利用蚁群算法求解最佳工艺路线请给代码实例
时间: 2023-11-13 09:04:19 浏览: 32
以下是一个简单的 Python 代码实例,用于演示如何使用蚁群算法求解最佳工艺路线问题:
```python
import random
# 蚂蚁数量
ANT_COUNT = 50
# 迭代次数
ITERATION = 100
# 信息素重要程度
ALPHA = 1
# 启发函数重要程度
BETA = 5
# 信息素挥发速度
RHO = 0.5
# 初始信息素浓度
INIT_PHEROMONE = 1.0
# 最大信息素浓度
MAX_PHEROMONE = 100.0
# 工艺路线图,这里用邻接矩阵表示
graph = [
[0, 2, 3, 1, 4],
[2, 0, 1, 2, 3],
[3, 1, 0, 4, 1],
[1, 2, 4, 0, 2],
[4, 3, 1, 2, 0]
]
# 初始化信息素浓度矩阵
pheromone = [[INIT_PHEROMONE] * len(graph) for _ in range(len(graph))]
# 计算启发函数值
def calculate_heuristic(from_node, to_node):
return 1.0 / graph[from_node][to_node]
# 根据概率选择下一步
def select_next_node(ant, visited):
current_node = ant[-1]
denominator = 0.0
for i in range(len(graph)):
if i not in visited:
denominator += pheromone[current_node][i] ** ALPHA * calculate_heuristic(current_node, i) ** BETA
probabilities = []
for i in range(len(graph)):
if i not in visited:
numerator = pheromone[current_node][i] ** ALPHA * calculate_heuristic(current_node, i) ** BETA
probabilities.append(numerator / denominator)
else:
probabilities.append(0.0)
next_node = probabilities.index(max(probabilities))
return next_node
# 更新信息素浓度
def update_pheromone():
for i in range(len(graph)):
for j in range(len(graph)):
pheromone[i][j] *= (1 - RHO)
pheromone[i][j] = max(pheromone[i][j], 0.0001)
# 蚁群算法主函数
def ant_colony_optimization():
global pheromone
best_path = []
best_distance = float('inf')
for _ in range(ITERATION):
ants = [[random.randint(0, len(graph) - 1)] for _ in range(ANT_COUNT)]
for i in range(len(graph) - 1):
for ant in ants:
visited = set(ant)
next_node = select_next_node(ant, visited)
ant.append(next_node)
distances = []
for ant in ants:
distance = sum([graph[ant[i]][ant[i+1]] for i in range(len(graph) - 1)])
distances.append(distance)
if distance < best_distance:
best_distance = distance
best_path = ant[:]
for i in range(len(graph) - 1):
for j in range(len(graph)):
delta_pheromone = 0.0
for ant in ants:
if (ant[i] == j and ant[i+1] == j):
delta_pheromone += 1.0 / graph[ant[i]][ant[i+1]]
pheromone[i][j] = (1 - RHO) * pheromone[i][j] + delta_pheromone
update_pheromone()
return best_path, best_distance
# 测试代码
best_path, best_distance = ant_colony_optimization()
print('最佳工艺路线为:', best_path)
print('最短距离为:', best_distance)
```
注:该代码仅为演示用途,可能存在不足之处,如有需要请自行修改和完善。
相关推荐
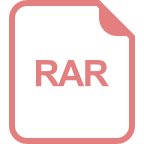














