不基于opencv库,使用soble算子,在二值化图像中检测出来黑色边框矩形,提供代码
时间: 2023-06-03 17:06:37 浏览: 73
以下是使用sobel算子在二值化图像中检测黑色边框矩形的示例代码:
```python
import cv2
import numpy as np
# 读取二值化图像
img = cv2.imread('binary_image.png', 0)
# 对图像进行膨胀操作,填充图像内部的小洞
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
img = cv2.dilate(img, kernel, iterations=1)
# 使用sobel算子对图像进行边缘检测
sobelx = cv2.Sobel(img, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(img, cv2.CV_64F, 0, 1, ksize=3)
abs_sobelx = np.absolute(sobelx)
abs_sobely = np.absolute(sobely)
sobel = cv2.convertScaleAbs(abs_sobelx + abs_sobely)
# 对边缘图像进行阈值处理,得到二值化边缘图像
sobel = cv2.medianBlur(sobel, 5)
ret, thresh = cv2.threshold(sobel, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
# 在二值化边缘图像中寻找轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓,寻找黑色矩形
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if img[y, x] == 0 and img[y + h, x + w] == 0:
# 绘制结果矩形
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 0, 255), 2)
# 显示结果
cv2.imshow('result', img)
cv2.waitKey()
cv2.destroyAllWindows()
```
其中,输入的二值化图像需保存为名为"binary_image.png"的文件。代码中的思路为:首先对图像进行膨胀操作,填充图像内部的小洞;然后使用sobel算子对图像进行边缘检测,并对边缘图像进行阈值处理得到二值化的边缘图像;最后寻找轮廓,并判断轮廓是否属于黑色矩形。
相关推荐
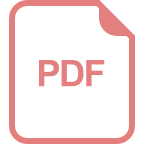
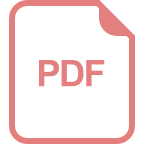
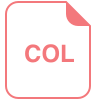













