python使用subprocess模块实现git更新分支代码到本地
时间: 2024-01-31 19:01:56 浏览: 151
可以使用subprocess模块调用命令行的git命令来更新分支代码到本地。以下是示例代码:
```python
import subprocess
# 切换到本地仓库目录
repo_path = "/path/to/your/repo"
os.chdir(repo_path)
# 执行git命令更新分支代码到本地
subprocess.call(["git", "pull", "origin", "your_branch_name"])
```
其中,`subprocess.call()`方法用于执行命令行命令,参数为一个列表,列表中的第一个元素为命令,后面的元素为命令的参数。在上面的示例中,`git pull origin your_branch_name`会从远程仓库拉取`your_branch_name`分支的最新代码到本地。
注意,执行该代码需要先安装并配置好Git环境。
相关问题
python使用subprocess模块实现git拉取分支代码到本地
可以使用 `subprocess` 模块来执行 `git` 命令,来拉取分支代码到本地。具体实现步骤如下:
1. 导入 subprocess 模块:
```python
import subprocess
```
2. 使用 `subprocess.run()` 方法来执行 git 命令,例如:
```python
subprocess.run(["git", "clone", "-b", "分支名", "远程仓库URL"])
```
其中,`git` 表示要执行的命令,`clone` 表示要进行的操作,`-b` 表示要拉取的分支名,`远程仓库URL` 表示要拉取的仓库的 URL。
如果要拉取的仓库已经存在本地,则可以使用 `git pull` 命令来更新代码,例如:
```python
subprocess.run(["git", "pull", "origin", "分支名"])
```
其中,`origin` 表示远程仓库的别名,`分支名` 表示要拉取的分支名。
完整的代码示例:
```python
import subprocess
# 拉取代码
subprocess.run(["git", "clone", "-b", "分支名", "远程仓库URL"])
# 更新代码
subprocess.run(["git", "pull", "origin", "分支名"])
```
注意,使用该方法需要保证系统上已经安装了 Git 工具。
python使用subprocess模块实现git pull更新分支代码到本地
可以使用Python的subprocess模块来执行Git命令。下面是一个示例代码,用于更新Git仓库中的分支代码到本地:
```python
import subprocess
# 设置Git仓库路径和分支名
repo_path = '/path/to/repo'
branch_name = 'main'
# 切换到Git仓库目录
os.chdir(repo_path)
# 执行Git命令
subprocess.run(['git', 'checkout', branch_name])
subprocess.run(['git', 'pull'])
```
在上面的代码中,首先设置Git仓库路径和分支名,然后使用Python的os模块切换到Git仓库目录。接下来,使用subprocess模块分别执行`git checkout`和`git pull`命令,以更新分支代码到本地。
阅读全文
相关推荐
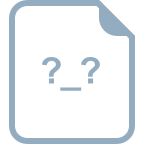
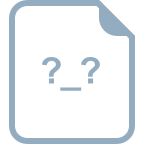
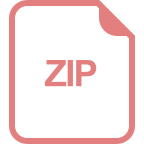




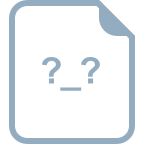
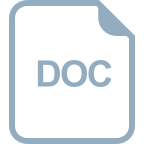
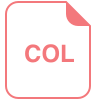


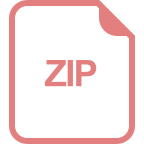
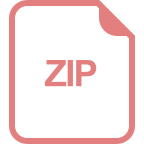
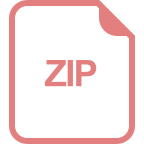
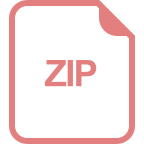