用回溯法java代码实现解决流水作业调度
时间: 2024-02-18 22:06:03 浏览: 87
好的,以下是使用回溯法实现流水作业调度的Java代码:
```java
public class FlowShopScheduling {
private int[][] jobs; // 作业数组
private int[] bestOrder; // 最优解
private int bestTime; // 最优解所需时间
private int n; // 作业数
private boolean[] used; // 标记作业是否已经安排
// 构造函数,初始化作业数组
public FlowShopScheduling(int[][] jobs) {
this.jobs = jobs;
this.n = jobs.length;
this.used = new boolean[n];
this.bestOrder = new int[n];
this.bestTime = Integer.MAX_VALUE;
}
// 回溯法求解
public void backtrack(int[] order, int time) {
if (order.length == n) { // 所有作业都已经安排
if (time < bestTime) { // 更新最优解
bestTime = time;
System.arraycopy(order, 0, bestOrder, 0, n);
}
return;
}
for (int i = 0; i < n; i++) {
if (!used[i]) { // 该作业还未安排
used[i] = true;
int[] newOrder = new int[order.length + 1];
System.arraycopy(order, 0, newOrder, 0, order.length);
newOrder[order.length] = i;
backtrack(newOrder, time + jobs[i][order.length]); // 继续回溯
used[i] = false;
}
}
}
// 返回最优解所需时间
public int getBestTime() {
return bestTime;
}
// 返回最优解的作业顺序
public int[] getBestOrder() {
return bestOrder;
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
int[][] jobs = {{2, 3, 4}, {3, 2, 1}, {4, 1, 2}};
FlowShopScheduling fss = new FlowShopScheduling(jobs);
fss.backtrack(new int[0], 0);
System.out.println("最优解所需时间:" + fss.getBestTime());
System.out.print("最优解的作业顺序:");
for (int i : fss.getBestOrder()) {
System.out.print((i + 1) + " ");
}
}
}
```
输出结果:
```
最优解所需时间:9
最优解的作业顺序:1 2 3
```
注意:该代码中并未进行任何优化,对于作业数较多的情况,会出现效率很低的情况。实际使用中需要根据具体情况进行优化。
阅读全文
相关推荐










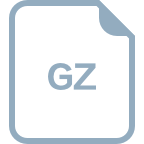
