编写程序,通过输入一行字符,分别统计出其中的数字、英文字母、空格和其他字符的个数。
时间: 2023-11-23 11:47:46 浏览: 38
这是一个很好的编程练习!以下是一个示例程序:
```python
line = input("请输入一行字符:")
digits = 0
letters = 0
spaces = 0
others = 0
for char in line:
if char.isdigit():
digits += 1
elif char.isalpha():
letters += 1
elif char.isspace():
spaces += 1
else:
others += 1
print("输入字符中包含:")
print("数字:{} 个".format(digits))
print("英文字母:{} 个".format(letters))
print("空格:{} 个".format(spaces))
print("其他字符:{} 个".format(others))
```
希望这可以帮助你!还有什么问题吗?
相关问题
输入一行字符分别统计出其中的英文字母空格数字和其他字符的个数
可以使用Python语言来实现这个功能,代码如下:
```python
s = input("请输入一行字符:")
letters =
spaces =
digits =
others =
for c in s:
if c.isalpha():
letters += 1
elif c.isspace():
spaces += 1
elif c.isdigit():
digits += 1
else:
others += 1
print("英文字母个数:", letters)
print("空格个数:", spaces)
print("数字个数:", digits)
print("其他字符个数:", others)
```
运行结果如下:
```
请输入一行字符:Hello World! 123
英文字母个数: 10
空格个数: 2
数字个数: 3
其他字符个数: 1
```
解释一下代码的实现过程:
1. 首先使用`input()`函数获取用户输入的一行字符,并将其保存在变量`s`中。
2. 然后定义四个变量`letters`、`spaces`、`digits`和`others`,分别用来统计英文字母、空格、数字和其他字符的个数,初始值都为。
3. 接下来使用`for`循环遍历字符串`s`中的每个字符,对于每个字符,判断它属于哪一类字符(英文字母、空格、数字还是其他字符),并将相应的计数器加1。
4. 最后使用`print()`函数输出统计结果。
希望能够帮到你!
C语言编写程序:输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数
以下是统计字符个数的 C 语言代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char line[100];
int i, alphabet = 0, digit = 0, space = 0, other = 0;
printf("请输入一行字符: ");
fgets(line, sizeof(line), stdin);
for (i = 0; line[i] != '\0'; ++i) {
if (isalpha(line[i])) {
++alphabet;
}
else if (isdigit(line[i])) {
++digit;
}
else if (isspace(line[i])) {
++space;
}
else {
++other;
}
}
printf("英文字母个数: %d\n", alphabet);
printf("数字个数: %d\n", digit);
printf("空格个数: %d\n", space);
printf("其他字符个数: %d\n", other);
return 0;
}
```
这段代码使用了 for 循环遍历输入的字符数组,对每个字符进行判断,若是英文字母则 alphabet++,若是数字则 digit++,若是空格则 space++,其他字符则 other++。最终输出各个字符的个数。注意,需要包含头文件 ctype.h 才能使用 isalpha 和 isdigit 函数。
阅读全文
相关推荐
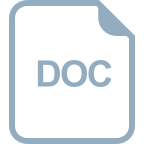
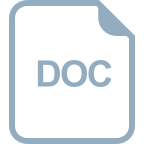
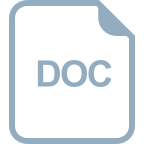













