用代码形式打出:用Bootstrap制作一个网站,要求要有前后端交互与异步请求,后端可接入第三方接口实现功能(实现天气预报平台、地图导航平台、在线音乐平台等等),或连接自己的数据库实现相关功能、平台(学生选课系统、学生管理系统、部门管理、商品管理系统、订单管理系统、投票系统等等)
时间: 2024-01-28 14:02:55 浏览: 22
这是一个相对复杂的任务,需要使用多种技术和语言来实现。以下是一个简单的示例代码,演示了如何使用Bootstrap和Python Flask框架实现一个具有前后端交互和异步请求的网站,同时接入了第三方天气API:
HTML代码(index.html):
```html
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Weather App</title>
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="text-center mt-5">Weather App</h1>
<div class="row justify-content-center mt-5">
<div class="col-md-4">
<div class="form-group">
<label for="city">City:</label>
<input type="text" class="form-control" id="city" placeholder="Enter city">
</div>
<button type="button" class="btn btn-primary" onclick="getWeather()">Get Weather</button>
</div>
</div>
<div class="row justify-content-center mt-5">
<div class="col-md-4">
<div class="card" style="display: none;" id="weather-card">
<div class="card-body">
<h5 class="card-title" id="city-name"></h5>
<h6 class="card-subtitle mb-2 text-muted" id="weather-description"></h6>
<p class="card-text" id="temperature"></p>
</div>
</div>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
function getWeather() {
// 获取城市名
var city = $('#city').val();
// 发送异步请求获取天气数据
$.get('/weather', {'city': city}, function (response) {
// 将数据填充到页面上
$('#city-name').text(response['city']);
$('#weather-description').text(response['weather']);
$('#temperature').text('Temperature: ' + response['temperature'] + '°C');
// 显示天气卡片
$('#weather-card').show();
});
}
</script>
</body>
</html>
```
Python代码(app.py):
```python
from flask import Flask, render_template, request, jsonify
import requests
app = Flask(__name__)
# 天气API的URL和API Key
weather_api_url = 'https://api.openweathermap.org/data/2.5/weather'
weather_api_key = 'your-api-key-here'
# 主页路由
@app.route('/')
def index():
return render_template('index.html')
# 天气路由,接受城市名并返回天气数据
@app.route('/weather')
def weather():
# 获取城市名
city = request.args.get('city')
# 调用天气API获取天气数据
response = requests.get(weather_api_url, params={'q': city, 'appid': weather_api_key})
data = response.json()
# 解析天气数据并返回
weather_data = {
'city': data['name'],
'weather': data['weather'][0]['description'],
'temperature': round(data['main']['temp'] - 273.15, 1)
}
return jsonify(weather_data)
if __name__ == '__main__':
app.run()
```
在这个示例中,我们使用了Bootstrap的样式和组件来构建一个简单的天气应用。用户可以在输入框中输入城市名,然后点击按钮获取该城市的天气数据。在后端,我们使用Python Flask框架来处理请求和响应,并调用第三方天气API获取数据。在前端,我们使用JavaScript和jQuery来发送异步请求和更新页面内容。
注意:这个示例只是一个简单的实现,可能存在一些性能问题和改进的空间。如果你需要更高性能或更复杂的网站,需要使用更高级的技术和框架。
相关推荐
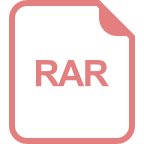
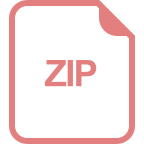
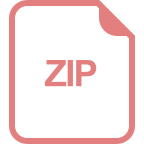














