根据name字段去user表中查询age字段的值 mybatisplus
时间: 2023-08-07 19:03:26 浏览: 135
在Mybatis Plus中,可以使用如下的代码来实现:
```java
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("name", "要查询的用户名");
User user = userMapper.selectOne(queryWrapper);
int age = user.getAge();
```
其中,`User`为对应的实体类,`userMapper`为对应的Mapper接口,`name`和`age`为实体类中对应的字段名,`要查询的用户名`为具体需要查询的用户名。通过`QueryWrapper`对象构建查询条件,然后调用`selectOne`方法查询到对应的用户实体。最后通过实体对象的`getAge`方法获取到该用户对应的年龄值。
相关问题
mybatisplus LambdaQueryWrapper 查询mysql指定字段 比如User表里的 string name,以及int age字段
好的,使用MyBatis Plus的LambdaQueryWrapper查询指定字段可以通过以下代码实现:
```java
LambdaQueryWrapper<User> wrapper = new LambdaQueryWrapper<User>()
.select(User::getName, User::getAge) // 指定要查询的字段
.eq(User::getName, "张三"); // 添加条件,这里以姓名为例
List<User> userList = userMapper.selectList(wrapper); // 执行查询
```
上述代码中,`select` 方法用于指定要查询的字段,可以通过实体类的属性引用来指定,这样可以避免手写 SQL 时出现拼写错误等问题。
在添加查询条件时,可以使用类似 `eq`、`like`、`gt` 等方法来指定查询条件,这些方法的参数也可以使用实体类的属性引用来指定。最后,通过调用 `selectList` 方法来执行查询,并返回满足条件的结果集。
如果您还有其他问题,请继续提出,我会尽力为您解答。
mybatisplus批量更新list<user>的name和age字段的方法
您可以使用 MybatisPlus 提供的 `updateBatchById` 方法来批量更新 `List<User>` 对象的 `name` 和 `age` 字段,具体操作如下:
```java
List<User> userList = new ArrayList<>();
// 假设这里已经将 userList 填充好了
// 批量更新的字段值
User updateUser = new User();
updateUser.setName("newName");
updateUser.setAge(18);
// 构造更新条件
List<Long> userIdList = userList.stream().map(User::getId).collect(Collectors.toList());
UpdateWrapper<User> updateWrapper = new UpdateWrapper<>();
updateWrapper.in("id", userIdList);
// 执行批量更新操作
int result = userService.update(updateUser, updateWrapper);
```
需要注意的是,`updateBatchById` 方法只能更新指定 ID 的记录,而不能通过其他条件进行筛选。如果需要根据其他条件进行批量更新,可以使用 `UpdateWrapper` 对象进行条件构造,然后使用 `update` 方法进行更新。
阅读全文
相关推荐
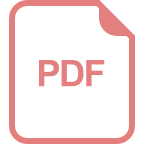














