假设把学生和监考教师都看作进程,学生有N人,教师1人。考场门口每次只能进出一个人,进考场的原则是先来先进。当N个学生都进入了考场后,教师才能发卷子。(N取10) 要求:在主线程中创建子线程分别模拟教师和学生的活动过程,实现两者的同步
时间: 2024-03-06 17:46:16 浏览: 23
可以使用互斥锁和条件变量来实现学生和教师的同步。
主线程中创建两个线程,一个线程模拟教师的活动过程,另一个线程模拟学生的活动过程。在学生进入考场时,需要获取互斥锁,判断当前考场人数是否已经达到N人,如果未达到,则释放互斥锁并等待条件变量。当考场人数达到N人时,教师线程会发卷子,此时会发送条件变量的信号,唤醒等待条件变量的学生线程继续执行。
下面是一个简单的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define N 10
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
int count = 0;
void *teacher_func(void *arg)
{
printf("Teacher is waiting for students\n");
pthread_mutex_lock(&mutex);
while (count < N)
{
pthread_cond_wait(&cond, &mutex);
}
printf("Teacher is giving papers\n");
pthread_mutex_unlock(&mutex);
return NULL;
}
void *student_func(void *arg)
{
int id = *(int *)arg;
printf("Student %d is waiting outside the exam room\n", id);
pthread_mutex_lock(&mutex);
while (count >= N)
{
pthread_cond_wait(&cond, &mutex);
}
printf("Student %d is entering the exam room\n", id);
count++;
if (count == N)
{
printf("All students have entered the exam room, teacher is giving papers\n");
pthread_cond_signal(&cond);
}
pthread_mutex_unlock(&mutex);
return NULL;
}
int main()
{
pthread_t teacher_tid, student_tid[N];
int i, id[N];
pthread_create(&teacher_tid, NULL, teacher_func, NULL);
for (i = 0; i < N; i++)
{
id[i] = i + 1;
pthread_create(&student_tid[i], NULL, student_func, &id[i]);
}
pthread_join(teacher_tid, NULL);
for (i = 0; i < N; i++)
{
pthread_join(student_tid[i], NULL);
}
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
在上面的示例代码中,我们使用了pthread_mutex_lock和pthread_mutex_unlock函数来获取和释放互斥锁,使用pthread_cond_wait和pthread_cond_signal函数来等待和发送条件变量的信号。在教师线程中,我们使用while循环来等待考场人数达到N人,同时在学生线程中,我们使用while循环来等待考场人数未达到N人。当考场人数达到N人时,教师线程会发送条件变量的信号,唤醒等待条件变量的学生线程继续执行。最后,我们使用pthread_join函数等待线程结束,使用pthread_mutex_destroy和pthread_cond_destroy函数销毁互斥锁和条件变量。
相关推荐
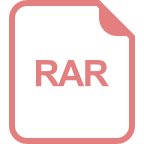
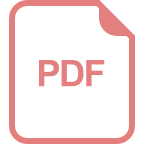














