vue3 ts directive
时间: 2023-06-23 17:58:47 浏览: 102
Vue 3中的directive可以通过TypeScript进行类型检查。在Vue 3中,指令的类型定义可以通过以下方式进行:
```typescript
import { Directive, DirectiveBinding } from 'vue';
const myDirective: Directive = {
mounted(el: HTMLElement, binding: DirectiveBinding) {
// 指令绑定到元素上时的逻辑
},
updated(el: HTMLElement, binding: DirectiveBinding) {
// 元素更新时的逻辑
}
};
export default myDirective;
```
在上面的代码中,我们首先引入了Vue 3的Directive和DirectiveBinding类型。然后,定义了一个myDirective指令,它包含了mounted和updated两个生命周期钩子函数。在这两个函数中,我们可以使用el和binding参数来操作元素和指令的绑定值。最后,我们通过export default将myDirective指令导出,以便在其他组件中使用。
需要注意的是,如果我们在使用自定义指令时没有进行类型检查,那么在模板中使用该指令时,TypeScript编译器可能会给出警告。因此,建议在使用指令时,尽可能地进行类型检查,以保证代码的健壮性和可维护性。
相关问题
program a login page with vue ,ts , element ui
Sure, here's an example of how to program a login page with Vue.js, TypeScript, and Element UI:
First, you'll need to install the necessary dependencies. Run the following command in your project directory:
```
npm install --save vue vue-class-component vue-property-decorator element-ui
```
Next, create a new TypeScript file for your login page component:
```typescript
import { Component, Vue } from 'vue-property-decorator';
import { Form, FormItem, Input, Button } from 'element-ui';
@Component({
components: {
[Form.name]: Form,
[FormItem.name]: FormItem,
[Input.name]: Input,
[Button.name]: Button,
},
})
export default class LoginPage extends Vue {
// data properties
private username: string = '';
private password: string = '';
// methods
private handleSubmit(): void {
// handle form submission here
}
// template
private render() {
return (
<div>
<h1>Login Page</h1>
<Form>
<FormItem label="Username">
<Input v-model={this.username}/>
</FormItem>
<FormItem label="Password">
<Input type="password" v-model={this.password}/>
</FormItem>
<Button type="primary" onClick={this.handleSubmit}>Login</Button>
</Form>
</div>
);
}
}
```
In this example, we're using the `@Component` decorator from the `vue-class-component` package to define our component. We've also imported the necessary Element UI components (`Form`, `FormItem`, `Input`, and `Button`) and registered them with the component using the `components` option.
Inside the component, we've defined two data properties (`username` and `password`) to store the user's input. We've also defined a `handleSubmit` method to handle the form submission.
Finally, in the `render` method, we're using JSX syntax to define the component's template. We're rendering a `Form` component with two `FormItem` components for the username and password inputs, and a `Button` component for the login button. We're using the `v-model` directive to bind the input values to the `username` and `password` data properties, and the `onClick` event to trigger the `handleSubmit` method when the login button is clicked.
That's it! You should now have a basic login page component with Vue.js, TypeScript, and Element UI. Of course, you'll need to add your own authentication logic to the `handleSubmit` method, but this should give you a good starting point.
vue3+ts+elementplus懒加载指令封装
对于Vue 3和TypeScript结合使用Element Plus进行懒加载指令的封装,可以按照以下步骤进行:
1. 创建一个新的文件,例如`lazyLoadDirective.ts`,在其中导入Vue、Directive和IntersectionObserver。
```typescript
import { App, Directive, DirectiveBinding } from 'vue';
import { DirectiveOptions } from 'vue';
// 导入IntersectionObserver
import IntersectionObserver from 'intersection-observer';
// 创建指令选项对象
const lazyLoadDirective: DirectiveOptions = {
// 指令生命周期钩子函数
mounted(el: HTMLElement, binding: DirectiveBinding) {
// 创建IntersectionObserver实例
const observer = new IntersectionObserver((entries) => {
// 当目标元素进入可视区域时加载内容
if (entries[0].isIntersecting) {
// 执行绑定的回调函数
binding.value();
// 关闭观察器
observer.disconnect();
}
});
// 开始观察目标元素
observer.observe(el);
}
};
// 导出指令对象
export default lazyLoadDirective;
```
2. 在`main.ts`文件中导入并注册该指令。
```typescript
import { createApp } from 'vue';
import App from './App.vue';
import lazyLoadDirective from './lazyLoadDirective.ts';
const app = createApp(App);
// 注册指令
app.directive('lazyload', lazyLoadDirective);
app.mount('#app');
```
3. 在组件中使用指令。
```html
<template>
<div v-lazyload="loadContent">Lazy Load Content</div>
</template>
<script>
export default {
methods: {
loadContent() {
// 加载内容的逻辑
}
}
}
</script>
```
以上是一种简单的方式来封装懒加载指令。请注意,此代码仅供参考,你可能需要根据具体的需求进行适当的修改和调整。
阅读全文
相关推荐
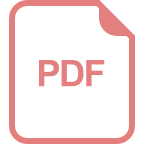
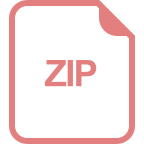
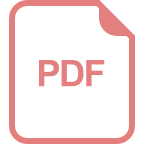
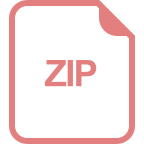
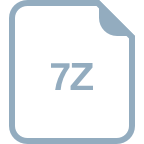
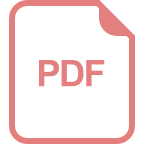
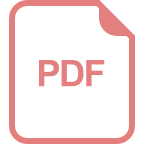
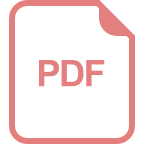
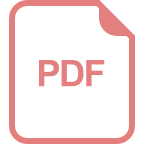
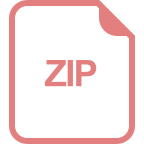





