springboot实现token
时间: 2023-11-05 12:56:23 浏览: 145
Spring Boot可以使用Spring Security框架实现Token认证,下面是一个简单的示例:
1. 添加依赖
在pom.xml中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 配置Security
在Spring Boot的配置文件中添加以下配置:
```
# 关闭CSRF保护
security.enable-csrf=false
# 配置登录地址和拦截路径
security.oauth2.resource.filter-order=3
security.oauth2.resource.user-info-uri=http://localhost:8080/oauth/userinfo
security.oauth2.client.access-token-uri=http://localhost:8080/oauth/token
security.oauth2.client.client-id=clientapp
security.oauth2.client.client-secret=123456
security.oauth2.client.grant-type=client_credentials
security.oauth2.client.scope=read
```
3. 创建Token
在应用程序中创建Token,可以使用JWT(JSON Web Token)。
使用JWT创建Token的步骤如下:
- 创建JWT Header
- 创建JWT Payload
- 使用所选算法签名JWT Header和JWT Payload
可以使用以下代码创建JWT Token:
```
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
public class JwtUtil {
private final String secret = "mysecretkey";
public String generateToken(String username) {
Date now = new Date();
Date expiryDate = new Date(now.getTime() + 3600 * 1000);
return Jwts.builder()
.setSubject(username)
.setIssuedAt(new Date())
.setExpiration(expiryDate)
.signWith(SignatureAlgorithm.HS512, secret)
.compact();
}
}
```
4. 验证Token
在Spring Security中,可以使用Filter来验证Token。可以创建一个TokenAuthenticationFilter来实现Token验证。
TokenAuthenticationFilter的作用是在HTTP请求中获取Token,并将Token转换为Authentication对象。
可以使用以下代码创建TokenAuthenticationFilter:
```
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.web.authentication.AbstractAuthenticationProcessingFilter;
import org.springframework.security.web.util.matcher.AntPathRequestMatcher;
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class TokenAuthenticationFilter extends AbstractAuthenticationProcessingFilter {
public TokenAuthenticationFilter(String defaultFilterProcessesUrl, AuthenticationManager authManager) {
super(new AntPathRequestMatcher(defaultFilterProcessesUrl));
setAuthenticationManager(authManager);
}
@Override
public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response) {
String token = request.getHeader("Authorization");
if (token == null) {
throw new TokenException("Token not found");
}
Authentication authentication = new UsernamePasswordAuthenticationToken(token, token);
return getAuthenticationManager().authenticate(authentication);
}
@Override
protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response, FilterChain chain, Authentication authResult) throws IOException, ServletException {
SecurityContextHolder.getContext().setAuthentication(authResult);
chain.doFilter(request, response);
}
}
```
5. 配置Security
在Spring Security的配置中,可以添加TokenAuthenticationFilter来实现Token验证。
可以使用以下代码配置Security:
```
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpMethod;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomUserDetailsService customUserDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers(HttpMethod.POST, "/api/authenticate").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new TokenAuthenticationFilter("/api/**", authenticationManager()))
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(customUserDetailsService).passwordEncoder(passwordEncoder());
}
@Override
protected AuthenticationManager authenticationManager() throws Exception {
return super.authenticationManager();
}
private PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
在上述代码中,TokenAuthenticationFilter添加到了Spring Security的过滤器链中,并且设置了过滤路径为/api/**。在configure方法中,还添加了一个自定义的UserDetailsService,用于从数据库中获取用户信息。
以上是一个简单的Token认证实现示例,实际应用中还需要对Token进行过期时间验证、Token存储、Token刷新等处理。
阅读全文
相关推荐
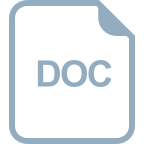
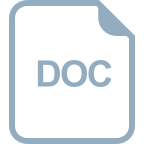
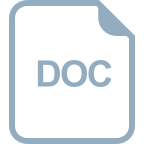


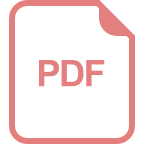
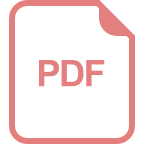











