用C++语言帮我写一个利用线性表实现通讯录管理
时间: 2023-05-25 10:01:49 浏览: 93
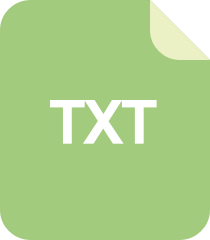
用线性表实现的通讯录,C++代码实现

程序。
好的,以下是利用线性表实现通讯录管理程序的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_PHONE_LEN 11
typedef struct contact {
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
struct contact *next;
} Contact;
// 初始化通讯录为空
Contact *init_contact() {
Contact *head = (Contact*) malloc(sizeof(Contact));
head->next = NULL;
return head;
}
// 添加联系人到通讯录
void add_contact(Contact *head, char *name, char *phone) {
Contact *new_contact = (Contact*) malloc(sizeof(Contact));
strcpy(new_contact->name, name);
strcpy(new_contact->phone, phone);
new_contact->next = head->next;
head->next = new_contact;
printf("Contact added successfully.\n");
}
// 查找指定联系人
void search_contact(Contact *head, char *name) {
Contact *cur_contact = head->next;
int flag = 0;
while (cur_contact != NULL) {
if (strcmp(cur_contact->name, name) == 0) {
printf("Name: %s, Phone: %s\n", cur_contact->name, cur_contact->phone);
flag = 1;
break;
}
cur_contact = cur_contact->next;
}
if (!flag) {
printf("Contact not found.\n");
}
}
// 修改指定联系人的电话号码
void modify_contact(Contact *head, char *name, char *new_phone) {
Contact *cur_contact = head->next;
int flag = 0;
while (cur_contact != NULL) {
if (strcmp(cur_contact->name, name) == 0) {
strcpy(cur_contact->phone, new_phone);
printf("Phone number modified successfully.\n");
flag = 1;
break;
}
cur_contact = cur_contact->next;
}
if (!flag) {
printf("Contact not found.\n");
}
}
// 删除指定联系人
void delete_contact(Contact *head, char *name) {
Contact *cur_contact = head->next;
Contact *pre_contact = head;
int flag = 0;
while (cur_contact != NULL) {
if (strcmp(cur_contact->name, name) == 0) {
pre_contact->next = cur_contact->next;
free(cur_contact);
printf("Contact deleted successfully.\n");
flag = 1;
break;
}
pre_contact = cur_contact;
cur_contact = cur_contact->next;
}
if (!flag) {
printf("Contact not found.\n");
}
}
// 输出通讯录中的所有联系人
void print_contact(Contact *head) {
Contact *cur_contact = head->next;
if (cur_contact == NULL) {
printf("Contact list is empty.\n");
} else {
printf("Name\tPhone\n");
while (cur_contact != NULL) {
printf("%s\t%s\n", cur_contact->name, cur_contact->phone);
cur_contact = cur_contact->next;
}
}
}
int main() {
Contact *head = init_contact();
int choice;
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
char new_phone[MAX_PHONE_LEN];
while (1) {
printf("\n-- Contact Management System --\n");
printf("[1] Add contact\n");
printf("[2] Search contact\n");
printf("[3] Modify phone number\n");
printf("[4] Delete contact\n");
printf("[5] Print contact list\n");
printf("[0] Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1: // 添加联系人
printf("Enter name: ");
scanf("%s", name);
printf("Enter phone number: ");
scanf("%s", phone);
add_contact(head, name, phone);
break;
case 2: // 查找联系人
printf("Enter name: ");
scanf("%s", name);
search_contact(head, name);
break;
case 3: // 修改电话号码
printf("Enter name: ");
scanf("%s", name);
printf("Enter new phone number: ");
scanf("%s", new_phone);
modify_contact(head, name, new_phone);
break;
case 4: // 删除联系人
printf("Enter name: ");
scanf("%s", name);
delete_contact(head, name);
break;
case 5: // 输出所有联系人
print_contact(head);
break;
case 0: // 退出程序
printf("Exiting Contact Management System.\n");
exit(0);
default:
printf("Invalid choice, please try again.\n");
break;
}
}
return 0;
}
```
这是一个基于命令行界面的通讯录管理程序,支持添加、查找、修改、删除联系人,并且可以输出整个通讯录中的所有联系人。通过链表来实现联系人的添加、删除等操作,程序运行效率较高,并且可以处理较大量级的通讯录数据。
阅读全文
相关推荐
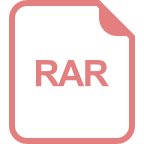

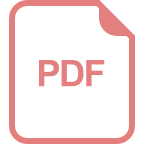
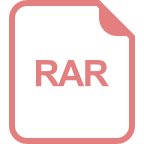
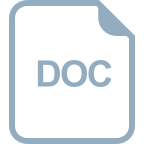
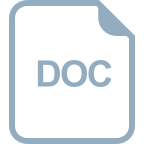
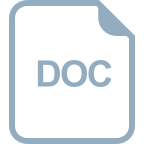



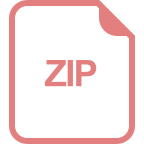
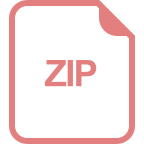
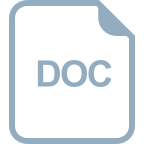
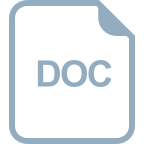
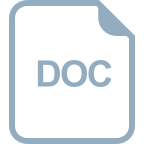