使用android studio 设计一个xml页面,要求: 1.页面全局使用LinearLayout布局;页面上方占用总页面的20%,页面上方使用LinearLayout,内容为textview文本框; 2.页面下方左侧占比30%使用RecycleViewAdapter,画新的xml文件,内容为使用LinearLayout填充4个button按钮,分别命名为b1,b2,b3,b4; 3.页面下方右侧占比70%为4个不同的fragment页面,分别为命名为f1,f2,f3,f4,按不同的button按钮显示不同的fragment页面
时间: 2024-03-05 19:54:31 浏览: 59
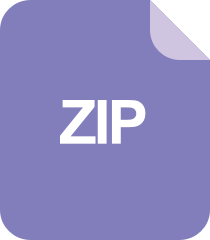
Android开发课堂作业记单词APP登录注册页面跳转
好的,我会尽力回答你的问题,以下是答案:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<!-- 页面上方占用总页面的20% -->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="20"
android:orientation="vertical">
<!-- 页面上方使用LinearLayout,内容为textview文本框 -->
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="页面上方的文本框" />
</LinearLayout>
<!-- 页面下方占比30%使用RecycleViewAdapter -->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="30"
android:orientation="horizontal">
<!-- 左侧占比30% -->
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="3"
android:orientation="vertical">
<!-- 画新的xml文件,内容为使用LinearLayout填充4个button按钮,分别命名为b1,b2,b3,b4 -->
<Button
android:id="@+id/b1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="按钮1" />
<Button
android:id="@+id/b2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="按钮2" />
<Button
android:id="@+id/b3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="按钮3" />
<Button
android:id="@+id/b4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="按钮4" />
</LinearLayout>
<!-- 右侧占比70%为4个不同的fragment页面,分别为命名为f1,f2,f3,f4,按不同的button按钮显示不同的fragment页面 -->
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="7" />
</LinearLayout>
</LinearLayout>
```
在Java代码中,你需要为四个按钮设置点击监听器,并在监听器中切换Fragment,以下是示例代码:
```java
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Button mB1, mB2, mB3, mB4;
private FrameLayout mFragmentContainer;
private Fragment mF1, mF2, mF3, mF4;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mB1 = findViewById(R.id.b1);
mB2 = findViewById(R.id.b2);
mB3 = findViewById(R.id.b3);
mB4 = findViewById(R.id.b4);
mFragmentContainer = findViewById(R.id.fragment_container);
mB1.setOnClickListener(this);
mB2.setOnClickListener(this);
mB3.setOnClickListener(this);
mB4.setOnClickListener(this);
mF1 = new Fragment1();
mF2 = new Fragment2();
mF3 = new Fragment3();
mF4 = new Fragment4();
getSupportFragmentManager()
.beginTransaction()
.add(R.id.fragment_container, mF1)
.add(R.id.fragment_container, mF2)
.add(R.id.fragment_container, mF3)
.add(R.id.fragment_container, mF4)
.hide(mF1)
.hide(mF2)
.hide(mF3)
.hide(mF4)
.commit();
// 默认显示第一个 Fragment
showFragment(mF1);
}
@Override
public void onClick(View view) {
switch (view.getId()) {
case R.id.b1:
showFragment(mF1);
break;
case R.id.b2:
showFragment(mF2);
break;
case R.id.b3:
showFragment(mF3);
break;
case R.id.b4:
showFragment(mF4);
break;
}
}
private void showFragment(Fragment fragment) {
getSupportFragmentManager()
.beginTransaction()
.hide(mF1)
.hide(mF2)
.hide(mF3)
.hide(mF4)
.show(fragment)
.commit();
}
}
```
在上面的代码中,`Fragment1`、`Fragment2`、`Fragment3`、`Fragment4` 分别代表四个不同的 Fragment 页面,你需要自己实现这四个 Fragment 的布局和逻辑。在 `onCreate` 方法中,我使用 `add` 方法将这四个 Fragment 添加到 `FrameLayout` 中,并默认显示第一个 Fragment。在点击按钮的时候,调用 `showFragment` 方法来切换显示不同的 Fragment。
阅读全文
相关推荐
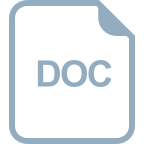
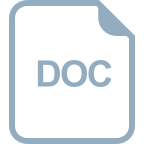


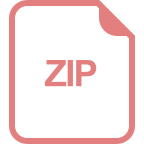
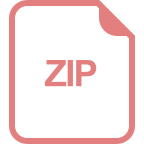
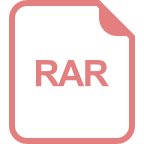
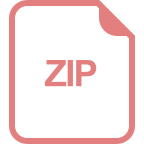
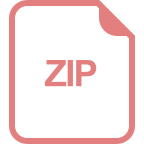
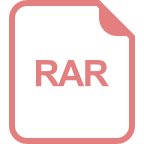
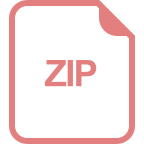
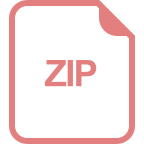
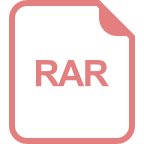
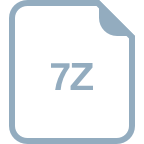

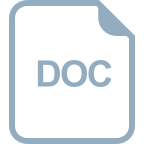
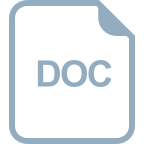