
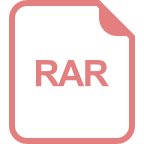
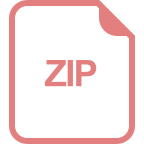
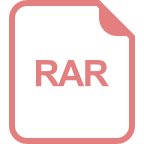
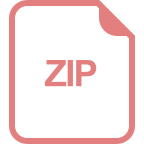
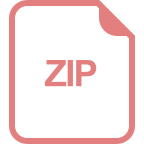
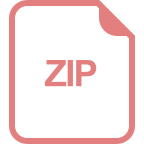
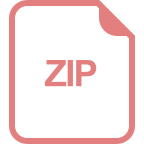
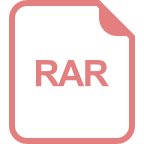
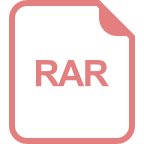
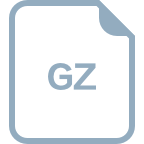
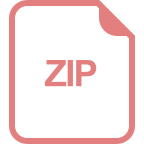
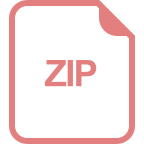
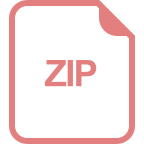
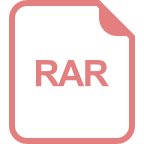
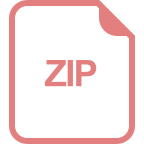