图书管理系统设计与实现
时间: 2023-10-23 18:08:55 浏览: 24
1.需求分析
1.1 用户需求
1.2 功能需求
1.3 性能需求
1.4 安全需求
2.系统设计
2.1 系统架构设计
2.2 数据库设计
2.3 界面设计
2.4 功能设计
3.系统实现
3.1 环境配置
3.2 数据库实现
3.3 功能实现
3.4 界面实现
4.系统测试
4.1 功能测试
4.2 性能测试
4.3 安全测试
5.系统部署
5.1 系统安装
5.2 数据库部署
5.3 界面部署
5.4 功能部署
6.系统维护
6.1 系统优化
6.2 数据备份
6.3 故障排除
6.4 安全维护
7.总结
7.1 实现效果
7.2 难点分析
7.3 优化建议
相关问题
图书管理系统设计与实现单链表
好的,我可以和你讨论关于图书管理系统设计与实现单链表的问题。
首先,单链表是一种常见的数据结构,用于存储一系列的数据元素。在图书管理系统中,我们可以使用单链表来存储图书信息。
单链表由多个节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。在图书管理系统中,每个节点可以存储一本书的信息,例如书名、作者、出版社、ISBN号等等。
我们可以使用一个头指针来指向链表的第一个节点。通过遍历链表,我们可以实现对图书信息的增、删、改、查等操作。
下面是一个简单的示例代码,用于实现单链表存储图书信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Book {
char title[50];
char author[50];
char publisher[50];
char isbn[20];
struct Book *next;
};
struct Book *head = NULL;
void add_book() {
struct Book *new_book = malloc(sizeof(struct Book));
printf("Please enter the book title: ");
scanf("%s", new_book->title);
printf("Please enter the book author: ");
scanf("%s", new_book->author);
printf("Please enter the book publisher: ");
scanf("%s", new_book->publisher);
printf("Please enter the book ISBN: ");
scanf("%s", new_book->isbn);
new_book->next = NULL;
if (head == NULL) {
head = new_book;
} else {
struct Book *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_book;
}
printf("Book added successfully!\n");
}
void list_books() {
if (head == NULL) {
printf("No books in the library!\n");
return;
}
printf("%-50s %-50s %-50s %-20s\n", "Title", "Author", "Publisher", "ISBN");
struct Book *current = head;
while (current != NULL) {
printf("%-50s %-50s %-50s %-20s\n", current->title, current->author, current->publisher, current->isbn);
current = current->next;
}
}
void search_book() {
char isbn[20];
printf("Please enter the ISBN of the book you want to search: ");
scanf("%s", isbn);
struct Book *current = head;
while (current != NULL) {
if (strcmp(current->isbn, isbn) == 0) {
printf("%-50s %-50s %-50s %-20s\n", "Title", "Author", "Publisher", "ISBN");
printf("%-50s %-50s %-50s %-20s\n", current->title, current->author, current->publisher, current->isbn);
return;
}
current = current->next;
}
printf("Book with ISBN %s not found!\n", isbn);
}
void delete_book() {
char isbn[20];
printf("Please enter the ISBN of the book you want to delete: ");
scanf("%s", isbn);
if (head == NULL) {
printf("No books in the library!\n");
return;
}
if (strcmp(head->isbn, isbn) == 0) {
struct Book *temp = head;
head = head->next;
free(temp);
printf("Book deleted successfully!\n");
return;
}
struct Book *current = head;
while (current->next != NULL) {
if (strcmp(current->next->isbn, isbn) == 0) {
struct Book *temp = current->next;
current->next = current->next->next;
free(temp);
printf("Book deleted successfully!\n");
return;
}
current = current->next;
}
printf("Book with ISBN %s not found!\n", isbn);
}
void update_book() {
char isbn[20];
printf("Please enter the ISBN of the book you want to update: ");
scanf("%s", isbn);
struct Book *current = head;
while (current != NULL) {
if (strcmp(current->isbn, isbn) == 0) {
printf("Please enter the new book title: ");
scanf("%s", current->title);
printf("Please enter the new book author: ");
scanf("%s", current->author);
printf("Please enter the new book publisher: ");
scanf("%s", current->publisher);
printf("Please enter the new book ISBN: ");
scanf("%s", current->isbn);
printf("Book updated successfully!\n");
return;
}
current = current->next;
}
printf("Book with ISBN %s not found!\n", isbn);
}
int main() {
int option;
do {
printf("Please select an option:\n");
printf("1. Add a book to the library\n");
printf("2. List all books in the library\n");
printf("3. Search for a book by ISBN\n");
printf("4. Delete a book from the library\n");
printf("5. Update a book in the library\n");
printf("6. Exit\n");
printf("Your selection: ");
scanf("%d", &option);
switch (option) {
case 1:
add_book();
break;
case 2:
list_books();
break;
case 3:
search_book();
break;
case 4:
delete_book();
break;
case 5:
update_book();
break;
case 6:
printf("Goodbye!\n");
break;
default:
printf("Invalid option!\n");
break;
}
} while (option != 6);
return 0;
}
```
在这个示例代码中,我们定义了一个Book结构体,包含了书的各个信息和指向下一个节点的指针。我们还定义了一个头指针head,用于指向链表的第一个节点。
我们通过add_book()函数实现了向链表中添加图书的功能,通过list_books()函数实现了列出所有图书的功能,通过search_book()函数实现了通过ISBN号搜索图书的功能,通过delete_book()函数实现了删除指定图书的功能,通过update_book()函数实现了更新指定图书信息的功能。
在main()函数中,我们通过一个循环来实现了用户与程序的交互,让用户能够选择相应的操作。
基于python web的图书管理系统设计与实现
基于Python Web的图书管理系统设计与实现可以使用Django框架来完成。下面是该系统的设计和实现步骤:
1.需求分析
该系统需要实现以下功能:
- 用户登录、注册、修改密码等操作
- 图书的添加、删除、修改、查询等操作
- 图书的分类、排序、分页等功能
2.设计架构
该系统的架构采用MVT模式,即Model-View-Template模式。其中,Model用于定义数据模型,View用于处理用户请求并返回响应,Template用于渲染页面。
3.数据模型
该系统的数据模型包括用户模型、书籍模型和图片模型。其中,用户模型包括用户名、密码、邮箱等字段;书籍模型包括书名、作者、出版社、价格等字段;图片模型包括图片名称、图片路径等字段。
4.关键代码说明
- 路由部分:在urls.py文件中定义了系统的路由,包括用户登录、注册、修改密码等操作的路由,以及图书的添加、删除、修改、查询等操作的路由。
- 重设密码部分:在views.py文件中定义了重设密码的函数,该函数通过验证用户的邮箱地址来重设密码。
- 添加书籍部分:在views.py文件中定义了添加书籍的函数,该函数通过表单获取用户输入的书籍信息,并将其保存到数据库中。
- 书籍列表部分:在views.py文件中定义了书籍列表的函数,该函数从数据库中获取所有的书籍信息,并将其渲染到页面上。
相关推荐
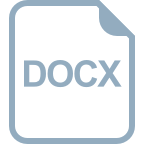












