用python写一个定时器
时间: 2023-07-01 08:12:18 浏览: 135
你可以使用 Python 的 `time` 模块中的 `sleep` 函数来实现定时器。以下是一个示例代码,可以在终端或者命令行中运行:
```python
import time
# 设置定时器时间,单位为秒
timer_duration = 10
# 记录定时器开始时间
start_time = time.time()
while True:
# 获取当前时间
current_time = time.time()
# 计算时间差
time_difference = current_time - start_time
# 判断是否达到定时器时间
if time_difference >= timer_duration:
print("Time's up!")
break
# 输出剩余时间
print("Time remaining: ", int(timer_duration - time_difference))
# 等待一秒钟
time.sleep(1)
```
在上面的代码中,我们使用了一个 `while` 循环来不断计算当前时间和开始时间之间的时间差,然后判断是否达到了设定的定时器时间。如果达到了,就输出提醒信息并退出循环;否则,就输出剩余时间,并且等待一秒钟后再次循环。
相关问题
用python写一个定时器,定时器可以被控制
在Python中,我们可以使用`threading`模块结合`time`模块来创建一个简单的线程定时器,这样它可以被控制(如暂停、继续或停止)。以下是一个基本的例子:
```python
import threading
import time
class TimerThread(threading.Thread):
def __init__(self, interval, function, args=()):
threading.Thread.__init__(self)
self.interval = interval
self.function = function
self.args = args
self.paused = False
def run(self):
while not self.paused:
self.function(*self.args)
time.sleep(self.interval)
# 添加控制函数
def pause(self):
self.paused = True
def resume(self):
self.paused = False
if self.is_alive(): # 确保线程未终止再恢复
self.run()
# 使用示例
def print_message():
print("Timer triggered at", time.ctime())
timer = TimerThread(5, print_message) # 每隔5秒触发print_message函数
timer.start()
# 控制定时器
time.sleep(3) # 先运行一段时间
timer.pause() # 暂停
time.sleep(2) # 再次唤醒
timer.resume() # 继续执行
```
在这个例子中,`TimerThread`类有一个`pause`方法用于暂停定时器,`resume`方法用于恢复。你可以随时调用这两个方法来控制定时任务。
帮我用Python写一个定时器代码
很高兴为您服务!首先,您可以使用Python自带的time模块来编写定时器代码,例如:import time# 定义要延迟多长时间,以秒为单位
delay_time = 3# 记录定时器开始的时间
start_time = time.time()# 在此处添加你要执行的代码
print("This code is executed after 3 seconds")# 在此处添加延迟的代码
time.sleep(delay_time)# 计算出定时器结束的时间
end_time = time.time()# 计算出定时器实际运行的时间
run_time = end_time - start_time# 打印定时器实际运行的时间
print("The timer ran for {} seconds".format(run_time))
阅读全文
相关推荐











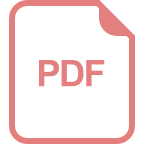
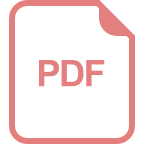