超市食品信息管理系统运用SQL 作为后台数据库,运用c++作为前台数据的c++代码
时间: 2023-12-08 07:03:24 浏览: 130
以下是一个简单的超市食品信息管理系统的 C++ 代码,使用 SQL 作为后台数据库:
```c++
#include <iostream>
#include <windows.h>
#include <sqlext.h>
#include <sqltypes.h>
#include <sql.h>
using namespace std;
void showMenu();
void addProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle);
void searchProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle);
void updateProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle);
void deleteProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle);
void showAllProducts(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle);
int main()
{
// Initialize variables
SQLHANDLE sqlEnvHandle;
SQLHANDLE sqlConnHandle;
SQLHANDLE sqlStmtHandle;
SQLRETURN retcode;
// Allocate an environment handle
retcode = SQLAllocHandle(SQL_HANDLE_ENV, SQL_NULL_HANDLE, &sqlEnvHandle);
// Set the ODBC version environment attribute
retcode = SQLSetEnvAttr(sqlEnvHandle, SQL_ATTR_ODBC_VERSION, (SQLPOINTER)SQL_OV_ODBC3, 0);
// Allocate a connection handle
retcode = SQLAllocHandle(SQL_HANDLE_DBC, sqlEnvHandle, &sqlConnHandle);
// Connect to the database using DSN
retcode = SQLConnect(sqlConnHandle, (SQLCHAR*)"DSN", SQL_NTS, NULL, 0, NULL, 0);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO)
{
cout << "Connected to database." << endl;
// Allocate a statement handle
retcode = SQLAllocHandle(SQL_HANDLE_STMT, sqlConnHandle, &sqlStmtHandle);
// Show the main menu
showMenu();
// Free the statement handle
SQLFreeHandle(SQL_HANDLE_STMT, sqlStmtHandle);
// Disconnect from the database
SQLDisconnect(sqlConnHandle);
}
else
{
cout << "Failed to connect to database." << endl;
}
// Free the connection handle
SQLFreeHandle(SQL_HANDLE_DBC, sqlConnHandle);
// Free the environment handle
SQLFreeHandle(SQL_HANDLE_ENV, sqlEnvHandle);
return 0;
}
void showMenu()
{
SQLHANDLE sqlConnHandle;
SQLHANDLE sqlStmtHandle;
SQLRETURN retcode;
// Allocate an environment handle
retcode = SQLAllocHandle(SQL_HANDLE_ENV, SQL_NULL_HANDLE, &sqlConnHandle);
// Set the ODBC version environment attribute
retcode = SQLSetEnvAttr(sqlConnHandle, SQL_ATTR_ODBC_VERSION, (SQLPOINTER)SQL_OV_ODBC3, 0);
// Allocate a connection handle
retcode = SQLAllocHandle(SQL_HANDLE_DBC, sqlConnHandle, &sqlConnHandle);
// Connect to the database using DSN
retcode = SQLConnect(sqlConnHandle, (SQLCHAR*)"DSN", SQL_NTS, NULL, 0, NULL, 0);
int choice;
do
{
cout << endl;
cout << "1. Add a product" << endl;
cout << "2. Search for a product" << endl;
cout << "3. Update a product" << endl;
cout << "4. Delete a product" << endl;
cout << "5. Show all products" << endl;
cout << "6. Exit" << endl;
cout << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice)
{
case 1:
addProduct(sqlConnHandle, sqlStmtHandle);
break;
case 2:
searchProduct(sqlConnHandle, sqlStmtHandle);
break;
case 3:
updateProduct(sqlConnHandle, sqlStmtHandle);
break;
case 4:
deleteProduct(sqlConnHandle, sqlStmtHandle);
break;
case 5:
showAllProducts(sqlConnHandle, sqlStmtHandle);
break;
case 6:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
break;
}
} while (choice != 6);
// Disconnect from the database
SQLDisconnect(sqlConnHandle);
// Free the connection handle
SQLFreeHandle(SQL_HANDLE_DBC, sqlConnHandle);
// Free the environment handle
SQLFreeHandle(SQL_HANDLE_ENV, sqlConnHandle);
}
void addProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle)
{
SQLRETURN retcode;
char name[50];
float price;
int quantity;
cout << endl;
cout << "Enter product name: ";
cin.ignore();
cin.getline(name, 50);
cout << "Enter product price: ";
cin >> price;
cout << "Enter product quantity: ";
cin >> quantity;
// Prepare the SQL statement
retcode = SQLPrepare(sqlStmtHandle, (SQLCHAR*)"INSERT INTO products (name, price, quantity) VALUES (?, ?, ?)", SQL_NTS);
// Bind parameters
SQLBindParameter(sqlStmtHandle, 1, SQL_PARAM_INPUT, SQL_C_CHAR, SQL_VARCHAR, 50, 0, name, 0, NULL);
SQLBindParameter(sqlStmtHandle, 2, SQL_PARAM_INPUT, SQL_C_FLOAT, SQL_REAL, 0, 0, &price, 0, NULL);
SQLBindParameter(sqlStmtHandle, 3, SQL_PARAM_INPUT, SQL_C_LONG, SQL_INTEGER, 0, 0, &quantity, 0, NULL);
// Execute the SQL statement
retcode = SQLExecute(sqlStmtHandle);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO)
{
cout << "Product added successfully." << endl;
}
else
{
cout << "Failed to add product." << endl;
}
}
void searchProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle)
{
SQLRETURN retcode;
char name[50];
cout << endl;
cout << "Enter product name: ";
cin.ignore();
cin.getline(name, 50);
// Prepare the SQL statement
retcode = SQLPrepare(sqlStmtHandle, (SQLCHAR*)"SELECT * FROM products WHERE name = ?", SQL_NTS);
// Bind parameter
SQLBindParameter(sqlStmtHandle, 1, SQL_PARAM_INPUT, SQL_C_CHAR, SQL_VARCHAR, 50, 0, name, 0, NULL);
// Execute the SQL statement
retcode = SQLExecute(sqlStmtHandle);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO)
{
// Fetch the data
SQLCHAR name[50];
float price;
int quantity;
SQLBindCol(sqlStmtHandle, 1, SQL_C_CHAR, name, 50, NULL);
SQLBindCol(sqlStmtHandle, 2, SQL_C_FLOAT, &price, 0, NULL);
SQLBindCol(sqlStmtHandle, 3, SQL_C_LONG, &quantity, 0, NULL);
while (SQLFetch(sqlStmtHandle) == SQL_SUCCESS)
{
cout << "Product name: " << name << endl;
cout << "Product price: " << price << endl;
cout << "Product quantity: " << quantity << endl;
cout << endl;
}
}
else
{
cout << "Failed to search for product." << endl;
}
}
void updateProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle)
{
SQLRETURN retcode;
char name[50];
float price;
int quantity;
cout << endl;
cout << "Enter product name: ";
cin.ignore();
cin.getline(name, 50);
cout << "Enter new product price: ";
cin >> price;
cout << "Enter new product quantity: ";
cin >> quantity;
// Prepare the SQL statement
retcode = SQLPrepare(sqlStmtHandle, (SQLCHAR*)"UPDATE products SET price = ?, quantity = ? WHERE name = ?", SQL_NTS);
// Bind parameters
SQLBindParameter(sqlStmtHandle, 1, SQL_PARAM_INPUT, SQL_C_FLOAT, SQL_REAL, 0, 0, &price, 0, NULL);
SQLBindParameter(sqlStmtHandle, 2, SQL_PARAM_INPUT, SQL_C_LONG, SQL_INTEGER, 0, 0, &quantity, 0, NULL);
SQLBindParameter(sqlStmtHandle, 3, SQL_PARAM_INPUT, SQL_C_CHAR, SQL_VARCHAR, 50, 0, name, 0, NULL);
// Execute the SQL statement
retcode = SQLExecute(sqlStmtHandle);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO)
{
cout << "Product updated successfully." << endl;
}
else
{
cout << "Failed to update product." << endl;
}
}
void deleteProduct(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle)
{
SQLRETURN retcode;
char name[50];
cout << endl;
cout << "Enter product name: ";
cin.ignore();
cin.getline(name, 50);
// Prepare the SQL statement
retcode = SQLPrepare(sqlStmtHandle, (SQLCHAR*)"DELETE FROM products WHERE name = ?", SQL_NTS);
// Bind parameter
SQLBindParameter(sqlStmtHandle, 1, SQL_PARAM_INPUT, SQL_C_CHAR, SQL_VARCHAR, 50, 0, name, 0, NULL);
// Execute the SQL statement
retcode = SQLExecute(sqlStmtHandle);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO)
{
cout << "Product deleted successfully." << endl;
}
else
{
cout << "Failed to delete product." << endl;
}
}
void showAllProducts(SQLHANDLE& sqlConnHandle, SQLHANDLE& sqlStmtHandle)
{
SQLRETURN retcode;
// Prepare the SQL statement
retcode = SQLPrepare(sqlStmtHandle, (SQLCHAR*)"SELECT * FROM products", SQL_NTS);
// Execute the SQL statement
retcode = SQLExecute(sqlStmtHandle);
if (retcode == SQL_SUCCESS || retcode == SQL_SUCCESS_WITH_INFO)
{
// Fetch the data
SQLCHAR name[50];
float price;
int quantity;
SQLBindCol(sqlStmtHandle, 1, SQL_C_CHAR, name, 50, NULL);
SQLBindCol(sqlStmtHandle, 2, SQL_C_FLOAT, &price, 0, NULL);
SQLBindCol(sqlStmtHandle, 3, SQL_C_LONG, &quantity, 0, NULL);
while (SQLFetch(sqlStmtHandle) == SQL_SUCCESS)
{
cout << "Product name: " << name << endl;
cout << "Product price: " << price << endl;
cout << "Product quantity: " << quantity << endl;
cout << endl;
}
}
else
{
cout << "Failed to show all products." << endl;
}
}
```
请注意,在使用此代码之前,需要先创建一个名为“DSN”的 ODBC 数据源,并在其中创建一个名为“products”的数据表,该数据表应包含三个列:name、price 和 quantity。
阅读全文
相关推荐





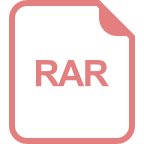

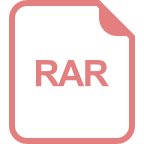
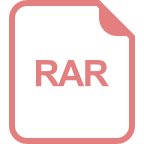


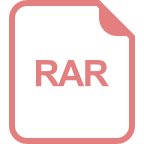

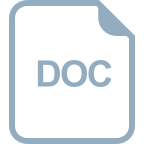
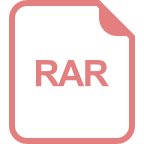



