MSP430F5529实现计算器
时间: 2023-08-02 08:43:09 浏览: 86
MSP430F5529是一款微控制器,你可以通过编程实现计算器的功能。下面是一个简单的计算器实现的示例代码:
```C
#include <msp430.h>
int main(void)
{
WDTCTL = WDTPW | WDTHOLD; // stop watchdog timer
PM5CTL0 &= ~LOCKLPM5; // disable the GPIO power-on default high-impedance mode
P1DIR |= BIT0; // set P1.0 as output
P1OUT &= ~BIT0; // set P1.0 to low initially
char num1[10], num2[10], op;
int result;
while(1)
{
// read in the first number
int i = 0;
do
{
while((UCA0IFG & UCTXIFG) == 0); // wait for TX buffer to be empty
UCA0TXBUF = getchar(); // send prompt to serial console
num1[i] = getchar(); // read in character from serial console
i++;
} while(num1[i-1] != '\n'); // continue reading until newline character is received
num1[i-1] = '\0'; // replace newline character with null terminator
// read in the operator
while((UCA0IFG & UCTXIFG) == 0); // wait for TX buffer to be empty
UCA0TXBUF = getchar(); // send prompt to serial console
op = getchar(); // read in operator character from serial console
// read in the second number
int j = 0;
do
{
while((UCA0IFG & UCTXIFG) == 0); // wait for TX buffer to be empty
UCA0TXBUF = getchar(); // send prompt to serial console
num2[j] = getchar(); // read in character from serial console
j++;
} while(num2[j-1] != '\n'); // continue reading until newline character is received
num2[j-1] = '\0'; // replace newline character with null terminator
// perform calculation based on operator
switch(op)
{
case '+':
result = atoi(num1) + atoi(num2);
break;
case '-':
result = atoi(num1) - atoi(num2);
break;
case '*':
result = atoi(num1) * atoi(num2);
break;
case '/':
result = atoi(num1) / atoi(num2);
break;
default:
result = 0;
break;
}
// output result to serial console and LED
printf("Result: %d\n", result);
if(result > 0)
P1OUT |= BIT0; // turn on LED if result is positive
else
P1OUT &= ~BIT0; // turn off LED if result is negative or zero
}
}
```
这段代码通过串口与计算机进行交互,读取两个数字和一个运算符,并根据运算符进行计算。运算结果通过串口返回到计算机,并且通过一个LED灯显示在开发板上。你可以根据自己的需求对代码进行修改和优化。
阅读全文
相关推荐


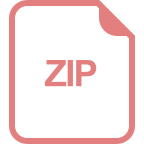
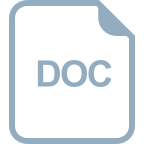
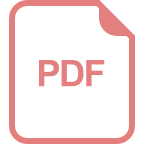
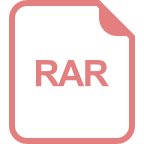
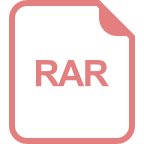
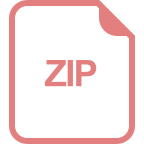
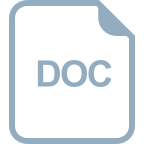
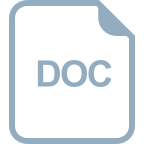
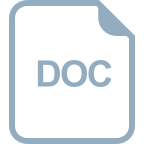
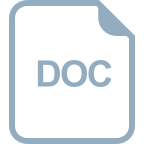
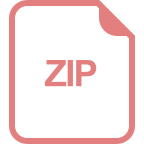
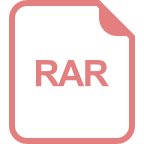