java如何将有多张sheet页的excel文件转成一张竖向排列的图片
时间: 2024-02-11 10:05:32 浏览: 73
要将有多张sheet页的Excel文件转成一张竖向排列的图片,可以使用Java中的Apache POI和Apache PDFBox库。具体步骤如下:
1. 使用Apache POI读取Excel文件,获取每个sheet页的内容。
2. 将每个sheet页的内容绘制成图片,可以使用Java中的Graphics2D类或第三方库,如JFreeChart。
3. 将每个sheet页的图片合并成一张竖向排列的图片,可以使用Java中的BufferedImage类。
4. 将合并后的图片保存为PDF文件,可以使用Apache PDFBox库。
以下是示例代码:
```java
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageIO;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToPdfConverter {
public static void main(String[] args) throws Exception {
String excelFilePath = "path/to/excel/file.xlsx";
String pdfFilePath = "path/to/pdf/file.pdf";
int imageWidth = 600; // 图片宽度
int imageHeight = 800; // 图片高度
int marginTop = 20; // 图片上边距
int marginBottom = 20; // 图片下边距
int marginLeft = 20; // 图片左边距
int marginRight = 20; // 图片右边距
List<BufferedImage> images = new ArrayList<>();
Workbook workbook = new XSSFWorkbook(new FileInputStream(excelFilePath));
for (int i = 0; i < workbook.getNumberOfSheets(); i++) {
Sheet sheet = workbook.getSheetAt(i);
BufferedImage image = new BufferedImage(imageWidth, imageHeight, BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = image.createGraphics();
g2d.fillRect(0, 0, imageWidth, imageHeight);
g2d.translate(marginLeft, marginTop);
float scaleX = (float) (imageWidth - marginLeft - marginRight) / sheet.getPrintSetup().getPaperSize().getWidth();
float scaleY = (float) (imageHeight - marginTop - marginBottom) / sheet.getPrintSetup().getPaperSize().getHeight();
g2d.scale(scaleX, scaleY);
sheet.setZoom(1, 1);
sheet.setDisplayGridlines(false);
sheet.setPrintGridlines(false);
sheet.setFitToPage(true);
sheet.autoSizeColumn(0);
sheet.autoSizeRow(0);
sheet.getRow(0).setHeightInPoints(20);
for (Row row : sheet) {
for (Cell cell : row) {
g2d.drawString(cell.getStringCellValue(), (float) cell.getColumnIndex(), (float) row.getRowNum());
}
}
images.add(image);
}
workbook.close();
int totalHeight = images.stream().mapToInt(BufferedImage::getHeight).sum();
PDDocument document = new PDDocument();
PDPage page = new PDPage();
document.addPage(page);
float pageWidth = page.getMediaBox().getWidth();
float pageHeight = page.getMediaBox().getHeight();
float y = pageHeight - marginTop;
for (BufferedImage image : images) {
float height = (float) image.getHeight();
float width = (float) image.getWidth();
float x = (pageWidth - marginLeft - marginRight - width) / 2 + marginLeft;
y -= height;
if (y < marginBottom) {
page = new PDPage();
document.addPage(page);
y = pageHeight - marginTop - height;
}
PDImageXObject pdImage = LosslessFactory.createFromImage(document, image);
try (PDPageContentStream contents = new PDPageContentStream(document, page, AppendMode.APPEND, true)) {
contents.drawImage(pdImage, x, y, width, height);
}
y -= marginBottom;
}
document.save(new FileOutputStream(pdfFilePath));
document.close();
}
}
```
以上代码使用Apache POI读取Excel文件,将每个sheet页的内容绘制成图片,并使用Apache PDFBox将图片合并成一张PDF文件。其中,images列表存储每个sheet页的图片,totalHeight为所有图片的总高度,document为PDF文件对象,page为PDF文件的一页。在合并图片时,若当前页的剩余空间不足以放置当前图片,则新建一页。最终将PDF文件保存到指定路径。
阅读全文
相关推荐
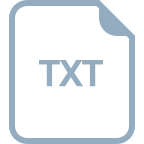
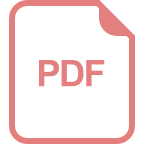
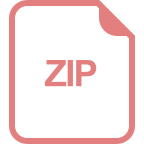
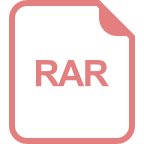
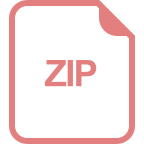
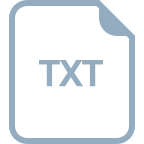
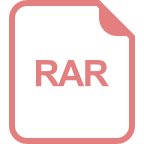
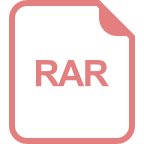
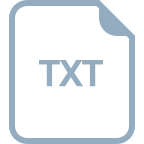
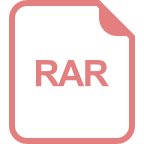
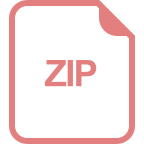
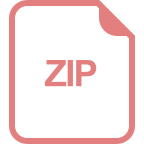
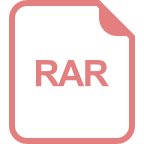