还需要再设计一张修改信息结果查询表吗
时间: 2024-01-02 09:04:23 浏览: 53
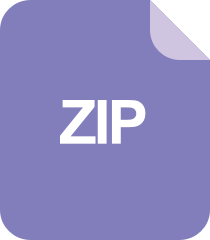
基于Java实现的班级信息管理系统.zip

如果需要对批量修改的结果进行查询和统计,可以考虑设计一张修改信息结果查询表,以下是一个基本的设计方案:
1. t_update_result 表
| 字段名 | 数据类型 | 说明 |
| ------ | ------ | ------ |
| id | int(11) | 主键 |
| cert_type | varchar(50) | 证件类型 |
| cert_no | varchar(50) | 证件号码 |
| cust_name_before | varchar(50) | 修改前客户名称 |
| cust_no_before | varchar(50) | 修改前客户编号 |
| cust_name_after | varchar(50) | 修改后客户名称 |
| cust_no_after | varchar(50) | 修改后客户编号 |
| update_time | datetime | 修改时间 |
| update_result | int(1) | 修改结果(0:失败,1:成功) |
2. t_update_result 表索引设计
| 索引名 | 索引类型 | 索引字段 | 说明 |
| ------ | ------ | ------ | ------ |
| PRIMARY | PRIMARY KEY | id | 主键索引 |
| idx_cert_type | INDEX | cert_type | 证件类型索引 |
| idx_cert_no | INDEX | cert_no | 证件号码索引 |
| idx_update_time | INDEX | update_time | 修改时间索引 |
3. Java批量修改程序设计
Java程序在对 t_cust_info 表进行批量修改后,可以根据修改结果更新 t_update_result 表。以下是一个基本的 Java 批量修改程序设计:
```java
public void batchUpdate(List<Map<String, Object>> dataList) {
Connection conn = null;
PreparedStatement pstmt1 = null;
PreparedStatement pstmt2 = null;
try {
conn = getConnection();
conn.setAutoCommit(false);
pstmt1 = conn.prepareStatement("UPDATE t_cust_info SET cust_name = ?, cust_no = ? WHERE cert_type = ? AND cert_no = ?");
pstmt2 = conn.prepareStatement("INSERT INTO t_update_result(cert_type, cert_no, cust_name_before, cust_no_before, cust_name_after, cust_no_after, update_time, update_result) VALUES (?, ?, ?, ?, ?, ?, ?, ?)");
for (Map<String, Object> data : dataList) {
String certType = (String) data.get("cert_type");
String certNo = (String) data.get("cert_no");
String custNameBefore = getCustName(certType, certNo);
String custNoBefore = getCustNo(certType, certNo);
String custNameAfter = (String) data.get("cust_name");
String custNoAfter = (String) data.get("cust_no");
pstmt1.setString(1, custNameAfter);
pstmt1.setString(2, custNoAfter);
pstmt1.setString(3, certType);
pstmt1.setString(4, certNo);
int result = pstmt1.executeUpdate();
pstmt2.setString(1, certType);
pstmt2.setString(2, certNo);
pstmt2.setString(3, custNameBefore);
pstmt2.setString(4, custNoBefore);
pstmt2.setString(5, custNameAfter);
pstmt2.setString(6, custNoAfter);
pstmt2.setTimestamp(7, new Timestamp(System.currentTimeMillis()));
pstmt2.setInt(8, result > 0 ? 1 : 0);
pstmt2.executeUpdate();
}
conn.commit();
} catch (SQLException e) {
if (conn != null) {
try {
conn.rollback();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
} finally {
if (pstmt1 != null) {
try {
pstmt1.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (pstmt2 != null) {
try {
pstmt2.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
以上是一个基本的修改信息结果查询表设计方案和 Java 批量修改程序设计,具体实现过程中还需要根据实际情况进行调整和优化。
阅读全文
相关推荐
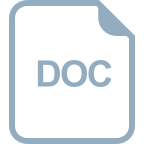
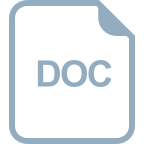
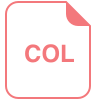









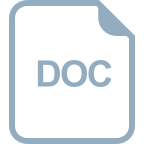
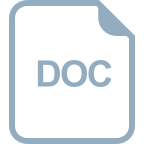
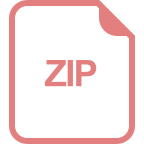
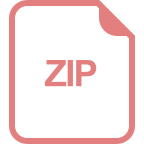