C语言写合并CSV文件
时间: 2024-01-07 21:05:11 浏览: 69
以下是一个简单的C语言程序,用于合并两个CSV文件。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
int main(int argc, char* argv[]) {
if (argc != 4) {
printf("Usage: %s file1.csv file2.csv output.csv\n", argv[0]);
return 1;
}
FILE* file1 = fopen(argv[1], "r");
if (file1 == NULL) {
printf("Error: Could not open file %s\n", argv[1]);
return 1;
}
FILE* file2 = fopen(argv[2], "r");
if (file2 == NULL) {
printf("Error: Could not open file %s\n", argv[2]);
fclose(file1);
return 1;
}
FILE* output = fopen(argv[3], "w");
if (output == NULL) {
printf("Error: Could not open file %s\n", argv[3]);
fclose(file1);
fclose(file2);
return 1;
}
char line[MAX_LINE_LEN];
// 从file1读取一行,写入output
while (fgets(line, MAX_LINE_LEN, file1) != NULL) {
fputs(line, output);
}
// 从file2读取一行,写入output
while (fgets(line, MAX_LINE_LEN, file2) != NULL) {
fputs(line, output);
}
// 关闭所有文件
fclose(file1);
fclose(file2);
fclose(output);
return 0;
}
```
该程序接受三个参数:两个CSV文件的文件名和输出文件的文件名。它首先打开输入文件,然后从每个文件中读取一行并将其写入输出文件。最后,它关闭所有文件并返回0。
阅读全文
相关推荐
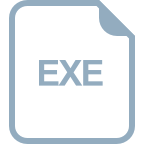



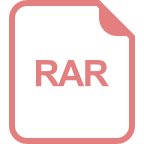
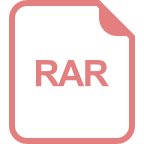
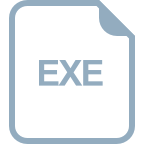
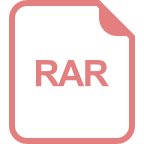
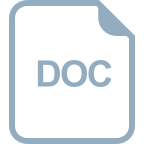
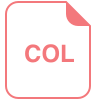
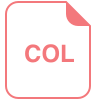
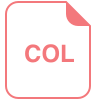
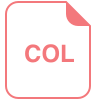
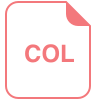
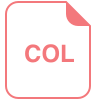
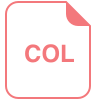
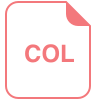