利用python实现通过卷积神经网络的图像压缩算法实现,目标是输入一张图片,对图片进行压缩,然后再将图片输出
时间: 2023-07-16 11:12:12 浏览: 80
首先,我们需要安装必要的库:Tensorflow、Keras、NumPy、Matplotlib。可以使用以下命令安装:
```
pip install tensorflow keras numpy matplotlib
```
接下来,我们需要加载并预处理图像。这里我们将使用Pillow库来加载图像,然后使用NumPy库来将图像转换为张量(tensor),以便在神经网络中使用。
```python
from PIL import Image
import numpy as np
def load_image(path):
image = Image.open(path)
image = image.convert('RGB')
image = image.resize((224, 224)) # 将图像缩放为 224x224
image_array = np.array(image)
image_array = np.expand_dims(image_array, axis=0) # 将图像转换为张量
return image_array
```
接下来,我们需要构建卷积神经网络。这里我们将使用Keras库来构建模型。我们将使用一个简单的卷积神经网络,该网络包含一个卷积层、一个最大池化层和一个反卷积层。
```python
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, UpSampling2D
def build_model(input_shape):
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', padding='same', input_shape=input_shape))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(128, (3, 3), activation='relu', padding='same'))
model.add(UpSampling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu', padding='same'))
model.add(UpSampling2D((2, 2)))
model.add(Conv2D(32, (3, 3), activation='relu', padding='same'))
model.add(Conv2D(3, (3, 3), activation='sigmoid', padding='same'))
return model
```
现在我们可以加载图像并将其输入到模型中进行压缩。
```python
image = load_image('image.jpg')
model = build_model(image.shape[1:])
compressed_image = model.predict(image)
```
最后,我们可以将压缩后的图像保存到文件中。
```python
from PIL import Image
def save_image(image_array, path):
image_array = image_array * 255 # 将像素值缩放到 0-255 的范围
image_array = np.clip(image_array, 0, 255).astype('uint8') # 将像素值截断到 0-255 的范围,并将数据类型转换为整数
image_array = np.squeeze(image_array, axis=0) # 去掉批次维度
image = Image.fromarray(image_array)
image.save(path)
save_image(compressed_image, 'compressed_image.jpg')
```
这样,我们就完成了通过卷积神经网络的图像压缩算法的实现。
阅读全文
相关推荐
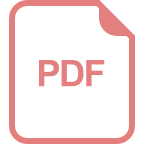
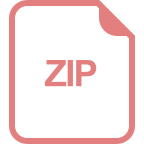
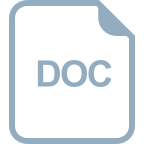
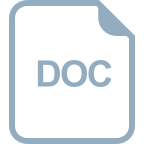
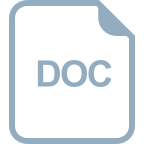
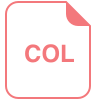
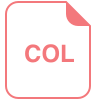
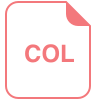
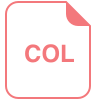
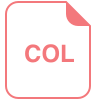

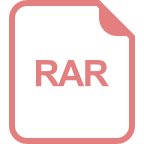
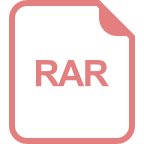
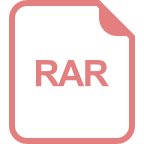
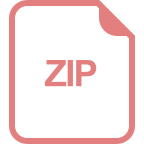
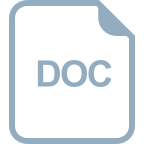
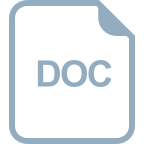
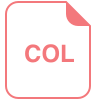