c++ hook PostMessage
时间: 2023-11-11 18:05:52 浏览: 74
The PostMessage function is used to post a message to the message queue of a thread. This function is one of the ways to communicate between different threads in Windows.
To hook the PostMessage function, you can use the Detours library provided by Microsoft. Detours allows you to intercept function calls and redirect them to your own function.
Here's an example of how to hook the PostMessage function using Detours:
```c++
#include <windows.h>
#include <detours.h>
// Define a function pointer for the original PostMessage function
typedef BOOL (WINAPI *POSTMESSAGE)(HWND, UINT, WPARAM, LPARAM);
// Define a global variable to hold the address of the original PostMessage function
POSTMESSAGE g_pOrigPostMessage = NULL;
// Define your own PostMessage function
BOOL WINAPI MyPostMessage(HWND hWnd, UINT Msg, WPARAM wParam, LPARAM lParam)
{
// Do something before calling the original PostMessage function
// ...
// Call the original PostMessage function
BOOL bRet = g_pOrigPostMessage(hWnd, Msg, wParam, lParam);
// Do something after calling the original PostMessage function
// ...
return bRet;
}
// Hook the PostMessage function
BOOL HookPostMessage()
{
// Get the address of the original PostMessage function
HMODULE hUser32 = GetModuleHandle("user32.dll");
g_pOrigPostMessage = (POSTMESSAGE)GetProcAddress(hUser32, "PostMessageA");
// Detour the PostMessage function to your own function
if (DetourTransactionBegin() == NO_ERROR)
{
if (DetourUpdateThread(GetCurrentThread()) == NO_ERROR)
{
if (DetourAttach(&(PVOID&)g_pOrigPostMessage, MyPostMessage) == NO_ERROR)
{
if (DetourTransactionCommit() == NO_ERROR)
{
return TRUE;
}
}
}
}
return FALSE;
}
// Unhook the PostMessage function
BOOL UnhookPostMessage()
{
// Detour the PostMessage function back to the original function
if (DetourTransactionBegin() == NO_ERROR)
{
if (DetourUpdateThread(GetCurrentThread()) == NO_ERROR)
{
if (DetourDetach(&(PVOID&)g_pOrigPostMessage, MyPostMessage) == NO_ERROR)
{
if (DetourTransactionCommit() == NO_ERROR)
{
return TRUE;
}
}
}
}
return FALSE;
}
// Example usage
int main()
{
// Hook the PostMessage function
if (HookPostMessage())
{
// Call the PostMessage function
PostMessage(NULL, WM_USER, 0, 0);
// Unhook the PostMessage function
UnhookPostMessage();
}
return 0;
}
```
Note that the Detours library is not officially supported by Microsoft and is intended for research and experimentation purposes only. Use it at your own risk.
相关推荐
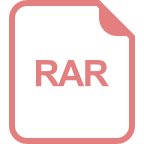














