写vue代码实现倒计时
时间: 2023-03-07 20:14:17 浏览: 98
我可以给你一些参考代码:<template>
<div>
<h1>倒计时示例:</h1>
<span>{{minutes}} 分 {{seconds}} 秒</span>
</div>
</template><script>
export default {
data() {
return {
minutes: 0,
seconds: 0
}
},
created() {
this.countdown();
},
methods: {
countdown() {
let vm = this;
setInterval(function() {
if (vm.seconds > 0) {
vm.seconds--;
}
if (vm.seconds === 0) {
if (vm.minutes === 0) {
clearInterval(this);
} else {
vm.minutes--;
vm.seconds = 59;
}
}
}, 1000);
}
}
}
</script>
相关问题
vue3实现倒计时功能
在Vue3中,你可以通过以下步骤实现倒计时功能:
1. 在data中定义倒计时相关的变量,如倒计时剩余时间countDown、是否停止倒计时isStop等。
2. 在mounted钩子函数中,调用setInterval函数,每秒更新countdown的值,当countdown为0时,将isStop设置为true,停止倒计时。
3. 在template中使用{{countDown}}来显示倒计时的剩余时间。
具体的代码如下:
```
<template>
<div>
{{ countDown }}
</div>
</template>
<script>
export default {
data() {
return {
countDown: 60,
isStop: false
};
},
mounted() {
setInterval(() => {
if (!this.isStop && this.countDown > 0) {
this.countDown--;
} else {
this.isStop = true;
}
}, 1000);
}
};
</script>
```
这样,每次组件渲染时都会显示当前的倒计时剩余时间,直到倒计时结束。
用vue实现验证码倒计时按钮
你可以使用Vue来实现验证码倒计时按钮。下面是一个简单的示例:
首先,在你的Vue组件中,你需要定义一个倒计时的变量和一个按钮状态的变量:
```vue
<template>
<div>
<button :disabled="countdown > 0" @click="startCountdown">{{ buttonText }}</button>
</div>
</template>
<script>
export default {
data() {
return {
countdown: 0,
buttonText: '获取验证码'
};
},
methods: {
startCountdown() {
this.countdown = 10; // 设置倒计时时间
this.buttonText = `${this.countdown} 秒后重新发送`;
const timer = setInterval(() => {
this.countdown--;
this.buttonText = `${this.countdown} 秒后重新发送`;
if (this.countdown === 0) {
clearInterval(timer);
this.buttonText = '获取验证码';
}
}, 1000);
}
}
};
</script>
```
在上面的代码中,我们使用了一个按钮和一个文本来显示倒计时信息。当点击按钮时,`startCountdown` 方法会被调用。在 `startCountdown` 方法中,我们使用 `setInterval` 来每秒更新倒计时时间,并根据时间的变化更新按钮的文本。当倒计时结束后,我们清除定时器并将按钮文本重置为初始状态。
请注意,这只是一个简单的示例,你可能需要根据你的实际需求进行修改和扩展。
相关推荐
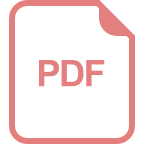
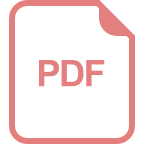
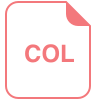
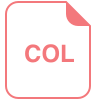
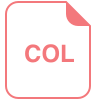
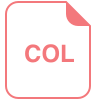
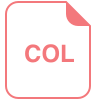







