springboot导出excel
时间: 2023-10-23 07:48:57 浏览: 45
好的,关于springboot导出excel的问题,我可以为您提供一些解决方案。您可以通过使用Apache POI框架来实现导出excel。步骤可以分为以下几个部分:1.创建Excel工作簿和表单;2.设置表头;3.添加数据;4.设置单元格样式;5.导出Excel文件。需要注意的是,您需要添加相应的依赖,如Apache POI和Apache POI OOXML。
如果您需要更加详细的代码实现,您可以参考一些相关的博客文章或者开源项目。希望这些信息可以帮助到您。
相关问题
springboot 导出excel
Spring Boot是一个快速开发框架,Spring Boot的特点是可以快速开发,容易组合,简洁,生产就绪等等。而导出Excel文件也是常用的需求,为了方便大家,Spring Boot提供了一些简单的方式来导出Excel文件。
首先,需要引入以下依赖:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.0.0</version>
</dependency>
```
接着,需要定义一个数据类,并在该类中定义对应的属性,如下:
```
@Data
public class ExcelData {
private String name;
private Integer age;
private String address;
}
```
在Controller中定义一个返回Excel文件的接口,如下:
```
@RequestMapping("/downloadExcel")
public void downloadExcel(HttpServletResponse response) throws IOException {
// 创建Excel workbook对象
XSSFWorkbook workbook = new XSSFWorkbook();
// 创建Excel sheet对象
XSSFSheet sheet = workbook.createSheet("学生信息");
// 定义表头
XSSFRow header = sheet.createRow(0);
header.createCell(0).setCellValue("姓名");
header.createCell(1).setCellValue("年龄");
header.createCell(2).setCellValue("地址");
// 添加数据
List<ExcelData> dataList = new ArrayList<>();
dataList.add(new ExcelData("张三", 20, "北京"));
dataList.add(new ExcelData("李四", 25, "上海"));
dataList.add(new ExcelData("王五", 30, "广州"));
for (int i = 0; i < dataList.size(); i++) {
XSSFRow row = sheet.createRow(i + 1);
row.createCell(0).setCellValue(dataList.get(i).getName());
row.createCell(1).setCellValue(dataList.get(i).getAge());
row.createCell(2).setCellValue(dataList.get(i).getAddress());
}
// 设置下载文件名以及响应头
response.setHeader("Content-Disposition", "attachment;filename=student.xlsx");
response.setContentType("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet");
// 输出Excel文件
workbook.write(response.getOutputStream());
}
```
最后,在浏览器中访问该接口,即可下载Excel文件student.xlsx。
上面是基于Spring Boot导出Excel表格的简单流程,我们完全可以根据需求对其中的样式和结构进行定制和完善,例如通过自定义Excel样式和数据源来获得更好的导出效果。
springboot导出Excel
要在Spring Boot中导出Excel文件,可以使用Apache POI库。以下是一些基本步骤:
1. 添加POI依赖项。在pom.xml文件中,添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建Excel文件。使用POI库创建一个Excel文件,并添加一个或多个工作表。
```java
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 添加表头
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("ID");
headerRow.createCell(1).setCellValue("Name");
headerRow.createCell(2).setCellValue("Age");
// 添加数据
Row dataRow = sheet.createRow(1);
dataRow.createCell(0).setCellValue(1);
dataRow.createCell(1).setCellValue("John");
dataRow.createCell(2).setCellValue(25);
```
3. 将Excel文件写入输出流。使用Java IO将Excel文件写入输出流,以便用户可以下载文件。
```java
response.setContentType("application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment; filename=data.xlsx");
OutputStream outputStream = response.getOutputStream();
workbook.write(outputStream);
workbook.close();
outputStream.flush();
```
完整的示例代码如下:
```java
@GetMapping("/export")
public void exportToExcel(HttpServletResponse response) throws IOException {
// 创建Excel文件
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 添加表头
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("ID");
headerRow.createCell(1).setCellValue("Name");
headerRow.createCell(2).setCellValue("Age");
// 添加数据
Row dataRow = sheet.createRow(1);
dataRow.createCell(0).setCellValue(1);
dataRow.createCell(1).setCellValue("John");
dataRow.createCell(2).setCellValue(25);
// 写入输出流
response.setContentType("application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment; filename=data.xlsx");
OutputStream outputStream = response.getOutputStream();
workbook.write(outputStream);
workbook.close();
outputStream.flush();
}
```
在浏览器中访问该URL,即可下载生成的Excel文件。
相关推荐
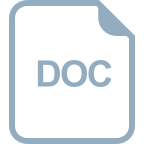
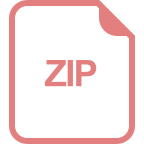
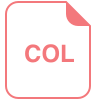
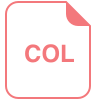
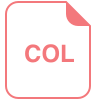
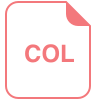
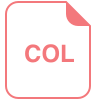







