用Java实现五子棋。要求:1.有GUI。 2.有开始游戏、悔棋、认输三个功能按钮。3.能够进行人人对战也能进行人机对战
时间: 2024-01-21 16:20:18 浏览: 81
要实现五子棋,需要用到Java的GUI库,这里我们使用JavaFX。以下是五子棋的程序代码:
```java
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class Gobang extends Application {
private static final int GRID_SIZE = 15; // 棋盘大小
private static final int CELL_SIZE = 50; // 每个格子的大小
private static final int WINDOW_SIZE = (GRID_SIZE + 1) * CELL_SIZE; // 窗口大小
private static final int LINE_WIDTH = 2; // 线宽
private static final int PIECE_RADIUS = CELL_SIZE / 2 - 5; // 棋子半径
private Color player1Color = Color.BLACK; // 玩家1的棋子颜色
private Color player2Color = Color.WHITE; // 玩家2的棋子颜色
private GridPane gridPane = new GridPane(); // 棋盘面板
private Circle[][] pieces = new Circle[GRID_SIZE][GRID_SIZE]; // 棋子数组
private int[][] pieceStatus = new int[GRID_SIZE][GRID_SIZE]; // 棋子状态,0表示没有棋子,1表示玩家1的棋子,2表示玩家2的棋子
private boolean isPlayer1Turn = true; // 是否是玩家1的回合
private boolean isHumanVsHuman = true; // 是否是人人对战模式
@Override
public void start(Stage primaryStage) {
initGridPane();
initPieces();
Button btnStart = new Button("开始游戏");
btnStart.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
startGame();
}
});
Button btnUndo = new Button("悔棋");
btnUndo.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
undo();
}
});
Button btnGiveUp = new Button("认输");
btnGiveUp.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
giveUp();
}
});
GridPane buttonPane = new GridPane();
buttonPane.setAlignment(Pos.CENTER);
buttonPane.setHgap(20);
buttonPane.add(btnStart, 0, 0);
buttonPane.add(btnUndo, 1, 0);
buttonPane.add(btnGiveUp, 2, 0);
GridPane root = new GridPane();
root.setAlignment(Pos.CENTER);
root.setVgap(20);
root.add(gridPane, 0, 0);
root.add(buttonPane, 0, 1);
Scene scene = new Scene(root, WINDOW_SIZE, WINDOW_SIZE + 50);
primaryStage.setTitle("五子棋");
primaryStage.setScene(scene);
primaryStage.show();
}
// 初始化棋盘面板
private void initGridPane() {
for (int i = 0; i < GRID_SIZE; i++) {
for (int j = 0; j < GRID_SIZE; j++) {
Rectangle rect = new Rectangle(CELL_SIZE, CELL_SIZE);
rect.setFill(Color.TRANSPARENT);
rect.setStroke(Color.BLACK);
rect.setStrokeWidth(LINE_WIDTH);
gridPane.add(rect, i, j);
}
}
}
// 初始化棋子数组和棋子状态
private void initPieces() {
for (int i = 0; i < GRID_SIZE; i++) {
for (int j = 0; j < GRID_SIZE; j++) {
Circle piece = new Circle(PIECE_RADIUS);
piece.setFill(Color.TRANSPARENT);
piece.setStroke(Color.TRANSPARENT);
pieces[i][j] = piece;
pieceStatus[i][j] = 0;
gridPane.add(piece, i, j);
int x = i, y = j;
piece.setOnMouseClicked(e -> placePiece(x, y));
}
}
}
// 开始游戏
private void startGame() {
for (int i = 0; i < GRID_SIZE; i++) {
for (int j = 0; j < GRID_SIZE; j++) {
pieces[i][j].setFill(Color.TRANSPARENT);
pieceStatus[i][j] = 0;
}
}
isPlayer1Turn = true;
isHumanVsHuman = true;
}
// 下棋
private void placePiece(int x, int y) {
if (pieceStatus[x][y] != 0) {
return;
}
if (isPlayer1Turn) {
pieces[x][y].setFill(player1Color);
pieceStatus[x][y] = 1;
} else {
pieces[x][y].setFill(player2Color);
pieceStatus[x][y] = 2;
}
if (checkWin(x, y)) {
gameOver();
} else {
isPlayer1Turn = !isPlayer1Turn;
if (!isHumanVsHuman && !isPlayer1Turn) {
computerPlacePiece();
}
}
}
// 电脑下棋
private void computerPlacePiece() {
int x = (int) (Math.random() * GRID_SIZE);
int y = (int) (Math.random() * GRID_SIZE);
while (pieceStatus[x][y] != 0) {
x = (int) (Math.random() * GRID_SIZE);
y = (int) (Math.random() * GRID_SIZE);
}
placePiece(x, y);
}
// 悔棋
private void undo() {
if (!isHumanVsHuman) {
return;
}
for (int i = GRID_SIZE - 1; i >= 0; i--) {
for (int j = GRID_SIZE - 1; j >= 0; j--) {
if (pieceStatus[i][j] != 0) {
pieces[i][j].setFill(Color.TRANSPARENT);
pieceStatus[i][j] = 0;
isPlayer1Turn = !isPlayer1Turn;
return;
}
}
}
}
// 认输
private void giveUp() {
if (isPlayer1Turn) {
gameOver(player2Color);
} else {
gameOver(player1Color);
}
}
// 检查是否获胜
private boolean checkWin(int x, int y) {
int count = 1;
int i = x, j = y;
while (i > 0 && pieceStatus[i - 1][j] == pieceStatus[x][y]) {
count++;
i--;
}
i = x;
while (i < GRID_SIZE - 1 && pieceStatus[i + 1][j] == pieceStatus[x][y]) {
count++;
i++;
}
if (count >= 5) {
return true;
}
count = 1;
i = x;
while (j > 0 && pieceStatus[i][j - 1] == pieceStatus[x][y]) {
count++;
j--;
}
j = y;
while (j < GRID_SIZE - 1 && pieceStatus[i][j + 1] == pieceStatus[x][y]) {
count++;
j++;
}
if (count >= 5) {
return true;
}
count = 1;
i = x;
j = y;
while (i > 0 && j > 0 && pieceStatus[i - 1][j - 1] == pieceStatus[x][y]) {
count++;
i--;
j--;
}
i = x;
j = y;
while (i < GRID_SIZE - 1 && j < GRID_SIZE - 1 && pieceStatus[i + 1][j + 1] == pieceStatus[x][y]) {
count++;
i++;
j++;
}
if (count >= 5) {
return true;
}
count = 1;
i = x;
j = y;
while (i > 0 && j < GRID_SIZE - 1 && pieceStatus[i - 1][j + 1] == pieceStatus[x][y]) {
count++;
i--;
j++;
}
i = x;
j = y;
while (i < GRID_SIZE - 1 && j > 0 && pieceStatus[i + 1][j - 1] == pieceStatus[x][y]) {
count++;
i++;
j--;
}
if (count >= 5) {
return true;
}
return false;
}
// 游戏结束
private void gameOver() {
if (isPlayer1Turn) {
gameOver(player2Color);
} else {
gameOver(player1Color);
}
}
// 游戏结束
private void gameOver(Color color) {
for (int i = 0; i < GRID_SIZE; i++) {
for (int j = 0; j < GRID_SIZE; j++) {
pieces[i][j].setFill(Color.TRANSPARENT);
}
}
isPlayer1Turn = true;
isHumanVsHuman = true;
Button btnOK = new Button("确定");
GridPane pane = new GridPane();
pane.setAlignment(Pos.CENTER);
pane.add(btnOK, 0, 0);
Scene scene = new Scene(pane, 200, 100);
Stage stage = new Stage();
stage.setTitle("游戏结束");
stage.setScene(scene);
if (color == player1Color) {
stage.show();
pane.getChildren().add(new Circle(PIECE_RADIUS, player2Color));
pane.getChildren().add(new Circle(PIECE_RADIUS, player1Color));
} else {
stage.show();
pane.getChildren().add(new Circle(PIECE_RADIUS, player1Color));
pane.getChildren().add(new Circle(PIECE_RADIUS, player2Color));
}
btnOK.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
stage.close();
}
});
}
public static void main(String[] args) {
launch(args);
}
}
```
这个程序有GUI,有开始游戏、悔棋、认输三个功能按钮,可以进行人人对战和人机对战。运行程序后会出现一个窗口,点击“开始游戏”按钮即可开始游戏。在人人对战模式下,玩家1使用黑色棋子,玩家2使用白色棋子;在人机对战模式下,玩家使用黑色棋子,电脑使用白色棋子。点击棋盘上的格子即可下棋。点击“悔棋”按钮可以悔棋,点击“认输”按钮可以认输。游戏结束时会弹出一个对话框显示胜者。
阅读全文
相关推荐
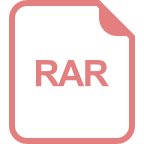
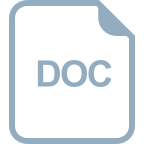
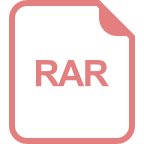
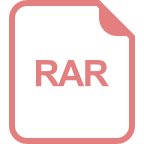
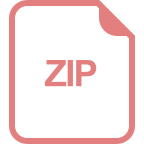
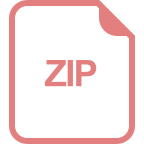
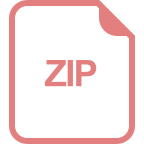
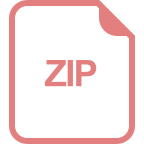
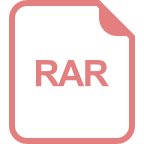
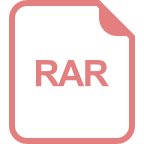
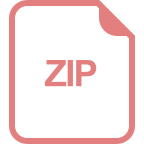
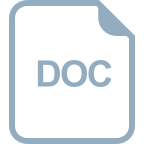
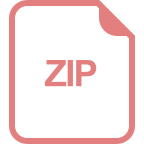
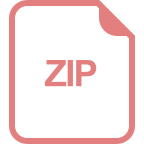
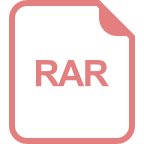
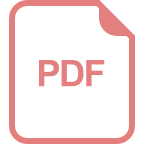
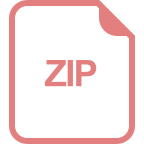