pymysql如何使用
时间: 2023-10-24 08:05:50 浏览: 129
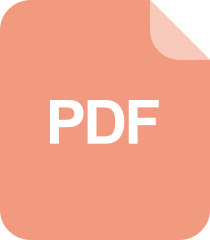
python中的pymysql模块基本使用

Pymysql可以用来连接MySQL数据库,首先需要安装pymysql模块,然后使用以下代码连接数据库:
```python
import pymysql
# 打开数据库连接
db = pymysql.connect(host="localhost", user="root",
password="password", database="test")
# 使用cursor()方法获取操作游标
cursor = db.cursor()
# SQL 查询语句
sql = "SELECT * FROM users"
# 执行SQL语句
cursor.execute(sql)
# 获取所有记录列表
results = cursor.fetchall()
# 遍历结果
for row in results:
print(row)
# 关闭数据库连接
db.close()
```
以上代码可以查询名为“users”的表中的所有记录并将其打印出来。如果想执行其他的SQL语句,只需要修改相应的sql变量即可。
阅读全文
相关推荐






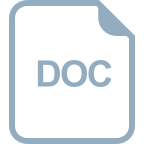









