用c语言实现凯撒密码。要求:1.由用户输入明文和位移量 2.可以进行解密操作
时间: 2024-04-30 12:23:48 浏览: 11
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100 // 最大明文长度
void encrypt(char *str, int shift); // 加密函数
void decrypt(char *str, int shift); // 解密函数
int main() {
char plain[MAX_LEN], choice;
int shift;
printf("Please enter the plaintext: ");
fgets(plain, MAX_LEN, stdin);
printf("Please enter the shift: ");
scanf("%d", &shift);
printf("Encrypt or Decrypt? (E/D): ");
while (getchar() != '\n'); // 清空输入缓冲区,避免影响后面的输入
scanf("%c", &choice);
if (choice == 'E' || choice == 'e') {
encrypt(plain, shift);
printf("Ciphertext: %s\n", plain);
} else if (choice == 'D' || choice == 'd') {
decrypt(plain, shift);
printf("Plaintext: %s\n", plain);
} else {
printf("Invalid choice.\n");
}
return 0;
}
void encrypt(char *str, int shift) {
int len = strlen(str);
for (int i = 0; i < len; ++i) {
if (str[i] >= 'a' && str[i] <= 'z') {
str[i] = 'a' + (str[i] - 'a' + shift) % 26;
} else if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] = 'A' + (str[i] - 'A' + shift) % 26;
}
}
}
void decrypt(char *str, int shift) {
encrypt(str, 26 - shift); // 解密就是把加密的位移量变成 26-位移量
}
```
代码思路:
1. 定义明文数组 `plain`,最大长度为 `MAX_LEN`。
2. 由用户输入明文和位移量。
3. 由用户选择加密或解密操作。
4. 加密操作:遍历明文数组,如果是小写字母,就将其转换成 `a + (当前字母-a+位移量)%26` 的形式;如果是大写字母,就将其转换成 `A + (当前字母-A+位移量)%26` 的形式。
5. 解密操作:由于加密的操作是对字母进行位移,所以解密的操作就是对字母进行相反的位移。具体实现是调用加密函数,传入的位移量为 `26-原来的位移量`。
6. 输出加密或解密后的密文或明文。
相关推荐
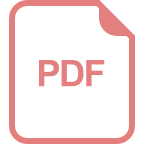
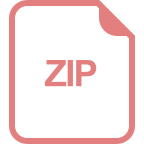
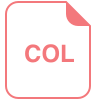
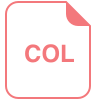
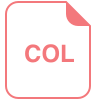
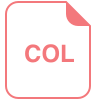
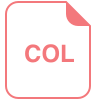









