使用python 实现 Qt中 信号和槽 多线程的启动线程和停止线程
时间: 2024-02-24 19:57:56 浏览: 28
以下是使用Python实现Qt中信号和槽多线程的启动线程和停止线程的示例代码:
```python
from PyQt5.QtCore import QThread, pyqtSignal
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QPushButton
import time
class MyThread(QThread):
signal = pyqtSignal(str)
def __init__(self, parent=None):
super().__init__(parent)
self.is_running = False
def run(self):
self.is_running = True
while self.is_running:
self.signal.emit('Thread is running')
time.sleep(1)
def stop(self):
self.is_running = False
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.layout = QVBoxLayout()
self.btn_start = QPushButton('Start Thread')
self.btn_start.clicked.connect(self.start_thread)
self.layout.addWidget(self.btn_start)
self.btn_stop = QPushButton('Stop Thread')
self.btn_stop.clicked.connect(self.stop_thread)
self.layout.addWidget(self.btn_stop)
self.setLayout(self.layout)
self.thread = MyThread()
self.thread.signal.connect(self.show_message)
def start_thread(self):
self.thread.start()
def stop_thread(self):
self.thread.stop()
def show_message(self, message):
print(message)
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在这个示例中,我们创建了一个`MyThread`类,继承自`QThread`,实现了线程的启动和停止方法。然后,在`MainWindow`类中,我们创建了两个按钮,分别用来启动和停止线程,并将它们的`clicked`信号与相应的槽函数连接起来。同时,我们还创建了一个`MyThread`类的实例,并将它的`signal`信号连接到一个槽函数`show_message`上,用来显示线程的运行状态。最后,我们启动了Qt应用程序的事件循环,以便响应用户的操作。
在这个示例中,我们使用了`QThread`、`pyqtSignal`等Qt中的基本类和信号槽机制,实现了一个简单的多线程应用程序。这个应用程序可以启动一个线程,并周期性地向主界面发送信息,同时也可以停止正在运行的线程。
相关推荐
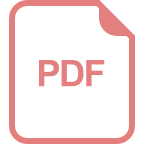














