public class DataBaseContext : DbContext { public DbSet<User> Users { get; set; } public DbSet<Cabin> Cabins { get; set; } public DbSet<Sensor> Sensors { get; set; } public DbSet<CommuServer> CommuServers { get; set; } public DbSet<ComServerPort> Ports { get; set; } public DbSet<CoreBoard> CoreBoards { get; set; } public DbSet<HullInfo> Hulls { get; set; } public DataBaseContext() : base("name=MyConnectionString") { } } /// <summary> /// 舱体 /// </summary> public class Cabin { /// <summary> /// 舱体ID /// </summary> [DatabaseGenerated(DatabaseGeneratedOption.Identity)] public int Id { get; set; } /// <summary> /// 舱体名称 /// </summary> [Key] public string Name { get; set; } /// <summary> /// 舱体内的通信设备 /// </summary> public virtual ICollection<CommuServer> CommuServers { get; set; } /// <summary> /// 核心板 /// </summary> public virtual CoreBoard CoreBoard { get; set; } }如何通过Cabin获取CoreBoard
时间: 2024-02-03 19:02:27 浏览: 95
可以通过以下代码来获取Cabin的CoreBoard:
```
var cabin = dbContext.Cabins.Include(c => c.CoreBoard).FirstOrDefault(c => c.Id == cabinId);
var coreBoard = cabin.CoreBoard;
```
其中,`dbContext`是`DataBaseContext`的实例,`cabinId`是需要查询的Cabin的Id。`Include`方法用于指定需要包含在查询结果中的导航属性,这里是`CoreBoard`。`FirstOrDefault`方法用于查找符合条件的第一个实体,这里是根据`Id`查找Cabin。最后,通过`cabin.CoreBoard`获取对应的CoreBoard实例。
相关问题
c# code:public class APPServerContext : DbContext { public readonly string DBAias; public readonly string UserID; public APPServerContext(string dbaias, string userid); public bool IsSqlServer { get; } public DbSet<sysBatchCodeNO> SYS_BATCHCODENO { get; set; } public DbSet<sysListCodeNO> SYS_LISTCODENO { get; set; } public DbSet<SYS_ORGSTRUCTURE> SYS_ORGSTRUCTURE { get; set; } public DbSet<sysPrgWorkbench> SYS_PRGWORKBENCH { get; set; } public DbSet<sysBarCodeNO> SYS_BARCODENO { get; set; } public DbSet<SYS_LOGINUSER> SYS_LOGINUSERS { get; set; } public bool IsMySql { get; } public DbSet<SYS_ENANNOU> SYS_ENANNOUS { get; set; } public bool IsOracle { get; } public DbParameter CreateParameter(string Key, object Value); public override void Dispose(); public string GetDBParName(string parameterName); public Hashtable PBValue(string KeyList); protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder); } using APPServerContext context1 = new APPServerContext(LoginInfo.DBAias, LoginInfo.UserID);await context1.Database.BeginTransactionAsync().ConfigureAwait(false); context1.Database.SqlQuery(exeSqlList[i]); error : Execution Timeout Expired. The timeout period elapsed prior to completion of the operation or the server is not responding. question :APPServerContext does not contain CommandTimeOut. How do I handle this error
You can set the Command Timeout value in the DbContext's configuration options using the "CommandTimeout" property. You can do this either in the OnConfiguring method or when creating a new instance of DbContextOptionsBuilder. Here's an example of setting the Command Timeout value to 60 seconds:
```
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer(connectionString)
.ConfigureWarnings(warnings => warnings.Throw(RelationalEventId.QueryClientEvaluationWarning))
.CommandTimeout(60);
}
```
Alternatively, you can also set the Command Timeout value when executing the SQL query using the "CommandTimeout" property of the DbCommand object. Here's an example:
```
using APPServerContext context1 = new APPServerContext(LoginInfo.DBAias, LoginInfo.UserID);
await context1.Database.BeginTransactionAsync().ConfigureAwait(false);
var command = context1.Database.GetDbConnection().CreateCommand();
command.CommandText = exeSqlList[i];
command.CommandTimeout = 60;
context1.Database.OpenConnection();
var result = await command.ExecuteNonQueryAsync().ConfigureAwait(false);
```
This will set the Command Timeout value to 60 seconds for the current SQL query execution.
DbSet<TEntity> IDbContext.Set<TEntity>() => Set<TEntity>();这个是在做什么
`IDbContext` 接口的实现类中的 `Set<TEntity>()` 方法,返回一个 `DbSet<TEntity>` 类型的对象。该方法的主要作用是获取一个特定类型的实体集合对象,以便对该实体集合进行 CRUD 操作。
在使用 Entity Framework Core 进行数据访问时,我们通常需要通过 `DbContext` 实例来获取一个实体集合,然后对该实体集合进行 CRUD 操作。而 `Set<TEntity>()` 方法就是用来获取该实体集合的方法。
在实现 `IDbContext` 接口时,我们需要将 `Set<TEntity>()` 方法实现为 `Set<TEntity>` 属性的访问器,即调用 `Set<TEntity>` 属性的 getter 方法即可返回该实体集合。由于 `DbSet<TEntity>` 类型本身就实现了 `IQueryable<TEntity>` 接口,因此我们可以直接在返回的 `DbSet<TEntity>` 对象上进行 LINQ 查询和其他数据访问操作。
阅读全文
相关推荐
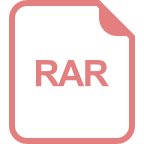
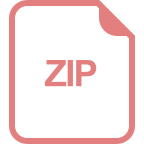












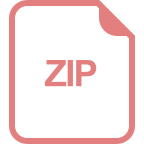
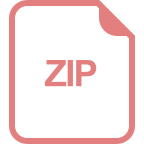