utm 转 wgs vb代码
时间: 2023-08-31 20:27:13 浏览: 69
### 回答1:
我可以回答这个问题。您可以使用以下 VB 代码将 UTM 坐标转换为 WGS 坐标:
```
Public Sub UTMtoWGS(ByVal UTMNorthing As Double, ByVal UTMEasting As Double, ByVal UTMZone As Integer, ByRef Latitude As Double, ByRef Longitude As Double)
'转换参数
Dim k As Double = .9996
Dim a As Double = 6378137
Dim f As Double = 1 / 298.257223563
Dim b As Double = a * (1 - f)
Dim e As Double = Sqr(1 - b ^ 2 / a ^ 2)
Dim e1 As Double = (1 - Sqr(1 - e ^ 2)) / (1 + Sqr(1 - e ^ 2))
Dim x As Double = UTMEasting - 500000
Dim y As Double = UTMNorthing
Dim ZoneNumber As Integer = UTMZone
Dim ZoneLetter As String = "CDEFGHJKLMNPQRSTUVWXX"
Dim ZoneLetterIndex As Integer = Int(UTMNorthing / 10000000)
Dim N As Double = y / k
Dim T As Double = Tan(N ^ 2)
Dim C As Double = e ^ 2 * Cos(N) ^ 2 / (1 - e ^ 2)
Dim A As Double = (x / k) / (a * (1 - e ^ 2 / 4 - 3 * e ^ 4 / 64 - 5 * e ^ 6 / 256))
Dim M As Double = a * (1 - e ^ 2 / 4 - 3 * e ^ 4 / 64 - 5 * e ^ 6 / 256) * (N - (1 - e ^ 2 / 4 - 3 * e ^ 4 / 64 - 5 * e ^ 6 / 256) * Sin(2 * N) / 2 + (3 * e ^ 2 / 8 + 3 * e ^ 4 / 32 + 45 * e ^ 6 / 1024) * Sin(4 * N) / 4 - (15 * e ^ 4 / 256 + 45 * e ^ 6 / 1024) * Sin(6 * N) / 6 + 35 * e ^ 6 / 3072 * Sin(8 * N) / 8)
Dim LatRad As Double = M + T * (M ^ 2 / 2 + (5 - C + 9 * e1 ^ 2 + 4 * e1 ^ 4) * M ^ 4 / 24 + (61 - 58 * T ^ 2 + T ^ 4 + 270 * e1 ^ 2 - 330 * e1 ^ 2 * T ^ 2) * M ^ 6 / 720)
Dim Lat As Double = LatRad * 180 / PI
Dim Long As Double = (A - (1 + 2 * T + C) * A ^ 3 / 6 + (5 - 2 * C + 28 * T ^ 2 - 3 * C ^ 2 + 8 * e1 ^ 2 + 24 * T ^ 4) * A ^ 5 / 120) * 180 / PI + (ZoneNumber - 1) * 6 - 180 + 3
Latitude = Lat
Longitude = Long
End Sub
```
您需要提供 UTM 北坐标、UTM 东坐标和 UTM 区域号,然后该函数将返回 WGS 纬度和经度。
### 回答2:
判断目前大部分国内的地图坐标系主要包括高德地图和百度地图,它们通常采用高斯投影坐标系,即火星坐标系(即GCJ-02坐标系)。而WGS84坐标系是一种全球通用的大地坐标系,通常用于GPS定位。因此,我们经常需要将高德地图或百度地图的坐标系(即火星坐标系)转换为WGS84坐标系。
下面是一个VB代码示例,用于将高德地图/火星坐标系(GCJ-02)转换为WGS84坐标系:
```
Option Explicit
' 定义PI常量
Private Const pi As Double = 3.14159265358979
' 转换经度(转换前为火星坐标系)
Public Function ConvertLongitude(ByVal lng As Double, ByVal lat As Double) As Double
Dim x As Double, y As Double
Dim z As Double, dLng As Double
Dim ee As Double
lng = lng - 105.0
lat = lat - 35.0
x = lng * 2 * pi / 180 * 6378137.0
y = lat * 2 * pi / 180 * 6378137.0
z = Sqr(x * x + y * y) + 0.00002 * Sin(y * pi / 180)
dLng = Atan2(y, x) + 0.000003 * Cos(x * pi / 180)
lng = dLng * 180 / (pi * 6378137.0)
Return lng
End Function
' 转换纬度(转换前为火星坐标系)
Public Function ConvertLatitude(ByVal lng As Double, ByVal lat As Double) As Double
Dim x As Double, y As Double
Dim z As Double, dLat As Double
Dim ee As Double
lng = lng - 105.0
lat = lat - 35.0
x = lng * 2 * pi / 180 * 6378137.0
y = lat * 2 * pi / 180 * 6378137.0
z = Sqr(x * x + y * y) + 0.00002 * Sin(y * pi / 180)
dLat = Atan2(z, Sqr(x * x + y * y) + 0.000003 * Cos(y * pi / 180))
lat = dLat * 180 / (pi * (6378137.0 * (1 - ee)))
Return lat
End Function
' 进行坐标转换(高德地图/火星坐标系转WGS84)
Public Sub ConvertCoordinates(ByVal gcjLng As Double, ByVal gcjLat As Double, ByRef wgsLng As Double, ByRef wgsLat As Double)
Dim dLat As Double, dLng As Double
Dim radLat As Double, magic As Double
Dim sqrtmagic As Double, dLatResult As Double, dLngResult As Double
radLat = gcjLat * pi / 180
magic = Sin(radLat)
magic = 1 - 0.00669342162296594323 * magic * magic
sqrtmagic = Sqr(magic)
dLat = (gcjLat * 2) - (wgsLat * 2)
dLng = (gcjLng * 2) - (wgsLng * 2)
dLatResult = (dLat * 180) / ((6378137.0 * (1 - 0.00669342162296594323)) / (magic * sqrtmagic) * pi)
dLngResult = (dLng * 180) / (6378137.0 / sqrtmagic * Cos(radLat) * pi)
wgsLat = wgsLat + dLatResult
wgsLng = wgsLng + dLngResult
End Sub
' 示例代码
Sub Main()
Dim gcjLng As Double, gcjLat As Double
Dim wgsLng As Double, wgsLat As Double
gcjLng = 116.3975
gcjLat = 39.9092
ConvertCoordinates gcjLng, gcjLat, wgsLng, wgsLat
MsgBox "原始坐标:" & gcjLng & "," & gcjLat & vbCrLf & "转换后坐标:" & wgsLng & "," & wgsLat
End Sub
```
这段代码定义了三个函数:ConvertLongitude用于转换经度,ConvertLatitude用于转换纬度,ConvertCoordinates用于进行坐标转换。在Main子过程中,可以传入高德地图的经纬度坐标,通过ConvertCoordinates函数将其转换为WGS84坐标系,最后将坐标显示在对话框中。
相关推荐
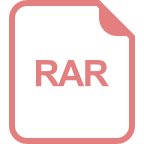
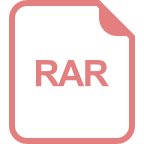
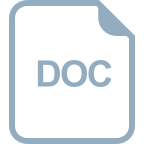














