用C++写五子棋人机对决
时间: 2024-01-08 20:29:10 浏览: 127
写五子棋人机对决的C++代码需要一定的算法和逻辑。下面是一个简单的示例代码,展示了如何实现一个简单的五子棋人机对战:
```cpp
#include <iostream>
#include <vector>
using namespace std;
const int SIZE = 15;
const int WIN_COUNT = 5;
const char EMPTY = '.';
const char PLAYER = 'X';
const char AI = 'O';
// 棋盘
vector<vector<char>> board(SIZE, vector<char>(SIZE, EMPTY));
// 判断是否胜利
bool isWin(int row, int col, char player) {
// 判断横向
int count = 0;
for (int i = col - WIN_COUNT + 1; i <= col; i++) {
if (i < 0 || i >= SIZE) continue;
if (board[row][i] == player) {
count++;
if (count == WIN_COUNT) return true;
} else {
count = 0;
}
}
// 判断纵向
count = 0;
for (int i = row - WIN_COUNT + 1; i <= row; i++) {
if (i < 0 || i >= SIZE) continue;
if (board[i][col] == player) {
count++;
if (count == WIN_COUNT) return true;
} else {
count = 0;
}
}
// 判断左斜向
count = 0;
for (int i = -WIN_COUNT + 1; i <= 0; i++) {
int r = row + i;
int c = col + i;
if (r < 0 || r >= SIZE || c < 0 || c >= SIZE) continue;
if (board[r][c] == player) {
count++;
if (count == WIN_COUNT) return true;
} else {
count = 0;
}
}
// 判断右斜向
count = 0;
for (int i = -WIN_COUNT + 1; i <= 0; i++) {
int r = row + i;
int c = col - i;
if (r < 0 || r >= SIZE || c < 0 || c >= SIZE) continue;
if (board[r][c] == player) {
count++;
if (count == WIN_COUNT) return true;
} else {
count = 0;
}
}
return false;
}
// AI下棋
void aiMove() {
int maxScore = 0;
int bestRow = 0, bestCol = 0;
// 遍历每个空位
for (int row = 0; row < SIZE; row++) {
for (int col = 0; col < SIZE; col++) {
if (board[row][col] == EMPTY) {
int score = 0;
// 计算当前位置的得分
// 这里可以根据需要编写具体的评估函数
if (score > maxScore) {
maxScore = score;
bestRow = row;
bestCol = col;
}
}
}
}
// 在得分最高的位置下棋
board[bestRow][bestCol] = AI;
}
// 打印棋盘
void printBoard() {
for (int row = 0; row < SIZE; row++) {
for (int col = 0; col < SIZE; col++) {
cout << board[row][col] << " ";
}
cout << endl;
}
}
int main() {
int row, col;
while (true) {
cout << "请输入行号和列号(以空格分隔):";
cin >> row >> col;
// 判断输入是否合法
if (row < 0 || row >= SIZE || col < 0 || col >= SIZE || board[row][col] != EMPTY) {
cout << "输入无效,请重新输入!" << endl;
continue;
}
// 玩家下棋
board[row][col] = PLAYER;
printBoard();
// 判断是否胜利
if (isWin(row, col, PLAYER)) {
cout << "恭喜你,你赢了!" << endl;
break;
}
// AI下棋
aiMove();
printBoard();
// 判断是否胜利
if (isWin(row, col, AI)) {
cout << "很遗憾,你输了!" << endl;
break;
}
}
return 0;
}
```
这只是一个简单的示例,AI的下棋策略可以根据实际需求进行优化和改进。
相关推荐
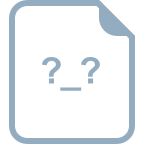













