python制作图形界面的画图代码
时间: 2023-12-15 22:46:50 浏览: 78
以下是一个简单的Python程序,使用tkinter库制作图形界面的画图:
```python
from tkinter import *
class PaintApp:
def __init__(self, master):
self.master = master
self.master.title("Paint App")
self.master.geometry("500x500")
self.master.config(bg="white")
self.brush_size = 2
self.brush_color = "black"
self.canvas = Canvas(self.master, width=500, height=500, bg="white")
self.canvas.pack()
self.canvas.bind("<B1-Motion>", self.draw)
self.controls()
def draw(self, event):
x1, y1 = (event.x - self.brush_size), (event.y - self.brush_size)
x2, y2 = (event.x + self.brush_size), (event.y + self.brush_size)
self.canvas.create_oval(x1, y1, x2, y2, fill=self.brush_color, outline=self.brush_color)
def change_brush_size(self, size):
self.brush_size = size
def change_brush_color(self, color):
self.brush_color = color
def controls(self):
brush_size_label = Label(self.master, text="Brush Size:", font=("Arial", 12), bg="white")
brush_size_label.place(x=10, y=10)
size_1_button = Button(self.master, text="1", width=3, command=lambda: self.change_brush_size(1))
size_1_button.place(x=100, y=10)
size_2_button = Button(self.master, text="2", width=3, command=lambda: self.change_brush_size(2))
size_2_button.place(x=130, y=10)
size_3_button = Button(self.master, text="3", width=3, command=lambda: self.change_brush_size(3))
size_3_button.place(x=160, y=10)
brush_color_label = Label(self.master, text="Brush Color:", font=("Arial", 12), bg="white")
brush_color_label.place(x=10, y=40)
color_red_button = Button(self.master, text="Red", width=5, bg="red", command=lambda: self.change_brush_color("red"))
color_red_button.place(x=100, y=40)
color_green_button = Button(self.master, text="Green", width=5, bg="green", command=lambda: self.change_brush_color("green"))
color_green_button.place(x=155, y=40)
color_blue_button = Button(self.master, text="Blue", width=5, bg="blue", command=lambda: self.change_brush_color("blue"))
color_blue_button.place(x=210, y=40)
root = Tk()
PaintApp(root)
root.mainloop()
```
该程序创建一个画布和一些控件,允许用户选择画笔大小和颜色,并在画布上绘制。控件使用Button和Label部件,可以根据需要进行扩展和更改。
阅读全文
相关推荐

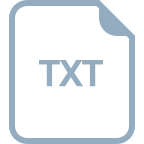
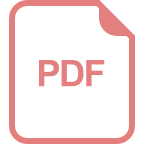
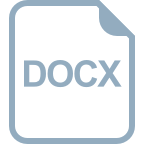
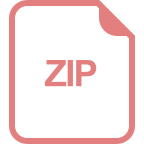
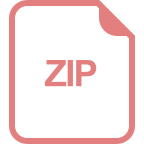
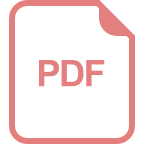
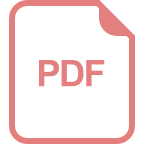
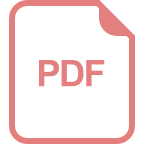
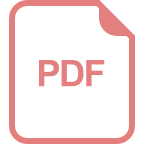
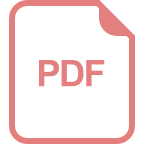
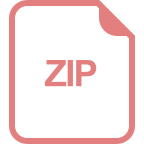
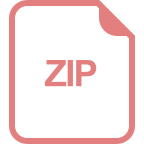
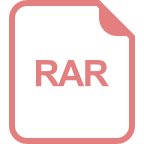
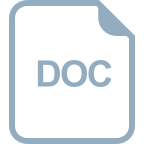
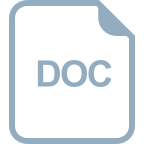