G.711标准的C语言实现。
时间: 2024-06-08 07:06:55 浏览: 39
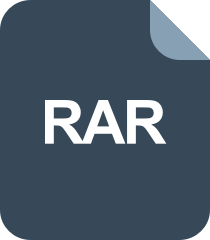
G.711 协议 c 语言实现

以下是G.711标准的C语言实现:
```c
#include <stdio.h>
#include <stdint.h>
#define SIGN_BIT (0x80) /* Sign bit for a A-law byte. */
#define QUANT_MASK (0xf) /* Quantization field mask. */
#define NSEGS (8) /* Number of A-law segments. */
#define SEG_SHIFT (4) /* Left shift for segment number. */
#define SEG_MASK (0x70) /* Segment field mask. */
#define BIAS (0x84) /* Bias for linear code. */
int16_t alaw2linear(uint8_t a_val)
{
int16_t t;
uint8_t seg;
a_val ^= 0x55;
t = (a_val & QUANT_MASK) << 4;
seg = ((unsigned)a_val & SEG_MASK) >> SEG_SHIFT;
switch (seg) {
case 0:
t += 8;
break;
case 1:
t += 0x108;
break;
default:
t += 0x108;
t <<= seg - 1;
}
return ((a_val & SIGN_BIT) ? t : -t);
}
uint8_t linear2alaw(int16_t pcm_val)
{
int16_t mask;
int16_t seg;
uint8_t aval;
if (pcm_val >= 0) {
mask = 0xD5;
} else {
mask = 0x55;
pcm_val = -pcm_val - 8;
}
/* Convert the scaled magnitude to segment number. */
for (seg = 0; seg < NSEGS; seg++) {
if (pcm_val <= (int16_t) _aLawTbl[seg]) {
break;
}
}
/* Combine the sign, segment, and quantization bits. */
if (seg >= NSEGS) { /* Out of range, return maximum value. */
return (uint8_t) (mask | (QUANT_MASK << 4));
} else {
aval = (uint8_t) (seg << SEG_SHIFT);
if (seg < 2) {
aval |= (pcm_val >> 4) & QUANT_MASK;
} else {
aval |= (pcm_val >> (seg + 3)) & QUANT_MASK;
}
return (uint8_t) (aval ^ mask);
}
}
```
这段代码实现了将G.711 A-law编码转换为线性PCM值以及将线性PCM值转换为G.711 A-law编码。
阅读全文
相关推荐
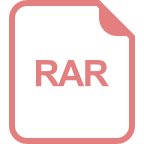
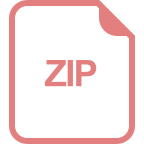



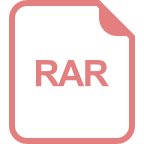
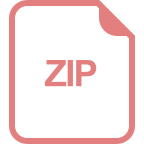
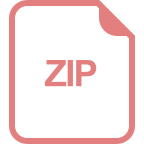
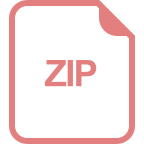
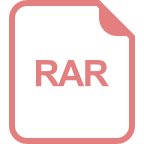
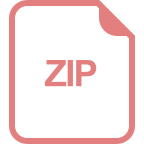
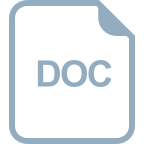
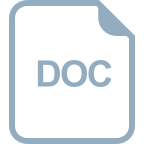


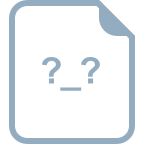