基于vgg的图像风格迁移代码
时间: 2023-05-11 17:00:53 浏览: 287
基于VGG的图像风格迁移代码涉及到深度学习中的卷积神经网络技术,可以用Python语言和算法库来实现。该代码主要由两部分组成,一部分是基于VGG卷积神经网络模型的图像内容和风格提取,另一部分是通过神经优化算法实现图像风格迁移。
首先,基于VGG卷积神经网络模型,可以通过代码将输入的图像转化为一系列的矩阵,然后利用这些矩阵提取图像的内容和风格。对于图像内容的提取,通常选择网络的一些深层特征,比如卷积层或池化层的输出,这些输出可以用于表示图像的内容。对于图像风格的提取,通常采用Gram Matrix方法,该方法通过将矩阵转化为向量并计算其内积来得到图像的风格特征。
其次,通过一系列的神经优化算法,可以实现基于VGG卷积神经网络模型的图像风格迁移。常用的神经优化算法有L-BFGS等,该算法通过对两个图像的内容和风格进行加权合成,从而实现图像风格的迁移。具体实现方法包括先通过VGG模型提取图像内容和风格的特征向量,然后将两个特征向量带入神经优化算法进行迭代处理,最终得到基于VGG的图像风格迁移效果。
总之,基于VGG的图像风格迁移代码在实现过程中需要结合卷积神经网络技术和神经优化算法,通过对图像内容和风格特征的提取和加权处理来实现图像风格迁移。这种方法能够在不同的图像任务中得到广泛应用,例如视觉效果的增强、图像生成和压缩等。
相关问题
vgg19图像风格迁移代码
VGG19图像风格迁移是一种通过深度学习实现图像风格转移的方法,其基于深度神经网络VGG19。其代码实现可以分为以下几步:
1. 导入必要的库:包括numpy、tensorflow等。
2. 加载预训练的VGG19模型:使用tensorflow的tf.keras.applications中的VGG19模型进行预训练。
3. 提取内容图片和风格图片的特征:使用预训练的VGG19模型提取内容图片和风格图片的特征。
4. 定义损失函数:将内容损失和风格损失加权求和作为总损失函数。
5. 使用优化器进行训练:通过梯度下降优化器来更新生成图片。
以下是简单的代码实现:
```
import numpy as np
import tensorflow as tf
from tensorflow.keras.applications import VGG19
from tensorflow.keras.preprocessing.image import load_img, img_to_array
# 加载VGG19模型,去掉最后一层输出
vgg = VGG19(include_top=False, weights='imagenet')
# 定义内容图片和风格图片的路径
content_path = 'content.jpg'
style_path = 'style.jpg'
# 加载图片并进行预处理
def load_and_process_img(path):
img = load_img(path)
img = img_to_array(img)
img = np.expand_dims(img, axis=0)
img = tf.keras.applications.vgg19.preprocess_input(img)
return img
# 提取内容图片和风格图片的特征
def get_feature_representations(model, content_path, style_path):
content_image = load_and_process_img(content_path)
style_image = load_and_process_img(style_path)
content_feature_maps = model.predict(content_image)
style_feature_maps = model.predict(style_image)
return content_feature_maps, style_feature_maps
# 计算Gram矩阵
def gram_matrix(input_tensor):
channels = int(input_tensor.shape[-1])
a = tf.reshape(input_tensor, [-1, channels])
n = tf.shape(a)
gram = tf.matmul(a, a, transpose_a=True)
return gram / tf.cast(n, tf.float32)
# 定义内容损失
def get_content_loss(base_content, target):
return tf.reduce_mean(tf.square(base_content - target))
# 定义风格损失
def get_style_loss(base_style, gram_target):
return tf.reduce_mean(tf.square(gram_matrix(base_style) - gram_target))
# 定义总损失函数
def compute_loss(model, loss_weights, init_image, gram_style_features, content_features):
style_weight, content_weight = loss_weights
model_outputs = model(init_image)
style_output_features = model_outputs[:len(gram_style_features)]
content_output_features = model_outputs[len(gram_style_features):]
style_score = 0
content_score = 0
weight_per_style_layer = 1.0 / float(len(style_output_features))
for target_style, comb_style in zip(gram_style_features, style_output_features):
style_score += weight_per_style_layer * get_style_loss(comb_style, target_style)
weight_per_content_layer = 1.0 / float(len(content_output_features))
for target_content, comb_content in zip(content_features, content_output_features):
content_score += weight_per_content_layer* get_content_loss(comb_content, target_content)
style_score *= style_weight
content_score *= content_weight
loss = style_score + content_score
return loss
# 定义优化器
def run_style_transfer(content_path, style_path,
num_iterations=1000,
content_weight=1e3,
style_weight=1e-2):
# 提取内容图片和风格图片的特征
content_features, style_features = get_feature_representations(vgg, content_path, style_path)
gram_style_features = [gram_matrix(feature) for feature in style_features]
init_image = load_and_process_img(content_path)
init_image = tf.Variable(init_image, dtype=tf.float32)
opt = tf.optimizers.Adam(learning_rate=5, beta_1=0.99, epsilon=1e-1)
# 定义损失权重
loss_weights = (style_weight, content_weight)
# 进行训练
for i in range(num_iterations):
with tf.GradientTape() as tape:
loss = compute_loss(vgg, loss_weights, init_image, gram_style_features, content_features)
grad = tape.gradient(loss, init_image)
opt.apply_gradients([(grad, init_image)])
clipped = tf.clip_by_value(init_image, clip_value_min=0.0, clip_value_max=255.0)
init_image.assign(clipped)
return init_image.numpy()
```
vgg19风格迁移代码
### 回答1:
VGG19风格迁移代码是一种用于将图像的内容与风格进行分离,并将两者合成以创建新图像的算法。该算法基于深度卷积神经网络VGG19,它是一种经典的视觉感知模型,用于图像分类和识别任务。
在实现VGG19风格迁移代码时,我们需要进行以下步骤:
1. 导入相关的Python库和模块,例如tensorflow、opencv和numpy等。
2. 加载VGG19模型的权重文件,以便使用VGG19进行图像特征提取。
3. 定义图片的内容损失函数,该函数用于衡量生成图像与原始图像之间的内容相似度。
4. 定义图片的风格损失函数,该函数用于衡量生成图像与目标风格图像之间的风格相似度。
5. 定义总体损失函数,该函数将内容损失和风格损失加权组合在一起,以平衡两者的影响。
6. 使用优化算法,如梯度下降法,来最小化总体损失函数,从而更新生成图像的像素值。
7. 重复步骤6,直到生成的图像与原始图像在内容和风格上都达到满意的程度。
需要注意的是,VGG19风格迁移代码是一种较为复杂和计算密集的算法,可能需要较长的训练时间和高性能的计算设备。因此,在实际应用中,可以使用预训练的VGG19模型,以加快风格迁移的速度。
这就是VGG19风格迁移代码的一般步骤和流程。通过这种方法,我们可以将不同图像的内容与风格进行有机地融合,从而创造出独特且具有艺术感的图像。
### 回答2:
VGG19是一种深度卷积神经网络模型,经常被用于图像分类任务。而风格迁移是一种计算机视觉的技术,它可以将一幅图像的风格迁移到另一幅图像上,从而创造出具有新风格的图像。
VGG19风格迁移代码实现的基本原理如下:
1. 导入VGG19模型的权重参数,这些参数在预训练模型中已经通过大规模训练集进行了优化,可以提取出图像中的不同特征。
2. 加载待进行风格迁移的两个图像,一个是内容图像,一个是风格图像,通过读取图像的像素值进行处理。
3. 对内容图像和风格图像分别进行预处理,将图像缩放至合适的大小,并通过减去均值来进行归一化。
4. 将内容图像和风格图像输入到VGG19网络中,分别提取出内容特征和风格特征,这些特征通过网络的不同层来表示不同等级和抽象程度的特征信息。
5. 使用内容图像的特征与风格图像的特征计算损失函数,通过最小化这个损失函数来求解风格迁移的目标图像。
6. 通过梯度下降等优化算法,对目标图像进行迭代优化,不断更新图像的像素值,使得目标图像的内容与内容图像的特征相似,同时与风格图像的特征相匹配。
7. 最后得到的目标图像即为风格迁移后的图像。
这是简单概括了VGG19风格迁移代码的运行过程。实际使用时,还需要在代码中设置合适的超参数、学习率,以及选择不同层的特征来表示风格等。这是一个复杂的计算过程,需要一定的计算资源和训练时间。
阅读全文
相关推荐
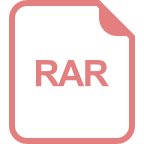
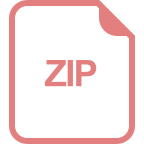
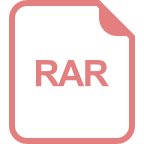
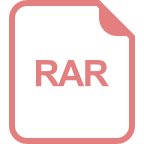
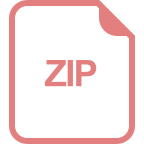
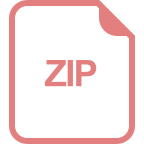
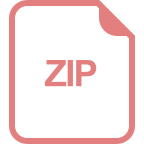
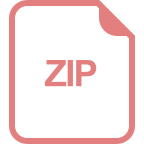
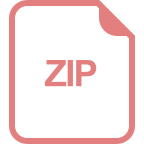
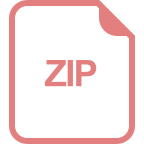
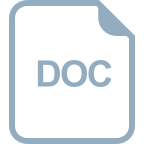
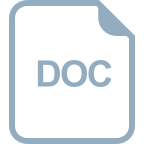
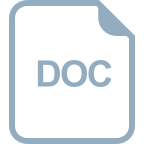
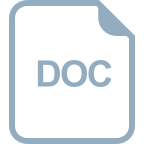

