企业微信机器人php
时间: 2023-07-26 16:17:01 浏览: 61
您好,关于企业微信机器人的开发,可以使用PHP开发语言进行实现。企业微信机器人可以实现自动回复、事件监听等功能,具体实现方式可以参考企业微信开发文档。
在PHP中,可以使用企业微信提供的API接口进行开发。首先需要在企业微信后台创建一个应用,并获取到应用的ID和Secret。然后可以使用企业微信提供的API接口进行消息发送、用户管理等操作。
以下是一个简单的PHP企业微信机器人代码示例:
```php
<?php
// 设置企业微信应用ID和Secret
$appId = 'your_app_id';
$appSecret = 'your_app_secret';
// 获取access_token
$accessToken = getAccessToken($appId, $appSecret);
// 处理接收到的消息
$postData = file_get_contents('php://input');
$msgData = json_decode($postData, true);
if (!empty($msgData)) {
$msgType = $msgData['MsgType'];
switch ($msgType) {
case 'text':
// 处理文本消息
$content = $msgData['Content'];
$replyContent = '您发送的消息是:' . $content;
sendTextMessage($msgData['FromUserName'], $replyContent, $accessToken);
break;
case 'event':
// 处理事件消息
$eventType = $msgData['Event'];
if ($eventType == 'subscribe') {
// 处理关注事件
$replyContent = '欢迎关注!';
sendTextMessage($msgData['FromUserName'], $replyContent, $accessToken);
}
break;
}
}
// 获取access_token
function getAccessToken($appId, $appSecret) {
$url = "https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid=$appId&corpsecret=$appSecret";
$response = file_get_contents($url);
$result = json_decode($response, true);
return $result['access_token'];
}
// 发送文本消息
function sendTextMessage($userId, $content, $accessToken) {
$url = "https://qyapi.weixin.qq.com/cgi-bin/message/send?access_token=$accessToken";
$data = array(
'touser' => $userId,
'msgtype' => 'text',
'text' => array('content' => $content),
);
$postData = json_encode($data);
$options = array(
'http' => array(
'method' => 'POST',
'header' => 'Content-type:application/json',
'content' => $postData,
'timeout' => 15 * 60,
),
);
$context = stream_context_create($options);
$response = file_get_contents($url, false, $context);
return $response;
}
```
以上代码实现了一个简单的企业微信机器人,可以通过收到的文本消息进行自动回复,也可以处理关注事件等。
相关推荐
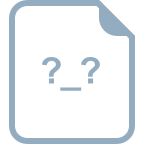
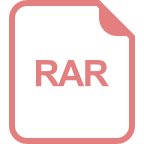














